PHP Strings & Patterns
PHP Regular Expression পর্ব-১
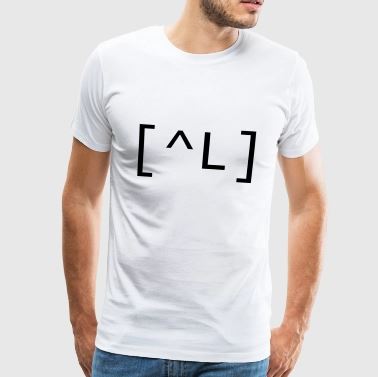
Regular Expression বা RegEx কি?
Regular Expression (যাকে আমরা সংক্ষিপ্তরূপে বলি RegEx) হচ্ছে কতগুলো সুনির্দিষ্ট ক্যারেকটারের (Character) এবং সিকোয়েন্স (Sequence) এর সমষ্টি, যা একটি Pattern তৈরী করতে ব্যবহৃত হয়। আর এই Pattern এর মাধ্যমে আমরা একটি string থেকে আমাদের প্রত্যাশিত sub string বা ক্ষুদ্র অক্ষর বিন্যাস Search অথবা replace করতে পারি। ১৯৫০ সালের দিকে মার্কিন গণিতবিদ Stephen Cole Kleene প্রথম Regular Expression এর ধারণার সূত্রপাত করেন
https://blog.w3programmers.com/php-course/
PHP Regular Expression এ Delimiter
PCRE Regular Expression এ Pattern কে যেকোনো non-alphanumeric, non-backslash, non-whitespace character দিয়ে আবদ্ধ করে দিতে হয়। যেগুলোকে বলা হয় Delimiter. সাধারণত PHP PCRE RegEx এ forward slashes (/), hash signs (#) এবং tildes (~) কে Delimeter হিসেবে ব্যবহৃত হয়। তা ছাড়া নিম্নের উদাহরণে Pattern এ দেওয়া সব গুলো Delimeter এ Valid.
1 2 3 4 | /foo bar/ #^[^0-9]$# +php+ %[a-zA-Z0-9_-]% |
বন্ধনী গুলো যেমন: (), {}, [], <> দিয়েও PHP PCRE তে Delimiter দেওয়া যায়। ঠিক নিচের মতো :
1 2 3 4 | (this [is] a (pattern)) {this [is] a (pattern)} [this [is] a (pattern)] <this [is] a (pattern)> |
PHP তে একটা বড় String এ কিভাবে কোনো কিছু খুঁজে বের করবেন?
PHP তে একটা বড় String এর মধ্যে কোনো কিছু আছে কিনা তা check করতে হলে আপনাকে ধাপ গুলো পূরণ করতে হবে।
- ১. যেকোনো একটা Delimiter দিয়ে যাকে search করতে চান তার Pattern তৈরী করুন।
- ২. preg_match() অথবা preg_match_all() ফাঙ্কশনটি call করুন। দুটি function এর ই প্রথম Parameter হবে আপনার তৈরী pattern টি , দ্বিতীয় Parameter হবে যেই String এর উপর Search চালাবেন সেটি। আর তৃতীয় Parameter টি হবে search করে পাওয়া value রাখার জন্য। সেক্ষেত্রে preg_match() এবং preg_match_all() function আপনার search করা value টি পেলে 1 রিটার্ন করবে, অন্যথায় 0 রিটার্ন করবে।
নিচের উদাহরণে সবগুলো Delimiter ব্যবহার করা হয়েছে :
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 | <?php // create a string $string = 'abcdefghijklmnopqrstuvwxyz0123456789' ; //Pattern with '/' Delimiter $pattern1 = "/pqr/" ; echo preg_match( $pattern1 , $string , $value ); //Output: 1 //Pattern with '#' Delimiter $pattern2 = "#pqr#" ; echo preg_match( $pattern2 , $string , $value ); //Output: 1 //Pattern with '+' Delimiter $pattern3 = "+pqr+" ; echo preg_match( $pattern3 , $string , $value ); //Output: 1 //Pattern with '%' Delimiter $pattern4 = "%pqr%" ; echo preg_match( $pattern4 , $string , $value ); //Output: 1 //Pattern with '~' Delimiter $pattern5 = "~pqr~" ; echo preg_match( $pattern5 , $string , $value ); //Output: 1 //Pattern with '( )' Delimiter $pattern6 = "(pqr)" ; echo preg_match( $pattern6 , $string , $value ); //Output: 1 //Pattern with '{ }' Delimiter $pattern7 = "{pqr}" ; echo preg_match( $pattern7 , $string , $value ); //Output: 1 //Pattern with '[ ]' Delimiter $pattern8 = "[pqr]" ; echo preg_match( $pattern8 , $string , $value ); //Output: 1 //Pattern with '< >' Delimiter $pattern9 = "<pqr>" ; echo preg_match( $pattern9 , $string , $value ); //Output: 1 ?> |
উল্লেখ্য , preg_match() function এর তৃতীয় Parameter এ Search করে প্রথম search value যেটি পায়, শুধু সেটি রাখে , অন্য দিকে preg_match_all() search করে পাওয়া সব গুলো value ই রাখে।
https://blog.w3programmers.com/php-course/
PHP Regular Expression এ Meta Characters
PCRE Regular Expression এ Pattern এর Delimiter এর মধ্যে কিছু Special Character ব্যবহার হয় কিছু Special কাজের জন্য, যেগুলোকে বলা হয় Meta Character. PHP PCRE RegEx এ দুই ধরণের Meta Character আছে। কিছু Meta Character আছে square brackets এর মধ্যে ব্যবহৃত হয় , আর কিছু আছে square brackets এর বাহিরে ব্যবহৃত হয়।
Meta-character | Description |
---|---|
\ | কোনো নির্দিষ্ট character কে escape করার জন্য ব্যবহৃত হয়। |
^ | String এর শুরু থেকে Search করার জন্য ব্যবহৃত হয়। |
$ | String এর শেষের দিক থেকে Search করার জন্য ব্যবহৃত হয়। |
. | যেকোনো একটি Character কে Match করার জন্য ব্যবহৃত হয়। |
[ | character class বা character group শুরু করার জন্য ব্যবহৃত হয়। |
] | character class বা character group শেষ করার জন্য ব্যবহৃত হয়। |
| | alternative বা OR Search করার জন্য ব্যবহৃত হয়। |
( | Sub Pattern শুরু করার জন্য ব্যবহৃত হয় |
) | Sub Pattern শেষ করার জন্য ব্যবহৃত হয় |
‘\’ backslash দিয়ে character কে escape করা
Example #1
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 | <?php // Sample email addresses $emailAddresses = array ( "john.doe@example.com" , "alice.smith@example.net" , "bob@example.org" ); // Regular expression pattern $pattern = '/\.com$/' ; // Validate email addresses foreach ( $emailAddresses as $email ) { if (preg_match( $pattern , $email )) { echo "Email ends with '.com': $email\n" ; } else { echo "Email does not end with '.com': $email\n" ; } } ?> |
Output
Email ends with '.com': john.doe@example.com Email does not end with '.com': alice.smith@example.net Email does not end with '.com': bob@example.org
Example #2
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 | <?php // Sample strings with a digit-hyphen-digit pattern $strings = array ( "123-456" , "789-abc" , "xyz-789" ); // Regular expression pattern $pattern = '/^\d+\-\d+$/' ; // Validate strings foreach ( $strings as $str ) { if (preg_match( $pattern , $str )) { echo "Valid pattern: $str\n" ; } else { echo "Invalid pattern: $str\n" ; } } ?> |
Output
Valid pattern: 123-456 Invalid pattern: 789-abc Invalid pattern: xyz-789
Caret (^) Sign দিয়ে String এর শুরু থেকে Search
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 | <?php // create a string $string = 'abcdefghijklmnopqrstuvwxyz0123456789' ; // try to match the beginning of the string if (preg_match( "/^abc/" , $string )) { // if it matches we echo this line echo 'The string begins with abc' ; } else { // if no match is found echo this line echo 'No match found' ; } ?> |
Output
The string begins with abc
Example #2
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 | <?php // Sample usernames $usernames = array ( "john_doe" , "alice123" , "123john" ); // Regular expression pattern $pattern = '/^[a-zA-Z]/' ; // Validate usernames foreach ( $usernames as $username ) { if (preg_match( $pattern , $username )) { echo "Valid username: $username\n" ; } else { echo "Invalid username: $username\n" ; } } ?> |
Output:
Valid username: john_doe Valid username: alice123 Invalid username: 123john
Example #3
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 | <?php // Sample strings with a prefix $strings = array ( "apple123" , "banana456" , "orange789" , "grapefruit" ); // The desired prefix $prefix = "orange" ; // Regular expression pattern $pattern = '/^' . preg_quote( $prefix , '/' ) . '/' ; // Validate strings foreach ( $strings as $str ) { if (preg_match( $pattern , $str )) { echo "String starts with '$prefix': $str\n" ; } else { echo "String does not start with '$prefix': $str\n" ; } } ?> |
Output
String does not start with 'orange': apple123 String does not start with 'orange': banana456 String starts with 'orange': orange789 String does not start with 'orange': grapefruit
dollar ($) Sign দিয়ে String এর শেষ থেকে Search
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 | <?php // create a string $string = 'abcdefghijklmnopqrstuvwxyz0123456789' ; // try to match the beginning of the string if (preg_match( "/89\$/" , $string )) { // if it matches we echo this line echo 'The string End with 89' ; } else { // if no match is found echo this line echo 'No match found' ; } ?> |
Output
The string End with 89
উল্লেখ্য, এখানে আমরা dollar ($) sign কে search value থেকে escape করার জন্য ( \ ) backword slash ব্যবহার করেছি।
Example #2
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 | <?php // Replace 'path/to/your/folder' with the actual path to your folder $folderPath = 'path/to/your/folder' ; // Desired file extension $desiredExtension = "txt" ; // Regular expression pattern $pattern = '/\.' . preg_quote( $desiredExtension , '/' ) . '$/' ; // Use scandir to get the list of files in the folder $files = scandir( $folderPath ); // Loop through the files and display the ones with the desired extension foreach ( $files as $file ) { if ( $file != "." && $file != ".." ) { if (preg_match( $pattern , $file )) { echo "File has the desired extension: $file\n" ; } else { echo "File does not have the desired extension: $file\n" ; } } } ?> |
Example #3
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 | <?php // Sample country codes $countryCodes = array ( "US" , "CA" , "GB" , "DE" , "FR" , "ABC" ); // Regular expression pattern for valid country codes $pattern = '/^[A-Z]{2}$/' ; // Validate country codes foreach ( $countryCodes as $code ) { if (preg_match( $pattern , $code )) { echo "Valid country code: $code\n" ; } else { echo "Invalid country code: $code\n" ; } } ?> |
Output
Valid country code: US Valid country code: CA Valid country code: GB Valid country code: DE Valid country code: FR Invalid country code: ABC
যেকোনো একটা character ম্যাচ করার জন্য dot বা full stop (.) sign এর ব্যবহার:
1 2 3 4 5 6 7 8 9 | <?php // create a string $string = 'The dog has no food.' ; // look for a match preg_match_all( "/d.g/" , $string , $matches ); print_r( $matches ); ?> |
Output
1 2 3 4 5 6 7 8 | Array ( [0] => Array ( [0] => dog ) ) |
Example #2
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 | <?php // Sample usernames $usernames = array ( "john.doe" , "alice_smith" , "bob123" , "user@example" ); // Regular expression pattern for valid usernames $pattern = '/^[a-zA-Z0-9._-]+$/' ; // Validate usernames foreach ( $usernames as $username ) { if (preg_match( $pattern , $username )) { echo "Valid username: $username\n" ; } else { echo "Invalid username: $username\n" ; } } ?> |
Output:
Valid username: john.doe Valid username: alice_smith Valid username: bob123 Invalid username: user@example
square brackets “[ ]” দিয়ে Character Class বা group এর মধ্যে Pattern Matching
01 02 03 04 05 06 07 08 09 10 | <?php // create a string $string = 'This is a big bog bug bag String' ; // Search for a match preg_match_all( "/b[aoiu]g/" , $string , $matches ); print_r( $matches ); ?> |
Output
01 02 03 04 05 06 07 08 09 10 11 | Array ( [0] => Array ( [0] => big [1] => bog [2] => bug [3] => bag ) ) |
Example #2
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 | <?php // Sample strings $strings = array ( "Hello123" , "Special@Chars" , "Alphanumeric567" , "Symbols!@#$" ); // Regular expression pattern for alphanumeric strings $pattern = '/^[a-zA-Z0-9]+$/' ; // Validate strings foreach ( $strings as $str ) { if (preg_match( $pattern , $str )) { echo "Alphanumeric string: $str\n" ; } else { echo "Non-alphanumeric string: $str\n" ; } } ?> |
Output
Alphanumeric string: Hello123 Non-alphanumeric string: Special@Chars Alphanumeric string: Alphanumeric567 Non-alphanumeric string: Symbols!@#$
Example #3
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 | <?php // Sample strings $strings = array ( "lowercase" , "MixedCase" , "123Numbers" , "Special@Chars" ); // Regular expression pattern for lowercase strings $pattern = '/^[a-z]+$/' ; // Validate strings foreach ( $strings as $str ) { if (preg_match( $pattern , $str )) { echo "Lowercase string: $str\n" ; } else { echo "Non-lowercase string: $str\n" ; } } ?> |
Output:
Lowercase string: lowercase Non-lowercase string: MixedCase Non-lowercase string: 123Numbers Non-lowercase string: Special@Chars
Parenthesis “( )” দিয়ে Sub Pattern তৈরী এবং Pattern Matching
01 02 03 04 05 06 07 08 09 10 | <?php // a simple string $string = "This is a Hello World script" ; // try to match the patterns Jello or Hello // put the matches in a variable called matches preg_match_all( "/(Je|He)llo/" , $string , $matches ); print_r( $matches ); ?> |
Output
01 02 03 04 05 06 07 08 09 10 11 12 13 | Array ( [0] => Array ( [0] => Hello ) [1] => Array ( [0] => He ) ) |
Example # 2
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 | <?php // Sample phone numbers $phoneNumbers = array ( "555-1234" , "(123) 456-7890" , "987-6543" , "(555) 789-0123" ); // Regular expression pattern to extract the area code $pattern = '/\((\d{3})\)/' ; // Extract and display the area code foreach ( $phoneNumbers as $phoneNumber ) { if (preg_match( $pattern , $phoneNumber , $matches )) { $areaCode = $matches [1]; echo "Phone number: $phoneNumber, Area code: $areaCode\n" ; } else { echo "No area code found for phone number: $phoneNumber\n" ; } } ?> |
Output
No area code found for phone number: 555-1234 Phone number: (123) 456-7890, Area code: 123 No area code found for phone number: 987-6543 Phone number: (555) 789-0123, Area code: 555
Example #3
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 | <?php // Sample date strings $dateStrings = array ( "2023-12-01" , "2023-06-15" , "2023-09-30" ); // Regular expression pattern to extract date components $pattern = '/^(\d{4})-(\d{2})-(\d{2})$/' ; // Extract and display date components foreach ( $dateStrings as $dateString ) { if (preg_match( $pattern , $dateString , $matches )) { $year = $matches [1]; $month = $matches [2]; $day = $matches [3]; echo "Date: $dateString, Year: $year, Month: $month, Day: $day\n" ; } else { echo "Invalid date format: $dateString\n" ; } } ?> |
Output
Date: 2023-12-01, Year: 2023, Month: 12, Day: 01 Date: 2023-06-15, Year: 2023, Month: 06, Day: 15 Date: 2023-09-30, Year: 2023, Month: 09, Day: 30
Meta-character | Description |
---|---|
\ | যেকোনো character কে escape এর জন্য ব্যবহৃত হয়। |
^ | যেকোনো character কে বাদ দিয়ে Pattern Matching এর জন্য ব্যবহৃত হয়। |
– | ক্যারেক্টার Range কে indicate করার জন্য ব্যবহৃত হয়। |
backword slash (\) দিয়ে square brackets এর মধ্যে কোনো character কে escape করা।
1 2 3 4 5 6 7 8 9 | <?php // create a string $string = 'This is a [templateVar]' ; // try to match our pattern preg_match_all( "/[\[\]]/" , $string , $matches ); print_r( $matches ); ?> |
Output
1 2 3 4 5 6 7 8 9 | Array ( [0] => Array ( [0] => [ [1] => ] ) ) |
Example #2
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 | <?php // Sample names $names = array ( "John Doe" , "Alice-Smith" , "123Name" , "Bob#Jones" ); // Regular expression pattern for names $pattern = '/^[a-zA-Z\s]+$/' ; // Validate names foreach ( $names as $name ) { if (preg_match( $pattern , $name )) { echo "Valid name: $name\n" ; } else { echo "Invalid name: $name\n" ; } } ?> |
Output:
Valid name: John Doe Invalid name: Alice-Smith Invalid name: 123Name Invalid name: Bob#Jones
Example #3
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 | <?php // Sample strings $strings = array ( "alpha-numeric" , "Special@Chars" , "12345" , "mixed123" ); // Regular expression pattern for a specific character set $pattern = '/^[a-zA-Z0-9\-]+$/' ; // Validate strings foreach ( $strings as $str ) { if (preg_match( $pattern , $str )) { echo "Valid string: $str\n" ; } else { echo "Invalid string: $str\n" ; } } ?> |
Output:
Valid string: alpha-numeric Invalid string: Special@Chars Valid string: 12345 Valid string: mixed123
Square Bracket এর মধ্যে Caret (^) Sign দিয়ে String এর নির্দিষ্ট একটি বা কতগুলো Character কে বাদ দিয়ে বাকি গুলো Search
1 2 3 4 5 6 7 8 9 | <?php // create a string $string = 'abcefghijklmnopqrstuvwxyz0123456789' ; // echo our string preg_match_all( "/[^b]/" , $string , $matches ); print_r( $matches ); ?> |
ব্যাখ্যা: এখানে b ব্যতীত বাকি গুলো print করবে।
1 2 3 4 5 6 7 8 9 | <?php // create a string $string = 'abcefghijklmnopqrstuvwxyz0123456789' ; // echo our string preg_match_all( "/[^a-z]/" , $string , $matches ); print_r( $matches ); ?> |
ব্যাখ্যা: এখানে a থেকে z character গুলো ব্যতীত বাকি গুলো print করবে। আর dash (-) sign ব্যবহার করা হয়েছে a-z range বুঝানোর জন্য।
Output
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 | Array ( [0] => Array ( [0] => 0 [1] => 1 [2] => 2 [3] => 3 [4] => 4 [5] => 5 [6] => 6 [7] => 7 [8] => 8 [9] => 9 ) ) |
Example #4
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 | <?php // Sample strings $strings = array ( "apple123" , "banana456" , "123orange" , "_grape" ); // Regular expression pattern to disallow strings starting with digits or underscores $pattern = '/^[^0-9_][a-zA-Z0-9]+$/' ; // Validate strings foreach ( $strings as $str ) { if (preg_match( $pattern , $str )) { echo "Valid string: $str\n" ; } else { echo "Invalid string: $str\n" ; } } ?> |
Output
Valid string: apple123 Valid string: banana456 Invalid string: 123orange Invalid string: _grape
PHP Regular Expression এ Special sequences
PHP Requla Expression এ কিছু Special Squence আছে যে গুলো ব্যবহার করে অনেক বড় একটা Pattern কে খুব সহজে ছোট করে ফেলা যায়। যেমন: (\w) character এর দ্বারা বুঝানো হয় যেকোনো alpha Numeric Character (a-z,A-Z,0-9,_) , নিচে সবগুলো Special sequence কে table আকারে দেওয়া হলো:
Special sequence Name | Description |
---|---|
\d | শুধু মাত্র Decimal Digit বুঝানোর জন্য। |
\D | Decimal Digit ছাড়া অন্য যেকোনো Character বুঝানোর জন্য। |
\h | যেকোনো horizontal whitespace character বুঝানোর জন্য ব্যবহৃত হয়। (since PHP 5.2.4) |
\H | horizontal whitespace character ছাড়া যেকোনো Character বুঝানোর জন্য ব্যবহৃত হয়। (since PHP 5.2.4) |
\s | যেকোনো whitespace character বুঝানোর জন্য ব্যবহৃত হয়। |
\S | whitespace character ছাড়া যেকোনো Character বুঝানোর জন্য ব্যবহৃত হয়। |
\v | যেকোনো vertical whitespace character বুঝানোর জন্য ব্যবহৃত হয়। (since PHP 5.2.4) |
\V | vertical whitespace character ছাড়া যেকোনো Character বুঝানোর জন্য ব্যবহৃত হয়(since PHP 5.2.4) |
\w | যেকোনো alpha numeric character বুঝানোর জন্য ব্যবহৃত হয়। |
\W | Non alpha numeric character বুঝানোর জন্য ব্যবহৃত হয়। |
[\d] দিয়ে শুধু মাত্র Decimal Digit গুলো Search
1 2 3 4 5 6 7 8 9 | <?php // create a string $string = 'abcefghijklmnopqrstuvwxyz0123456789' ; // echo our string preg_match_all( "/[\d]/" , $string , $matches ); print_r( $matches ); ?> |
Output
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 | Array ( [0] => Array ( [0] => 0 [1] => 1 [2] => 2 [3] => 3 [4] => 4 [5] => 5 [6] => 6 [7] => 7 [8] => 8 [9] => 9 ) ) |
Example #2
01 02 03 04 05 06 07 08 09 10 11 12 13 14 | <?php // Get the phone number from the form submission $phoneNumber = $_POST [ 'phone_number' ]; // Define the regular expression pattern $pattern = '/^[\d]+$/' ; // Perform the match if (preg_match( $pattern , $phoneNumber )) { echo "Phone number is valid: $phoneNumber" ; } else { echo "Invalid phone number. Please enter only numeric digits." ; } ?> |
এখন আমরা একটি লগ ফাইল থেকে শুধু Event Id গুলো extract করার একটি উদাহরণ দেখব। তার আগে আপনি নিম্নোক্ত টেক্সট দিয়ে logfile.txt নামে একটি ফাইল তৈরি করুন :
1 2 3 | [2023-12-24 10:30:45] Event 123: Something happened [2023-12-24 11:15:20] Event 456: Another event occurred [2023-12-24 12:00:01] Event 789: Yet another event |
এবার নিম্নোক্ত কোডগুলো লিখে পরীক্ষা করুন :
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 | <?php // Read the log file $logContent = file_get_contents ( 'logfile.txt' ); // Define the regular expression pattern $pattern = '/Event (\d+):/' ; // Perform the match if (preg_match_all( $pattern , $logContent , $matches )) { // Extracted numeric identifiers $eventIds = $matches [1]; // Print the results foreach ( $eventIds as $eventId ) { echo "Event ID: $eventId\n" ; } } else { echo "No matching events found in the log file." ; } ?> |
[\D] দিয়ে শুধু মাত্র Non Decimal Digit গুলো Search
1 2 3 4 5 6 7 8 9 | <?php // create a string $string = 'abcefghijklmnopqrstuvwxyz0123456789' ; // echo our string preg_match_all( "/[\D]/" , $string , $matches ); print_r( $matches ); ?> |
Output
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 | Array ( [0] => Array ( [0] => a [1] => b [2] => c [3] => e [4] => f [5] => g [6] => h [7] => i [8] => j [9] => k [10] => l [11] => m [12] => n [13] => o [14] => p [15] => q [16] => r [17] => s [18] => t [19] => u [20] => v [21] => w [22] => x [23] => y [24] => z ) ) |
Example #2
1 2 3 4 5 6 7 | $userInput = "JohnDoe" ; if (preg_match( '/^[\D]+$/' , $userInput )) { // Input contains only non-numeric characters echo "Valid input: $userInput" ; } else { echo "Invalid input. Please enter only letters." ; } |
Example #3
1 2 3 4 5 6 7 | $password = "P@ssw0rd" ; if (preg_match( '/[a-zA-Z]+.*[\D]+/' , $password )) { // Password meets the criteria echo "Valid password: $password" ; } else { echo "Invalid password. Ensure it contains letters and symbols." ; } |
[\s] Small s দিয়ে শুধু মাত্র white space গুলো Search
1 2 3 4 5 6 7 8 | <?php // create a string $string = 'abcdefghijklmnopqrstuvwxy 0123456789' ; // echo our string preg_match_all( "/[\s]/" , $string , $matches ); print_r( $matches ); ?> |
Output
01 02 03 04 05 06 07 08 09 10 | Array ( [0] => Array ( [0] => [1] => [2] => ) ) |
উল্লেখ্য এখানে আমাদের string এ ৩ টি whitespace আছে তাই array তিনটি null বা ফাঁকা index আসছে।
Example #2
01 02 03 04 05 06 07 08 09 10 11 12 | <?php // Sample sentence $sentence = "This is a sample sentence with multiple words." ; // Tokenize the sentence into words using \s (whitespace) as a delimiter $words = preg_split( '/\s+/' , $sentence ); // Print the result foreach ( $words as $word ) { echo "Word: $word\n" ; } ?> |
Output
Word: This Word: is Word: a Word: sample Word: sentence Word: with Word: multiple Word: words.
Example #3
এখন আমরা একটি products.txt ফাইল থেকে শুধু Product গুলো extract করার একটি উদাহরণ দেখব। তার আগে আপনি নিম্নোক্ত টেক্সট দিয়ে products.txt নামে একটি ফাইল তৈরি করুন :
1 2 3 | Laptop 799.99 10 Smartphone 499.99 20 Headphones 79.99 50 |
এবার নিম্নোক্ত কোডগুলো লিখে পরীক্ষা করুন :
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 | <?php // Read the product information from the text file $productData = file_get_contents ( 'products.txt' ); // Split each line into components using \s (whitespace) as a delimiter $lines = explode ( "\n" , $productData ); foreach ( $lines as $line ) { // Tokenize each line into components $components = preg_split( '/\s+/' , $line ); // Extracted components $productName = $components [0]; $price = $components [1]; $quantity = $components [2]; // Process the extracted data (in this example, just printing) echo "Product: $productName, Price: $price, Quantity: $quantity\n" ; } ?> |
Output
Product: Laptop, Price: 799.99, Quantity: 10 Product: Smartphone, Price: 499.99, Quantity: 20 Product: Headphones, Price: 79.99, Quantity: 50
Example #4
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 | <?php // Sample text with multiple sentences $text = "This is the first sentence. Here is the second one. And finally, the third." ; // Split the text into sentences using a period (.) as the delimiter $sentences = explode ( '.' , $text ); // Process each sentence foreach ( $sentences as $sentence ) { // Tokenize each sentence into words using \s (whitespace) as a delimiter $words = preg_split( '/\s+/' , trim( $sentence )); // Count the number of words in the sentence $wordCount = count ( $words ); // Print the result echo "Sentence: $sentence\n" ; echo "Word Count: $wordCount\n\n" ; } ?> |
Output
[\S] Capital S দিয়ে শুধু মাত্র Non white space গুলো Search
1 2 3 4 5 6 7 8 9 | <?php // create a string $string = 'abcdefghijklmnopqrstuvwxy 0123456789' ; // echo our string preg_match_all( "/[\S]/" , $string , $matches ); print_r( $matches ); ?> |
উপরের Code টির Output হিসেবে whitespace গুলো ব্যতীত বাকি সব Character ই আসবে।
Example #3
এখন আমরা একটি লগ ফাইল থেকে শুধু Date গুলো extract করার একটি উদাহরণ দেখব। তার আগে আপনি নিম্নোক্ত টেক্সট দিয়ে logfile.txt নামে একটি ফাইল তৈরি করুন :
1 2 3 | [2023-12-24 10:30:45] Event 123: Something happened [2023-12-24 11:15:20] Event 456: Another event occurred [2023-12-24 12:00:01] Event 789: Yet another event |
এবার নিম্নোক্ত কোডগুলো লিখে পরীক্ষা করুন :
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 | <?php // Read the log file $logContent = file_get_contents ( 'logfile.txt' ); // Extract timestamps using \S+ if (preg_match_all( '/\[\S+\]/' , $logContent , $matches )) { // Extracted timestamps $timestamps = $matches [0]; // Print the result foreach ( $timestamps as $timestamp ) { echo "Timestamp: $timestamp\n" ; } } else { echo "No timestamps found in the log file." ; } ?> |
Output:
Timestamp: [2023-01-01 12:30:45] Timestamp: [2023-01-02 08:15:20] Timestamp: [2023-01-03 15:00:01]
নোট: \h,\H,\v,\V গুলোও \s এবং \S এর মতোই , তবে এগুলো বিশেষ ভাবে horizontal এবং vertical white space নিয়ে কাজ করে।
[\w] দিয়ে শুধু মাত্র Alpha Numeric Character গুলো Search
1 2 3 4 5 6 7 8 9 | <?php // create a string $string = 'ab-cde*fg@ hi & jkl(mnopqr)stu+vw?x yz0>1234<567890' ; // match our pattern containing a special sequence preg_match_all( "/[\w]/" , $string , $matches ); print_r( $matches ); ?> |
Output
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 | Array ( [0] => Array ( [0] => a [1] => b [2] => c [3] => d [4] => e [5] => f [6] => g [7] => h [8] => i [9] => j [10] => k [11] => l [12] => m [13] => n [14] => o [15] => p [16] => q [17] => r [18] => s [19] => t [20] => u [21] => v [22] => w [23] => x [24] => y [25] => z [26] => 0 [27] => 1 [28] => 2 [29] => 3 [30] => 4 [31] => 5 [32] => 6 [33] => 7 [34] => 8 [35] => 9 [36] => 0 ) ) |
Example #2
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 | <?php // Sample string with email addresses $emailAddresses = "john.doe@example.com, alice_smith@example.org, bob123@example.net" ; // Extract usernames using \w if (preg_match_all( '/(\w+)@/' , $emailAddresses , $matches )) { // Extracted usernames $usernames = $matches [1]; // Print the result foreach ( $usernames as $username ) { echo "Username: $username\n" ; } } else { echo "No usernames found in the string." ; } ?> |
Output:
Username: john.doe Username: alice_smith Username: bob123
Example #3
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 | <?php // Sample string with hashtags $hashtags = "Check out this #awesome_post and use #Code_2023 for discounts! #programming #tech" ; // Extract hashtags using \w if (preg_match_all( '/#(\w+)/' , $hashtags , $matches )) { // Extracted hashtags $hashtagList = $matches [1]; // Print the result foreach ( $hashtagList as $hashtag ) { echo "Hashtag: $hashtag\n" ; } } else { echo "No hashtags found in the string." ; } ?> |
Output
Hashtag: awesome_post Hashtag: Code_2023 Hashtag: programming Hashtag: tech
[\W] দিয়ে শুধু মাত্র Non Alpha Numeric Character গুলো Search
1 2 3 4 5 6 7 8 9 | <?php // create a string $string = 'ab-cde*fg@ hi & jkl(mnopqr)stu+vw?x yz0>1234<567890' ; // match our pattern containing a special sequence preg_match_all( "/[\W]/" , $string , $matches ); print_r( $matches ); ?> |
Output
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 | Array ( [0] => Array ( [0] => - [1] => * [2] => @ [3] => [4] => [5] => & [6] => [7] => ( [8] => ) [9] => + [10] => ? [11] => [12] => > [13] => < ) ) |
3 thoughts on “PHP Regular Expression পর্ব-১”
Leave a Reply
You must be logged in to post a comment.
As-Salamu-Alaikum Sir. A very effective topic for PHP learners.
Walaikum Assalam, Thank you
যেকোনো একটা character ম্যাচ করার জন্য dot বা full stop (.) sign এর ব্যবহার:
Output
Array
(
[0] => Array
(
[0] => dog
)
)