Laravel Framework Basics
LARAVEL FRAMEWORK BASICS পর্ব-১৬: Creating a basic shopping cart system using Laravel Session
Shopping Cart এর মতো application develop করা নতুন ওয়েব ডেভেলপারদের কাছে খুবই আকর্ষণীয় একটি ব্যাপার।
আমরা এখন Laravel Session ব্যবহার করে খুবই সাধারণ, কিন্তু প্রফেশনাল একটি shopping cart তৈরি করব। আর এই shopping cart টির ফ্রন্টএন্ড ডিজাইনের জন্য আমরা tailwind css ব্যবহার করে করব। এটার product list দেখতে অনেকটা এই রকম হবে:
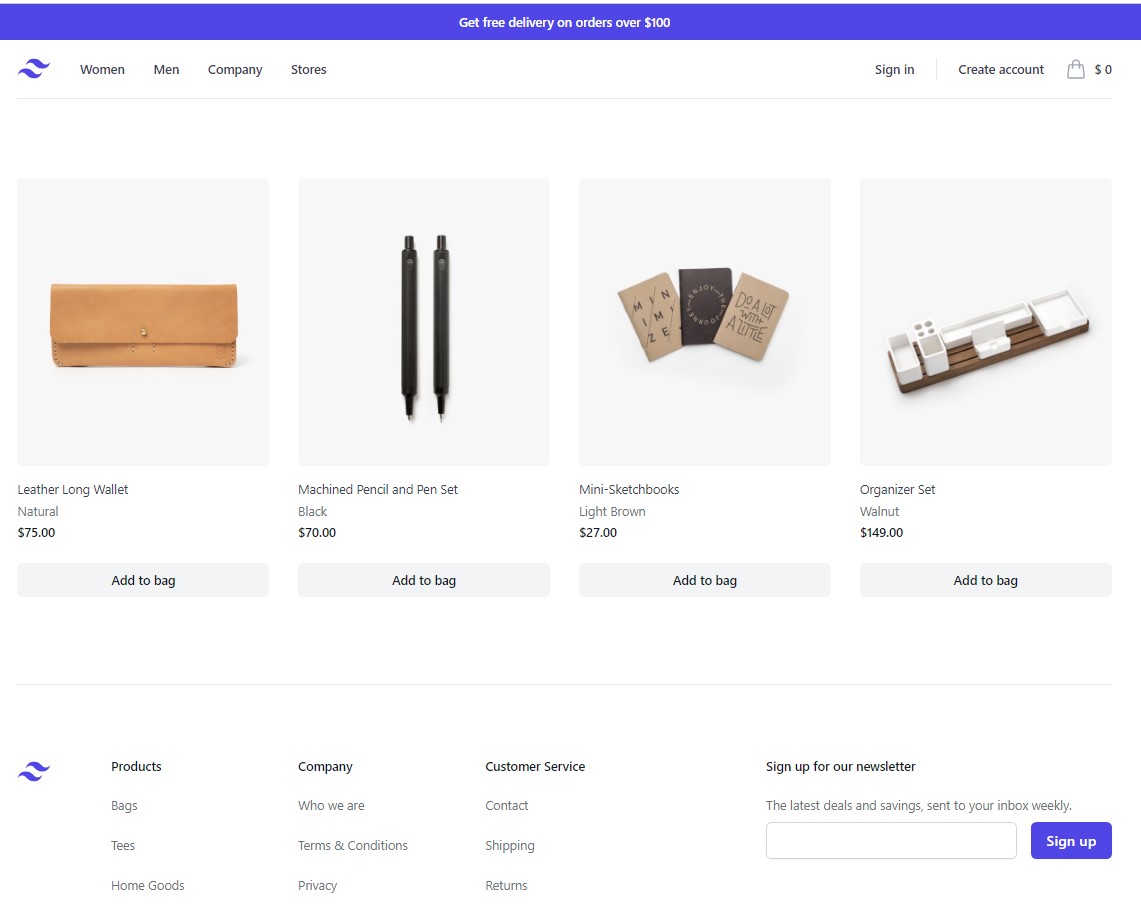
এবং এটির shopping cart দেখতে হবে নিম্নরুপঃ
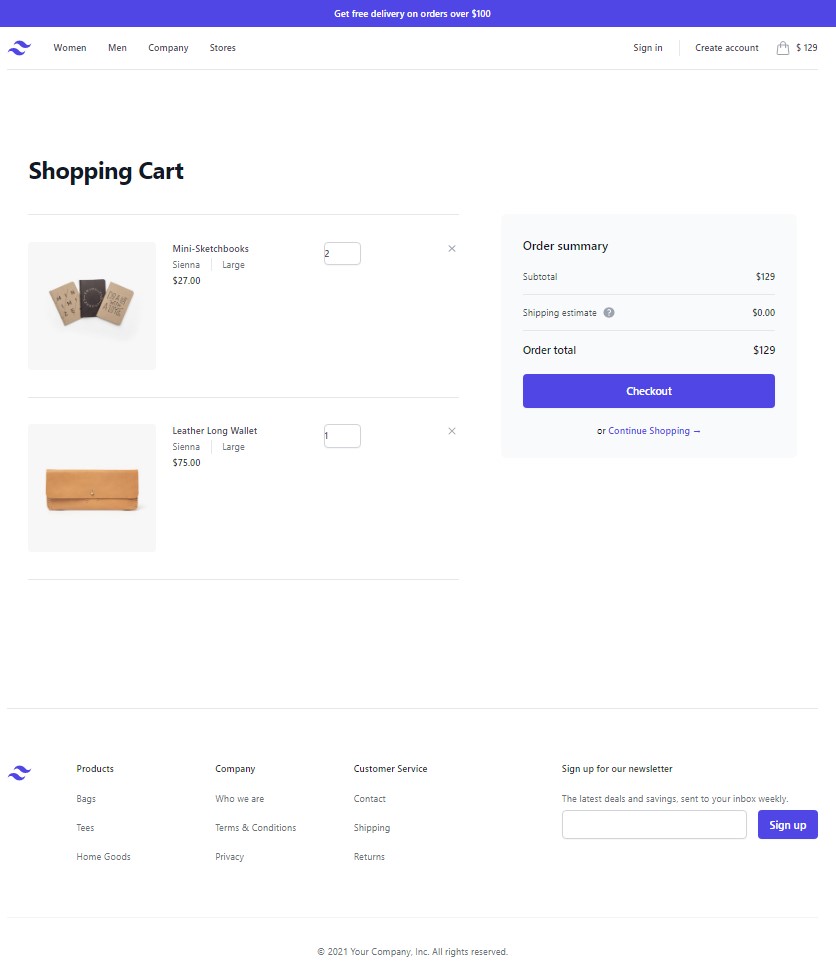
Finishing Database Related jobs first
আমরা যে shopping cart টি তৈরি করতে যাচ্ছি , তার কিছু কাজ ডেটাবেসের সাথে সম্পর্কৃত। তাই আমরা প্রথমে products নামে একটি Migration এবং Seeder ফাইল তৈরি করব।
প্রথমে ডেটাবেজে টেবিল তৈরির জন্য নিম্নোক্ত কমান্ডের মাধ্যমে একটি Migration File টি তৈরি করে ফেলুন :
1 | php artisan make:migration create_products_table |
এবার আপনার প্রজেক্টের database/migration ফোল্ডারে অবস্থিত create_products_table.php ফাইলে নিচের মতো করে আপডেট করে নিন।
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 | <?php use Illuminate\Database\Migrations\Migration; use Illuminate\Database\Schema\Blueprint; use Illuminate\Support\Facades\Schema; return new class extends Migration { /** * Run the migrations. * * @return void */ public function up() { Schema::create( 'products' , function (Blueprint $table ) { $table ->id(); $table ->string( "name" , 255)->nullable(); $table ->text( "description" )->nullable(); $table ->string( "image" , 255)->nullable(); $table ->decimal( "price" , 6, 2); $table ->timestamps(); }); } /** * Reverse the migrations. * * @return void */ public function down() { Schema::dropIfExists( 'products' ); } }; |
এবার আমরা নিচের আর্টিসান কমান্ডটি ব্যবহার করে Product নামে একটি Model তৈরি করব :
1 | php artisan make:model Product |
Migration File এর মতো একইভাবে আমাদের Model ফাইলটিকে নিচের মতো করে আপডেট করে নিব :
01 02 03 04 05 06 07 08 09 10 11 12 13 14 | <?php namespace App\Models; use Illuminate\Database\Eloquent\Factories\HasFactory; use Illuminate\Database\Eloquent\Model; class Product extends Model { use HasFactory; protected $fillable = [ 'name' , 'price' , 'description' , 'image' ]; } |
এবার নিম্নোক্ত কমান্ডের মাধ্যমে ডেটাবেজ টি আপডেট করে নিন , অর্থাৎ নতুন Product Table এর স্ট্রাকচার তৈরি করে নিন।
1 | php artisan migrate:refresh |
এখন আমরা ডেটাবেজে কিছু Dummy Product যুক্ত করার কাজ করব। আর এর জন্য আমরা Laravel Seeder এর সাহায্য নিবো। প্রথমে নিচের কমান্ড ব্যবহার করে একটি Seeder File তৈরি করে ফেলুন :
1 | php artisan make:seeder ProductSeeder |
এবার আপনার প্রজেক্টের database/seeders ফোল্ডারে অবস্থিত ProductSeeder.php ফাইলে নিচের মতো করে আপডেট করে নিন।
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 | <?php namespace Database\Seeders; use Illuminate\Database\Console\Seeds\WithoutModelEvents; use Illuminate\Database\Seeder; use App\Models\Product; class ProductSeeder extends Seeder { /** * Run the database seeds. * * @return void */ public function run() { $products =[ [ 'name' => 'Basic Tee' , 'description' => 'Sienn | Large' , 'image' => 'https://tailwindui.com/img/ecommerce-images/shopping-cart-page-01-product-01.jpg' , 'price' =>100 ], [ 'name' => 'Nomad Tumbler' , 'description' => 'White' , 'image' => 'https://tailwindui.com/img/ecommerce-images/shopping-cart-page-01-product-03.jpg' , 'price' =>35 ], [ 'name' => 'Leatherbound Daily Journal Set' , 'description' => 'Test Natural' , 'image' => 'https://tailwindui.com/img/ecommerce-images/home-page-02-product-03.jpg' , 'price' =>67 ], [ 'name' => 'Billfold Wallet' , 'description' => 'Natural' , 'image' => 'https://tailwindui.com/img/ecommerce-images/home-page-04-trending-product-02.jpg' , 'price' =>75 ] ]; foreach ( $products as $key => $value ){ Product::create( $value ); } } } |
এবার আপনার প্রজেক্টের database/seeders ফোল্ডারে অবস্থিত DatabaseSeeder.php ফাইলে নিচের মতো করে আপডেট করে নিন।
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 | <?php namespace Database\Seeders; use Illuminate\Database\Console\Seeds\WithoutModelEvents; use Illuminate\Database\Seeder; class DatabaseSeeder extends Seeder { /** * Seed the application's database. * * @return void */ public function run() { $this ->call([ ProductSeeder:: class , ]); } } |
এবার নিম্নোক্ত কমান্ডের মাধ্যমে ডেটাবেজ টি আপডেট করে নিন , অর্থাৎ Dummy Product গুলো Insert করে নিন।
1 | php artisan db:seed |
Creating Controller
এখন আমরা আমাদের Product এবং তার বিস্তারিত Database থেকে নিয়ে আসার জন্য এবং Session এ Product গুলো add এবং remove করার জন্য নিম্নোক্ত ProductController তৈরি করব :
1 | php artisan make:controller ProductController |
এবার app\Http\Controllers ফোল্ডারের মধ্যে সদ্য তৈরি হওয়া ProductController.php ফাইলে নিম্নোক্ত কোডগুলো দিয়ে আপডেট করে নিন :
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 | <?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\Product; class ProductController extends Controller { public function index() { $products = Product::all(); return view( 'pages.productlist' , compact( 'products' )); } public function cart() { return view( 'pages.cart' ); } public function addToCart( $id ) { $product = Product::findOrFail( $id ); $cart = session()->get( 'cart' , []); if (isset( $cart [ $id ])) { $cart [ $id ][ 'quantity' ]++; } else { $cart [ $id ] = [ "name" => $product ->name, "quantity" => 1, "price" => $product ->price, "image" => $product ->image ]; } session()->put( 'cart' , $cart ); return redirect()->back()->with( 'success' , 'Product added to cart successfully!' ); } public function update(Request $request ) { if ( $request ->id && $request ->quantity){ $cart = session()->get( 'cart' ); $cart [ $request ->id][ "quantity" ] = $request ->quantity; session()->put( 'cart' , $cart ); session()->flash( 'success' , 'Cart updated successfully' ); } } public function remove(Request $request ) { if ( $request ->id) { $cart = session()->get( 'cart' ); if (isset( $cart [ $request ->id])) { unset( $cart [ $request ->id]); session()->put( 'cart' , $cart ); } session()->flash( 'success' , 'Product removed successfully' ); } } } |
ব্যাস হয়ে গেল আমাদের Database এবং Controller এর কাজ। এখন আমরা প্রথমে Blade Templating এর কাজটি সেরে ফেলব। আর এই প্রজেক্ট আমরা Blade Directive এর পরিবর্তে Blade Component কে ব্যবহার করব।
Conversion of HTML Template to Laravel Blade Template
প্রথমে আমাদের Product HTML Template এর কোড গুলো দেখে নিনঃ
001 002 003 004 005 006 007 008 009 010 011 012 013 014 015 016 017 018 019 020 021 022 023 024 025 026 027 028 029 030 031 032 033 034 035 036 037 038 039 040 041 042 043 044 045 046 047 048 049 050 051 052 053 054 055 056 057 058 059 060 061 062 063 064 065 066 067 068 069 070 071 072 073 074 075 076 077 078 079 080 081 082 083 084 085 086 087 088 089 090 091 092 093 094 095 096 097 098 099 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 | <!doctype html> <html> <head> <meta charset= "UTF-8" > <meta name= "viewport" content= "width=device-width, initial-scale=1.0" > <script src= "https://cdn.tailwindcss.com" ></script> <script src= "https://unpkg.com/flowbite@1.5.3/dist/flowbite.js" ></script> <script src= "https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js" ></script> <title>Products List</title> </head> <body> <div class = "bg-white" > <p class = "flex h-10 items-center justify-center bg-indigo-600 px-4 text-sm font-medium text-white sm:px-6 lg:px-8" >Get free delivery on orders over $100 </p> <nav aria-label= "Top" class = "mx-auto max-w-7xl px-4 sm:px-6 lg:px-8" > <div class = "border-b border-gray-200" > <div class = "flex h-16 items-center" > <button type= "button" x-description= "Mobile menu toggle, controls the 'mobileMenuOpen' state." class = "rounded-md bg-white p-2 text-gray-400 lg:hidden" @click= "open = true" > <span class = "sr-only" >Open menu</span> <svg class = "h-6 w-6" x-description= "Heroicon name: outline/bars-3" xmlns= "http://www.w3.org/2000/svg" fill= "none" viewBox= "0 0 24 24" stroke-width= "1.5" stroke= "currentColor" aria-hidden= "true" > <path stroke-linecap= "round" stroke-linejoin= "round" d= "M3.75 6.75h16.5M3.75 12h16.5m-16.5 5.25h16.5" ></path> </svg> </button> <!-- Logo --> <div class = "ml-4 flex lg:ml-0" > <a href= "http://localhost:8000/shop" > <img class = "h-8 w-auto" src= "https://tailwindui.com/img/logos/workflow-mark.svg?color=indigo&shade=600" alt= "" > </a> </div> <!-- Flyout menus --> <div class = "hidden lg:ml-8 lg:block lg:self-stretch" > <div class = "flex h-full space-x-8" > <a href= "#" class = "flex items-center text-sm font-medium text-gray-700 hover:text-gray-800" >Women</a> <a href= "#" class = "flex items-center text-sm font-medium text-gray-700 hover:text-gray-800" >Men</a> <a href= "#" class = "flex items-center text-sm font-medium text-gray-700 hover:text-gray-800" >Company</a> <a href= "#" class = "flex items-center text-sm font-medium text-gray-700 hover:text-gray-800" >Stores</a> </div> </div> <div class = "ml-auto flex items-center" > <div class = "hidden lg:flex lg:flex-1 lg:items-center lg:justify-end lg:space-x-6" > <a href= "#" class = "text-sm font-medium text-gray-700 hover:text-gray-800" >Sign in</a> <span class = "h-6 w-px bg-gray-200" aria-hidden= "true" ></span> <a href= "#" class = "text-sm font-medium text-gray-700 hover:text-gray-800" >Create account</a> </div> <!-- Cart --> <div class = "ml-4 flow-root lg:ml-6" > <a href= "http://localhost:8000/shop/cart" class = "group -m-2 flex items-center p-2" > <svg class = "h-6 w-6 flex-shrink-0 text-gray-400 group-hover:text-gray-500" x-description= "Heroicon name: outline/shopping-bag" xmlns= "http://www.w3.org/2000/svg" fill= "none" viewBox= "0 0 24 24" stroke-width= "1.5" stroke= "currentColor" aria-hidden= "true" > <path stroke-linecap= "round" stroke-linejoin= "round" d= "M15.75 10.5V6a3.75 3.75 0 10-7.5 0v4.5m11.356-1.993l1.263 12c.07.665-.45 1.243-1.119 1.243H4.25a1.125 1.125 0 01-1.12-1.243l1.264-12A1.125 1.125 0 015.513 7.5h12.974c.576 0 1.059.435 1.119 1.007zM8.625 10.5a.375.375 0 11-.75 0 .375.375 0 01.75 0zm7.5 0a.375.375 0 11-.75 0 .375.375 0 01.75 0z" ></path> </svg> <span class = "ml-2 text-sm font-medium text-gray-700 group-hover:text-gray-800" >$ 129</span> <span class = "sr-only" >items in cart, view bag</span> </a> </div> </div> </div> </div> </nav> <main class = "mx-auto max-w-2xl px-4 pt-16 pb-24 sm:px-6 lg:max-w-7xl lg:px-8" > <div class = "mx-auto max-w-7xl mt-6 grid grid-cols-2 gap-x-4 gap-y-10 sm:gap-x-6 md:grid-cols-4 md:gap-y-0 lg:gap-x-8" > <div class = "group relative" > <div class = "h-56 w-full overflow-hidden rounded-md bg-gray-200 group-hover:opacity-75 lg:h-72 xl:h-80" > <img src= "https://tailwindui.com/img/ecommerce-images/home-page-04-trending-product-02.jpg" alt= "Leather Long Wallet" class = "h-full w-full object-cover object-center" > </div> <h3 class = "mt-4 text-sm text-gray-700" > <a href= "#" > <span class = "absolute inset-0" ></span> Leather Long Wallet </a> </h3> <p class = "mt-1 text-sm text-gray-500" >Natural</p> <p class = "mt-1 text-sm font-medium text-gray-900" > $75 .00</p> <div class = "mt-6" > <a href= "http://localhost:8000/shop/add-to-cart/1" class = "relative flex items-center justify-center rounded-md border border-transparent bg-gray-100 py-2 px-8 text-sm font-medium text-gray-900 hover:bg-gray-200" >Add to bag<span class = "sr-only" >, Halfsize Tote</span></a> </div> </div> <div class = "group relative" > <div class = "h-56 w-full overflow-hidden rounded-md bg-gray-200 group-hover:opacity-75 lg:h-72 xl:h-80" > <img src= "https://tailwindui.com/img/ecommerce-images/home-page-04-trending-product-03.jpg" alt= " Machined Pencil and Pen Set" class = "h-full w-full object-cover object-center" > </div> <h3 class = "mt-4 text-sm text-gray-700" > <a href= "#" > <span class = "absolute inset-0" ></span> Machined Pencil and Pen Set </a> </h3> <p class = "mt-1 text-sm text-gray-500" >Black</p> <p class = "mt-1 text-sm font-medium text-gray-900" > $70 .00</p> <div class = "mt-6" > <a href= "http://localhost:8000/shop/add-to-cart/2" class = "relative flex items-center justify-center rounded-md border border-transparent bg-gray-100 py-2 px-8 text-sm font-medium text-gray-900 hover:bg-gray-200" >Add to bag<span class = "sr-only" >, Halfsize Tote</span></a> </div> </div> <div class = "group relative" > <div class = "h-56 w-full overflow-hidden rounded-md bg-gray-200 group-hover:opacity-75 lg:h-72 xl:h-80" > <img src= "https://tailwindui.com/img/ecommerce-images/home-page-04-trending-product-04.jpg" alt= "Mini-Sketchbooks" class = "h-full w-full object-cover object-center" > </div> <h3 class = "mt-4 text-sm text-gray-700" > <a href= "#" > <span class = "absolute inset-0" ></span> Mini-Sketchbooks </a> </h3> <p class = "mt-1 text-sm text-gray-500" >Light Brown</p> <p class = "mt-1 text-sm font-medium text-gray-900" > $27 .00</p> <div class = "mt-6" > <a href= "http://localhost:8000/shop/add-to-cart/3" class = "relative flex items-center justify-center rounded-md border border-transparent bg-gray-100 py-2 px-8 text-sm font-medium text-gray-900 hover:bg-gray-200" >Add to bag<span class = "sr-only" >, Halfsize Tote</span></a> </div> </div> <div class = "group relative" > <div class = "h-56 w-full overflow-hidden rounded-md bg-gray-200 group-hover:opacity-75 lg:h-72 xl:h-80" > <img src= "https://tailwindui.com/img/ecommerce-images/home-page-04-trending-product-01.jpg" alt= "Organizer Set" class = "h-full w-full object-cover object-center" > </div> <h3 class = "mt-4 text-sm text-gray-700" > <a href= "#" > <span class = "absolute inset-0" ></span> Organizer Set </a> </h3> <p class = "mt-1 text-sm text-gray-500" >Walnut</p> <p class = "mt-1 text-sm font-medium text-gray-900" > $149 .00</p> <div class = "mt-6" > <a href= "http://localhost:8000/shop/add-to-cart/4" class = "relative flex items-center justify-center rounded-md border border-transparent bg-gray-100 py-2 px-8 text-sm font-medium text-gray-900 hover:bg-gray-200" >Add to bag<span class = "sr-only" >, Halfsize Tote</span></a> </div> </div> </div> </main> <footer aria-labelledby= "footer-heading" class = "bg-white" > <h2 id= "footer-heading" class = "sr-only" >Footer</h2> <div class = "mx-auto max-w-7xl px-4 sm:px-6 lg:px-8" > <div class = "border-t border-gray-200 py-20" > <div class = "grid grid-cols-1 md:grid-flow-col md:auto-rows-min md:grid-cols-12 md:gap-x-8 md:gap-y-16" > <!-- Image section --> <div class = "col-span-1 md:col-span-2 lg:col-start-1 lg:row-start-1" > <img src= "https://tailwindui.com/img/logos/mark.svg?color=indigo&shade=600" alt= "" class = "h-8 w-auto" > </div> <!-- Sitemap sections --> <div class = "col-span-6 mt-10 grid grid-cols-2 gap-8 sm:grid-cols-3 md:col-span-8 md:col-start-3 md:row-start-1 md:mt-0 lg:col-span-6 lg:col-start-2" > <div class = "grid grid-cols-1 gap-y-12 sm:col-span-2 sm:grid-cols-2 sm:gap-x-8" > <div> <h3 class = "text-sm font-medium text-gray-900" >Products</h3> <ul role= "list" class = "mt-6 space-y-6" > <li class = "text-sm" > <a href= "#" class = "text-gray-500 hover:text-gray-600" >Bags</a> </li> <li class = "text-sm" > <a href= "#" class = "text-gray-500 hover:text-gray-600" >Tees</a> </li> <li class = "text-sm" > <a href= "#" class = "text-gray-500 hover:text-gray-600" >Home Goods</a> </li> </ul> </div> <div> <h3 class = "text-sm font-medium text-gray-900" >Company</h3> <ul role= "list" class = "mt-6 space-y-6" > <li class = "text-sm" > <a href= "#" class = "text-gray-500 hover:text-gray-600" >Who we are</a> </li> <li class = "text-sm" > <a href= "#" class = "text-gray-500 hover:text-gray-600" >Terms & Conditions</a> </li> <li class = "text-sm" > <a href= "#" class = "text-gray-500 hover:text-gray-600" >Privacy</a> </li> </ul> </div> </div> <div> <h3 class = "text-sm font-medium text-gray-900" >Customer Service</h3> <ul role= "list" class = "mt-6 space-y-6" > <li class = "text-sm" > <a href= "#" class = "text-gray-500 hover:text-gray-600" >Contact</a> </li> <li class = "text-sm" > <a href= "#" class = "text-gray-500 hover:text-gray-600" >Shipping</a> </li> <li class = "text-sm" > <a href= "#" class = "text-gray-500 hover:text-gray-600" >Returns</a> </li> </ul> </div> </div> <!-- Newsletter section --> <div class = "mt-12 md:col-span-8 md:col-start-3 md:row-start-2 md:mt-0 lg:col-span-4 lg:col-start-9 lg:row-start-1" > <h3 class = "text-sm font-medium text-gray-900" >Sign up for our newsletter</h3> <p class = "mt-6 text-sm text-gray-500" >The latest deals and savings, sent to your inbox weekly.</p> <form class = "mt-2 flex sm:max-w-md" > <label for = "email-address" class = "sr-only" >Email address</label> <input id= "email-address" type= "text" autocomplete= "email" required= "" class = "w-full min-w-0 appearance-none rounded-md border border-gray-300 bg-white py-2 px-4 text-base text-gray-900 placeholder-gray-500 shadow-sm focus:border-indigo-500 focus:outline-none focus:ring-1 focus:ring-indigo-500" > <div class = "ml-4 flex-shrink-0" > <button type= "submit" class = "flex w-full items-center justify-center rounded-md border border-transparent bg-indigo-600 py-2 px-4 text-base font-medium text-white shadow-sm hover:bg-indigo-700 focus:outline-none focus:ring-2 focus:ring-indigo-500 focus:ring-offset-2" >Sign up</button> </div> </form> </div> </div> </div> <div class = "border-t border-gray-100 py-10 text-center" > <p class = "text-sm text-gray-500" >© 2021 Your Company, Inc. All rights reserved.</p> </div> </div> </footer> </div> </body> </html> |
আর আমাদের Shopping Cart HTML Template এর কোড গুলো দেখে নিনঃ
001 002 003 004 005 006 007 008 009 010 011 012 013 014 015 016 017 018 019 020 021 022 023 024 025 026 027 028 029 030 031 032 033 034 035 036 037 038 039 040 041 042 043 044 045 046 047 048 049 050 051 052 053 054 055 056 057 058 059 060 061 062 063 064 065 066 067 068 069 070 071 072 073 074 075 076 077 078 079 080 081 082 083 084 085 086 087 088 089 090 091 092 093 094 095 096 097 098 099 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 292 293 294 295 296 297 298 299 300 301 302 303 | <!doctype html> <html> <head> <meta charset= "UTF-8" > <meta name= "viewport" content= "width=device-width, initial-scale=1.0" > <script src= "https://cdn.tailwindcss.com" ></script> <script src= "https://unpkg.com/flowbite@1.5.3/dist/flowbite.js" ></script> <script src= "https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js" ></script> <title>Shopping Cart</title> </head> <body> <div class = "bg-white" > <p class = "flex h-10 items-center justify-center bg-indigo-600 px-4 text-sm font-medium text-white sm:px-6 lg:px-8" >Get free delivery on orders over $100 </p> <nav aria-label= "Top" class = "mx-auto max-w-7xl px-4 sm:px-6 lg:px-8" > <div class = "border-b border-gray-200" > <div class = "flex h-16 items-center" > <button type= "button" x-description= "Mobile menu toggle, controls the 'mobileMenuOpen' state." class = "rounded-md bg-white p-2 text-gray-400 lg:hidden" @click= "open = true" > <span class = "sr-only" >Open menu</span> <svg class = "h-6 w-6" x-description= "Heroicon name: outline/bars-3" xmlns= "http://www.w3.org/2000/svg" fill= "none" viewBox= "0 0 24 24" stroke-width= "1.5" stroke= "currentColor" aria-hidden= "true" > <path stroke-linecap= "round" stroke-linejoin= "round" d= "M3.75 6.75h16.5M3.75 12h16.5m-16.5 5.25h16.5" ></path> </svg> </button> <!-- Logo --> <div class = "ml-4 flex lg:ml-0" > <a href= "http://localhost:8000/shop" > <img class = "h-8 w-auto" src= "https://tailwindui.com/img/logos/workflow-mark.svg?color=indigo&shade=600" alt= "" > </a> </div> <!-- Flyout menus --> <div class = "hidden lg:ml-8 lg:block lg:self-stretch" > <div class = "flex h-full space-x-8" > <a href= "#" class = "flex items-center text-sm font-medium text-gray-700 hover:text-gray-800" >Women</a> <a href= "#" class = "flex items-center text-sm font-medium text-gray-700 hover:text-gray-800" >Men</a> <a href= "#" class = "flex items-center text-sm font-medium text-gray-700 hover:text-gray-800" >Company</a> <a href= "#" class = "flex items-center text-sm font-medium text-gray-700 hover:text-gray-800" >Stores</a> </div> </div> <div class = "ml-auto flex items-center" > <div class = "hidden lg:flex lg:flex-1 lg:items-center lg:justify-end lg:space-x-6" > <a href= "#" class = "text-sm font-medium text-gray-700 hover:text-gray-800" >Sign in</a> <span class = "h-6 w-px bg-gray-200" aria-hidden= "true" ></span> <a href= "#" class = "text-sm font-medium text-gray-700 hover:text-gray-800" >Create account</a> </div> <!-- Cart --> <div class = "ml-4 flow-root lg:ml-6" > <a href= "http://localhost:8000/shop/cart" class = "group -m-2 flex items-center p-2" > <svg class = "h-6 w-6 flex-shrink-0 text-gray-400 group-hover:text-gray-500" x-description= "Heroicon name: outline/shopping-bag" xmlns= "http://www.w3.org/2000/svg" fill= "none" viewBox= "0 0 24 24" stroke-width= "1.5" stroke= "currentColor" aria-hidden= "true" > <path stroke-linecap= "round" stroke-linejoin= "round" d= "M15.75 10.5V6a3.75 3.75 0 10-7.5 0v4.5m11.356-1.993l1.263 12c.07.665-.45 1.243-1.119 1.243H4.25a1.125 1.125 0 01-1.12-1.243l1.264-12A1.125 1.125 0 015.513 7.5h12.974c.576 0 1.059.435 1.119 1.007zM8.625 10.5a.375.375 0 11-.75 0 .375.375 0 01.75 0zm7.5 0a.375.375 0 11-.75 0 .375.375 0 01.75 0z" ></path> </svg> <span class = "ml-2 text-sm font-medium text-gray-700 group-hover:text-gray-800" >$ 129</span> <span class = "sr-only" >items in cart, view bag</span> </a> </div> </div> </div> </div> </nav> <main class = "mx-auto max-w-2xl px-4 pt-16 pb-24 sm:px-6 lg:max-w-7xl lg:px-8" > <div class = "bg-white" > <div class = "mx-auto max-w-2xl px-4 pt-16 pb-24 sm:px-6 lg:max-w-7xl lg:px-8" > <h2 class = "text-3xl font-bold tracking-tight text-gray-900 sm:text-4xl" >Shopping Cart</h2> <form class = "mt-12 lg:grid lg:grid-cols-12 lg:items-start lg:gap-x-12 xl:gap-x-16" > <section aria-labelledby= "cart-heading" class = "lg:col-span-7" > <h2 id= "cart-heading" class = "sr-only" >Items in your shopping cart</h2> <ul id= "cart" role= "list" class = "divide-y divide-gray-200 border-t border-b border-gray-200" > <li class = "flex py-6 sm:py-10" data-id= "3" > <div class = "flex-shrink-0" > <img src= "https://tailwindui.com/img/ecommerce-images/home-page-04-trending-product-04.jpg" alt= "Front of men's Basic Tee in sienna." class = "h-24 w-24 rounded-md object-cover object-center sm:h-48 sm:w-48" > </div> <div class = "ml-4 flex flex-1 flex-col justify-between sm:ml-6" > <div class = "relative pr-9 sm:grid sm:grid-cols-2 sm:gap-x-6 sm:pr-0" > <div> <div class = "flex justify-between" > <h3 class = "text-sm" > <a href= "#" class = "font-medium text-gray-700 hover:text-gray-800" >Mini-Sketchbooks</a> </h3> </div> <div class = "mt-1 flex text-sm" > <p class = "text-gray-500" >Sienna</p> <p class = "ml-4 border-l border-gray-200 pl-4 text-gray-500" >Large</p> </div> <p class = "mt-1 text-sm font-medium text-gray-900" > $27 .00</p> </div> <div class = "mt-4 sm:mt-0 sm:pr-9" > <label for = "quantity-0" class = "sr-only" >Quantity, Basic Tee</label> <input type= "number" value= "2" class = "quantity update-cart w-1/3 rounded-md border border-gray-300 py-1.5 text-left text-base font-medium leading-5 text-gray-700 shadow-sm focus:border-indigo-500 focus:outline-none focus:ring-1 focus:ring-indigo-500 sm:text-sm" /> <div class = "actions absolute top-0 right-0" > <button type= "button" class = "remove-from-cart -m-2 inline-flex p-2 text-gray-400 hover:text-gray-500" > <span class = "sr-only " >Remove</span> <!-- Heroicon name: mini/x-mark --> <svg class = "h-5 w-5" xmlns= "http://www.w3.org/2000/svg" viewBox= "0 0 20 20" fill= "currentColor" aria-hidden= "true" > <path d= "M6.28 5.22a.75.75 0 00-1.06 1.06L8.94 10l-3.72 3.72a.75.75 0 101.06 1.06L10 11.06l3.72 3.72a.75.75 0 101.06-1.06L11.06 10l3.72-3.72a.75.75 0 00-1.06-1.06L10 8.94 6.28 5.22z" /> </svg> </button> </div> </div> </div> </div> </li> <li class = "flex py-6 sm:py-10" data-id= "1" > <div class = "flex-shrink-0" > <img src= "https://tailwindui.com/img/ecommerce-images/home-page-04-trending-product-02.jpg" alt= "Front of men's Basic Tee in sienna." class = "h-24 w-24 rounded-md object-cover object-center sm:h-48 sm:w-48" > </div> <div class = "ml-4 flex flex-1 flex-col justify-between sm:ml-6" > <div class = "relative pr-9 sm:grid sm:grid-cols-2 sm:gap-x-6 sm:pr-0" > <div> <div class = "flex justify-between" > <h3 class = "text-sm" > <a href= "#" class = "font-medium text-gray-700 hover:text-gray-800" >Leather Long Wallet</a> </h3> </div> <div class = "mt-1 flex text-sm" > <p class = "text-gray-500" >Sienna</p> <p class = "ml-4 border-l border-gray-200 pl-4 text-gray-500" >Large</p> </div> <p class = "mt-1 text-sm font-medium text-gray-900" > $75 .00</p> </div> <div class = "mt-4 sm:mt-0 sm:pr-9" > <label for = "quantity-0" class = "sr-only" >Quantity, Basic Tee</label> <input type= "number" value= "1" class = "quantity update-cart w-1/3 rounded-md border border-gray-300 py-1.5 text-left text-base font-medium leading-5 text-gray-700 shadow-sm focus:border-indigo-500 focus:outline-none focus:ring-1 focus:ring-indigo-500 sm:text-sm" /> <div class = "actions absolute top-0 right-0" > <button type= "button" class = "remove-from-cart -m-2 inline-flex p-2 text-gray-400 hover:text-gray-500" > <span class = "sr-only " >Remove</span> <!-- Heroicon name: mini/x-mark --> <svg class = "h-5 w-5" xmlns= "http://www.w3.org/2000/svg" viewBox= "0 0 20 20" fill= "currentColor" aria-hidden= "true" > <path d= "M6.28 5.22a.75.75 0 00-1.06 1.06L8.94 10l-3.72 3.72a.75.75 0 101.06 1.06L10 11.06l3.72 3.72a.75.75 0 101.06-1.06L11.06 10l3.72-3.72a.75.75 0 00-1.06-1.06L10 8.94 6.28 5.22z" /> </svg> </button> </div> </div> </div> </div> </li> </ul> </section> <!-- Order summary --> <section aria-labelledby= "summary-heading" class = "mt-16 rounded-lg bg-gray-50 px-4 py-6 sm:p-6 lg:col-span-5 lg:mt-0 lg:p-8" > <h2 id= "summary-heading" class = "text-lg font-medium text-gray-900" >Order summary</h2> <dl class = "mt-6 space-y-4" > <div class = "flex items-center justify-between" > <dt class = "text-sm text-gray-600" >Subtotal</dt> <dd class = "text-sm font-medium text-gray-900" > $129 </dd> </div> <div class = "flex items-center justify-between border-t border-gray-200 pt-4" > <dt class = "flex items-center text-sm text-gray-600" > <span>Shipping estimate</span> <a href= "#" class = "ml-2 flex-shrink-0 text-gray-400 hover:text-gray-500" > <span class = "sr-only" >Learn more about how shipping is calculated</span> <!-- Heroicon name: mini/question-mark-circle --> <svg class = "h-5 w-5" xmlns= "http://www.w3.org/2000/svg" viewBox= "0 0 20 20" fill= "currentColor" aria-hidden= "true" > <path fill-rule= "evenodd" d= "M18 10a8 8 0 11-16 0 8 8 0 0116 0zM8.94 6.94a.75.75 0 11-1.061-1.061 3 3 0 112.871 5.026v.345a.75.75 0 01-1.5 0v-.5c0-.72.57-1.172 1.081-1.287A1.5 1.5 0 108.94 6.94zM10 15a1 1 0 100-2 1 1 0 000 2z" clip-rule= "evenodd" /> </svg> </a> </dt> <dd class = "text-sm font-medium text-gray-900" > $0 .00</dd> </div> <div class = "flex items-center justify-between border-t border-gray-200 pt-4" > <dt class = "text-base font-medium text-gray-900" >Order total</dt> <dd class = "text-base font-medium text-gray-900" > $129 </dd> </div> </dl> <div class = "mt-6" > <button type= "submit" class = "w-full rounded-md border border-transparent bg-indigo-600 py-3 px-4 text-base font-medium text-white shadow-sm hover:bg-indigo-700 focus:outline-none focus:ring-2 focus:ring-indigo-500 focus:ring-offset-2 focus:ring-offset-gray-50" >Checkout</button> </div> <div class = "mt-6 text-center text-sm" > <p> or <a href= "http://localhost:8000/shop" class = "font-medium text-indigo-600 hover:text-indigo-500" > Continue Shopping <span aria-hidden= "true" > →</span> </a> </p> </div> </section> </form> </div> </div> </main> <footer aria-labelledby= "footer-heading" class = "bg-white" > <h2 id= "footer-heading" class = "sr-only" >Footer</h2> <div class = "mx-auto max-w-7xl px-4 sm:px-6 lg:px-8" > <div class = "border-t border-gray-200 py-20" > <div class = "grid grid-cols-1 md:grid-flow-col md:auto-rows-min md:grid-cols-12 md:gap-x-8 md:gap-y-16" > <!-- Image section --> <div class = "col-span-1 md:col-span-2 lg:col-start-1 lg:row-start-1" > <img src= "https://tailwindui.com/img/logos/mark.svg?color=indigo&shade=600" alt= "" class = "h-8 w-auto" > </div> <!-- Sitemap sections --> <div class = "col-span-6 mt-10 grid grid-cols-2 gap-8 sm:grid-cols-3 md:col-span-8 md:col-start-3 md:row-start-1 md:mt-0 lg:col-span-6 lg:col-start-2" > <div class = "grid grid-cols-1 gap-y-12 sm:col-span-2 sm:grid-cols-2 sm:gap-x-8" > <div> <h3 class = "text-sm font-medium text-gray-900" >Products</h3> <ul role= "list" class = "mt-6 space-y-6" > <li class = "text-sm" > <a href= "#" class = "text-gray-500 hover:text-gray-600" >Bags</a> </li> <li class = "text-sm" > <a href= "#" class = "text-gray-500 hover:text-gray-600" >Tees</a> </li> <li class = "text-sm" > <a href= "#" class = "text-gray-500 hover:text-gray-600" >Home Goods</a> </li> </ul> </div> <div> <h3 class = "text-sm font-medium text-gray-900" >Company</h3> <ul role= "list" class = "mt-6 space-y-6" > <li class = "text-sm" > <a href= "#" class = "text-gray-500 hover:text-gray-600" >Who we are</a> </li> <li class = "text-sm" > <a href= "#" class = "text-gray-500 hover:text-gray-600" >Terms & Conditions</a> </li> <li class = "text-sm" > <a href= "#" class = "text-gray-500 hover:text-gray-600" >Privacy</a> </li> </ul> </div> </div> <div> <h3 class = "text-sm font-medium text-gray-900" >Customer Service</h3> <ul role= "list" class = "mt-6 space-y-6" > <li class = "text-sm" > <a href= "#" class = "text-gray-500 hover:text-gray-600" >Contact</a> </li> <li class = "text-sm" > <a href= "#" class = "text-gray-500 hover:text-gray-600" >Shipping</a> </li> <li class = "text-sm" > <a href= "#" class = "text-gray-500 hover:text-gray-600" >Returns</a> </li> </ul> </div> </div> <!-- Newsletter section --> <div class = "mt-12 md:col-span-8 md:col-start-3 md:row-start-2 md:mt-0 lg:col-span-4 lg:col-start-9 lg:row-start-1" > <h3 class = "text-sm font-medium text-gray-900" >Sign up for our newsletter</h3> <p class = "mt-6 text-sm text-gray-500" >The latest deals and savings, sent to your inbox weekly.</p> <form class = "mt-2 flex sm:max-w-md" > <label for = "email-address" class = "sr-only" >Email address</label> <input id= "email-address" type= "text" autocomplete= "email" required= "" class = "w-full min-w-0 appearance-none rounded-md border border-gray-300 bg-white py-2 px-4 text-base text-gray-900 placeholder-gray-500 shadow-sm focus:border-indigo-500 focus:outline-none focus:ring-1 focus:ring-indigo-500" > <div class = "ml-4 flex-shrink-0" > <button type= "submit" class = "flex w-full items-center justify-center rounded-md border border-transparent bg-indigo-600 py-2 px-4 text-base font-medium text-white shadow-sm hover:bg-indigo-700 focus:outline-none focus:ring-2 focus:ring-indigo-500 focus:ring-offset-2" >Sign up</button> </div> </form> </div> </div> </div> <div class = "border-t border-gray-100 py-10 text-center" > <p class = "text-sm text-gray-500" >© 2021 Your Company, Inc. All rights reserved.</p> </div> </div> </footer> </div> </body> </html> |
প্রথমে আমরা Layout তৈরির জন্য নিম্নোক্ত Blade Component গুলো তৈরি করব:
1 2 3 4 5 | php artisan make:component header php artisan make:component alert php artisan make:component main php artisan make:component footer php artisan make:component notice |
এখন আমরা মূল HTML File থেকে সংশ্লিষ্ট কোড গুলো উপরোক্ত কম্পোনেন্ট গুলোতে যোগ করব:
resources/views/component/header.blade.php
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 | <nav aria-label= "Top" class = "mx-auto max-w-7xl px-4 sm:px-6 lg:px-8" > <div class = "border-b border-gray-200" > <div class = "flex h-16 items-center" > <button type= "button" x-description= "Mobile menu toggle, controls the 'mobileMenuOpen' state." class = "rounded-md bg-white p-2 text-gray-400 lg:hidden" @click= "open = true" > <span class = "sr-only" >Open menu</span> <svg class = "h-6 w-6" x-description= "Heroicon name: outline/bars-3" xmlns= "http://www.w3.org/2000/svg" fill= "none" viewBox= "0 0 24 24" stroke-width= "1.5" stroke= "currentColor" aria-hidden= "true" > <path stroke-linecap= "round" stroke-linejoin= "round" d= "M3.75 6.75h16.5M3.75 12h16.5m-16.5 5.25h16.5" ></path> </svg> </button> <!-- Logo --> <div class = "ml-4 flex lg:ml-0" > <a href= "{{ url('/') }}" > <img class = "h-8 w-auto" src= "https://tailwindui.com/img/logos/workflow-mark.svg?color=indigo&shade=600" alt= "" > </a> </div> <!-- Flyout menus --> <div class = "hidden lg:ml-8 lg:block lg:self-stretch" > <div class = "flex h-full space-x-8" > <a href= "#" class = "flex items-center text-sm font-medium text-gray-700 hover:text-gray-800" >Women</a> <a href= "#" class = "flex items-center text-sm font-medium text-gray-700 hover:text-gray-800" >Men</a> <a href= "#" class = "flex items-center text-sm font-medium text-gray-700 hover:text-gray-800" >Company</a> <a href= "#" class = "flex items-center text-sm font-medium text-gray-700 hover:text-gray-800" >Stores</a> </div> </div> <div class = "ml-auto flex items-center" > <div class = "hidden lg:flex lg:flex-1 lg:items-center lg:justify-end lg:space-x-6" > <a href= "#" class = "text-sm font-medium text-gray-700 hover:text-gray-800" >Sign in</a> <span class = "h-6 w-px bg-gray-200" aria-hidden= "true" ></span> <a href= "#" class = "text-sm font-medium text-gray-700 hover:text-gray-800" >Create account</a> </div> @php $total = 0 @endphp @ foreach (( array ) session( 'cart' ) as $id => $details ) @php $total += $details [ 'price' ] * $details [ 'quantity' ] @endphp @ endforeach <!-- Cart --> <div class = "ml-4 flow-root lg:ml-6" > <a href= "{{ route('cart') }}" class = "group -m-2 flex items-center p-2" > <svg class = "h-6 w-6 flex-shrink-0 text-gray-400 group-hover:text-gray-500" x-description= "Heroicon name: outline/shopping-bag" xmlns= "http://www.w3.org/2000/svg" fill= "none" viewBox= "0 0 24 24" stroke-width= "1.5" stroke= "currentColor" aria-hidden= "true" > <path stroke-linecap= "round" stroke-linejoin= "round" d= "M15.75 10.5V6a3.75 3.75 0 10-7.5 0v4.5m11.356-1.993l1.263 12c.07.665-.45 1.243-1.119 1.243H4.25a1.125 1.125 0 01-1.12-1.243l1.264-12A1.125 1.125 0 015.513 7.5h12.974c.576 0 1.059.435 1.119 1.007zM8.625 10.5a.375.375 0 11-.75 0 .375.375 0 01.75 0zm7.5 0a.375.375 0 11-.75 0 .375.375 0 01.75 0z" ></path> </svg> <span class = "ml-2 text-sm font-medium text-gray-700 group-hover:text-gray-800" >$ {{ $total }}</span> <span class = "sr-only" >items in cart, view bag</span> </a> </div> </div> </div> </div> </nav> |
resources/views/component/footer.blade.php
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 | <footer aria-labelledby= "footer-heading" class = "bg-white" > <h2 id= "footer-heading" class = "sr-only" >Footer</h2> <div class = "mx-auto max-w-7xl px-4 sm:px-6 lg:px-8" > <div class = "border-t border-gray-200 py-20" > <div class = "grid grid-cols-1 md:grid-flow-col md:auto-rows-min md:grid-cols-12 md:gap-x-8 md:gap-y-16" > <!-- Image section --> <div class = "col-span-1 md:col-span-2 lg:col-start-1 lg:row-start-1" > <img src= "https://tailwindui.com/img/logos/mark.svg?color=indigo&shade=600" alt= "" class = "h-8 w-auto" > </div> <!-- Sitemap sections --> <div class = "col-span-6 mt-10 grid grid-cols-2 gap-8 sm:grid-cols-3 md:col-span-8 md:col-start-3 md:row-start-1 md:mt-0 lg:col-span-6 lg:col-start-2" > <div class = "grid grid-cols-1 gap-y-12 sm:col-span-2 sm:grid-cols-2 sm:gap-x-8" > <div> <h3 class = "text-sm font-medium text-gray-900" >Products</h3> <ul role= "list" class = "mt-6 space-y-6" > <li class = "text-sm" > <a href= "#" class = "text-gray-500 hover:text-gray-600" >Bags</a> </li> <li class = "text-sm" > <a href= "#" class = "text-gray-500 hover:text-gray-600" >Tees</a> </li> <li class = "text-sm" > <a href= "#" class = "text-gray-500 hover:text-gray-600" >Home Goods</a> </li> </ul> </div> <div> <h3 class = "text-sm font-medium text-gray-900" >Company</h3> <ul role= "list" class = "mt-6 space-y-6" > <li class = "text-sm" > <a href= "#" class = "text-gray-500 hover:text-gray-600" >Who we are</a> </li> <li class = "text-sm" > <a href= "#" class = "text-gray-500 hover:text-gray-600" >Terms & Conditions</a> </li> <li class = "text-sm" > <a href= "#" class = "text-gray-500 hover:text-gray-600" >Privacy</a> </li> </ul> </div> </div> <div> <h3 class = "text-sm font-medium text-gray-900" >Customer Service</h3> <ul role= "list" class = "mt-6 space-y-6" > <li class = "text-sm" > <a href= "#" class = "text-gray-500 hover:text-gray-600" >Contact</a> </li> <li class = "text-sm" > <a href= "#" class = "text-gray-500 hover:text-gray-600" >Shipping</a> </li> <li class = "text-sm" > <a href= "#" class = "text-gray-500 hover:text-gray-600" >Returns</a> </li> </ul> </div> </div> <!-- Newsletter section --> <div class = "mt-12 md:col-span-8 md:col-start-3 md:row-start-2 md:mt-0 lg:col-span-4 lg:col-start-9 lg:row-start-1" > <h3 class = "text-sm font-medium text-gray-900" >Sign up for our newsletter</h3> <p class = "mt-6 text-sm text-gray-500" >The latest deals and savings, sent to your inbox weekly.</p> <form class = "mt-2 flex sm:max-w-md" > <label for = "email-address" class = "sr-only" >Email address</label> <input id= "email-address" type= "text" autocomplete= "email" required= "" class = "w-full min-w-0 appearance-none rounded-md border border-gray-300 bg-white py-2 px-4 text-base text-gray-900 placeholder-gray-500 shadow-sm focus:border-indigo-500 focus:outline-none focus:ring-1 focus:ring-indigo-500" > <div class = "ml-4 flex-shrink-0" > <button type= "submit" class = "flex w-full items-center justify-center rounded-md border border-transparent bg-indigo-600 py-2 px-4 text-base font-medium text-white shadow-sm hover:bg-indigo-700 focus:outline-none focus:ring-2 focus:ring-indigo-500 focus:ring-offset-2" >Sign up</button> </div> </form> </div> </div> </div> <div class = "border-t border-gray-100 py-10 text-center" > <p class = "text-sm text-gray-500" >© 2021 Your Company, Inc. All rights reserved.</p> </div> </div> </footer> |
resources/views/component/alert.blade.php
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 | @ if (session( 'success' )) <div id= "alert-3" class = "mx-auto pointer-events-auto w-full max-w-sm overflow-hidden rounded-lg bg-white shadow-lg ring-1 ring-black ring-opacity-5" > <div class = "p-4" > <div class = "flex items-start" > <div class = "flex-shrink-0" > <!-- Heroicon name: outline/check-circle --> <svg class = "h-6 w-6 text-green-400" xmlns= "http://www.w3.org/2000/svg" fill= "none" viewBox= "0 0 24 24" stroke-width= "1.5" stroke= "currentColor" aria-hidden= "true" > <path stroke-linecap= "round" stroke-linejoin= "round" d= "M9 12.75L11.25 15 15 9.75M21 12a9 9 0 11-18 0 9 9 0 0118 0z" /> </svg> </div> <div class = "ml-3 w-0 flex-1 pt-0.5" > <p class = "text-sm font-medium text-gray-900" >{{ session( 'success' ) }}</p> </div> <div class = "ml-4 flex flex-shrink-0" > <button type= "button" class = "inline-flex rounded-md bg-white text-gray-400 hover:text-gray-500 focus:outline-none focus:ring-2 focus:ring-indigo-500 focus:ring-offset-2" data-dismiss-target= "#alert-3" aria-label= "Close" > <span class = "sr-only" >Close</span> <!-- Heroicon name: mini/x-mark --> <svg class = "h-5 w-5" xmlns= "http://www.w3.org/2000/svg" viewBox= "0 0 20 20" fill= "currentColor" aria-hidden= "true" > <path d= "M6.28 5.22a.75.75 0 00-1.06 1.06L8.94 10l-3.72 3.72a.75.75 0 101.06 1.06L10 11.06l3.72 3.72a.75.75 0 101.06-1.06L11.06 10l3.72-3.72a.75.75 0 00-1.06-1.06L10 8.94 6.28 5.22z" /> </svg> </button> </div> </div> </div> </div> </div> </div> @ endif |
resources/views/component/notic.blade.php
1 2 3 | <p class = "flex h-10 items-center justify-center bg-indigo-600 px-4 text-sm font-medium text-white sm:px-6 lg:px-8" > Get free delivery on orders over $100 </p> |
resources/views/component/main.blade.php
1 2 3 | <main class = "mx-auto max-w-2xl px-4 pt-16 pb-24 sm:px-6 lg:max-w-7xl lg:px-8" > {{ $slot }} </main> |
Creating Master Blade
এবার resources/views ফোল্ডারের মধ্যে master.blade.php নামে একটি ফাইল তৈরি করব, এবং নিচের মতো করে component গুলোকে যুক্ত করে দিবো।
resources/views/master.blade.php
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 | <!doctype html> <html> <head> <meta charset= "UTF-8" > <meta name= "viewport" content= "width=device-width, initial-scale=1.0" > <script src= "https://cdn.tailwindcss.com" ></script> <script src= "https://unpkg.com/flowbite@1.5.3/dist/flowbite.js" ></script> <script src= "https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js" ></script> <title>@ yield ( 'title' )</title> </head> <body> <div class = "bg-white" > <x-notice></x-notice> <x-nav></x-nav> <x-alert></x-alert> <x-main>@ yield ( 'content' )</x-main> <x-footer></x-footer> </div> @ yield ( 'scripts' ) </body> </html> |
Creating Pages
এবার আমরা resources/views/pages ফোল্ডারের মধ্যে productlist.blade.php এবং cart.blade.php নামে দুটি ব্লেড ফাইল তৈরি করব, এবং নিচের মতো করে কোডগুলোকে গুলোকে যুক্ত করে দিবো।
resources/views/pages/productlist.blade.php
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 | @ extends ( 'master' ) @section( 'title' , 'Products List' ) @section( 'content' ) <div class = "mx-auto max-w-7xl mt-6 grid grid-cols-2 gap-x-4 gap-y-10 sm:gap-x-6 md:grid-cols-4 md:gap-y-0 lg:gap-x-8" > @ foreach ( $products as $product ) <div class = "group relative" > <div class = "h-56 w-full overflow-hidden rounded-md bg-gray-200 group-hover:opacity-75 lg:h-72 xl:h-80" > <img src= "{{ $product->image }}" alt= "{{ $product->name }}" class = "h-full w-full object-cover object-center" > </div> <h3 class = "mt-4 text-sm text-gray-700" > <a href= "#" > <span class = "absolute inset-0" ></span> {{ $product ->name }} </a> </h3> <p class = "mt-1 text-sm text-gray-500" >{{ $product ->description }}</p> <p class = "mt-1 text-sm font-medium text-gray-900" >${{ $product ->price }}</p> <div class = "mt-6" > <a href= "{{ route('add.to.cart', $product->id) }}" class = "relative flex items-center justify-center rounded-md border border-transparent bg-gray-100 py-2 px-8 text-sm font-medium text-gray-900 hover:bg-gray-200" >Add to bag<span class = "sr-only" >, Halfsize Tote</span></a> </div> </div> @ endforeach </div> @endsection |
resources/views/pages/cart.blade.php
001 002 003 004 005 006 007 008 009 010 011 012 013 014 015 016 017 018 019 020 021 022 023 024 025 026 027 028 029 030 031 032 033 034 035 036 037 038 039 040 041 042 043 044 045 046 047 048 049 050 051 052 053 054 055 056 057 058 059 060 061 062 063 064 065 066 067 068 069 070 071 072 073 074 075 076 077 078 079 080 081 082 083 084 085 086 087 088 089 090 091 092 093 094 095 096 097 098 099 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 | @ extends ( 'master' ) @section( 'title' , 'Shopping Cart' ) @section( 'content' ) <div class = "bg-white" > <div class = "mx-auto max-w-2xl px-4 pt-16 pb-24 sm:px-6 lg:max-w-7xl lg:px-8" > <h2 class = "text-3xl font-bold tracking-tight text-gray-900 sm:text-4xl" >Shopping Cart</h2> <form class = "mt-12 lg:grid lg:grid-cols-12 lg:items-start lg:gap-x-12 xl:gap-x-16" > <section aria-labelledby= "cart-heading" class = "lg:col-span-7" > <h2 id= "cart-heading" class = "sr-only" >Items in your shopping cart</h2> <ul id= "cart" role= "list" class = "divide-y divide-gray-200 border-t border-b border-gray-200" > @php $total = 0 @endphp @ if (session( 'cart' )) @ foreach (session( 'cart' ) as $id => $details ) @php $total += $details [ 'price' ] * $details [ 'quantity' ] @endphp <li class = "flex py-6 sm:py-10" data-id= "{{ $id }}" > <div class = "flex-shrink-0" > <img src= "{{ $details['image'] }}" alt= "Front of men's Basic Tee in sienna." class = "h-24 w-24 rounded-md object-cover object-center sm:h-48 sm:w-48" > </div> <div class = "ml-4 flex flex-1 flex-col justify-between sm:ml-6" > <div class = "relative pr-9 sm:grid sm:grid-cols-2 sm:gap-x-6 sm:pr-0" > <div> <div class = "flex justify-between" > <h3 class = "text-sm" > <a href= "#" class = "font-medium text-gray-700 hover:text-gray-800" >{{ $details [ 'name' ] }}</a> </h3> </div> <div class = "mt-1 flex text-sm" > <p class = "text-gray-500" >Sienna</p> <p class = "ml-4 border-l border-gray-200 pl-4 text-gray-500" >Large</p> </div> <p class = "mt-1 text-sm font-medium text-gray-900" >${{ $details [ 'price' ] }}</p> </div> <div class = "mt-4 sm:mt-0 sm:pr-9" > <label for = "quantity-0" class = "sr-only" >Quantity, Basic Tee</label> <input type= "number" value= "{{ $details['quantity'] }}" class = "quantity update-cart w-1/3 rounded-md border border-gray-300 py-1.5 text-left text-base font-medium leading-5 text-gray-700 shadow-sm focus:border-indigo-500 focus:outline-none focus:ring-1 focus:ring-indigo-500 sm:text-sm" /> <div class = "actions absolute top-0 right-0" > <button type= "button" class = "remove-from-cart -m-2 inline-flex p-2 text-gray-400 hover:text-gray-500" > <span class = "sr-only " >Remove</span> <!-- Heroicon name: mini/x-mark --> <svg class = "h-5 w-5" xmlns= "http://www.w3.org/2000/svg" viewBox= "0 0 20 20" fill= "currentColor" aria-hidden= "true" > <path d= "M6.28 5.22a.75.75 0 00-1.06 1.06L8.94 10l-3.72 3.72a.75.75 0 101.06 1.06L10 11.06l3.72 3.72a.75.75 0 101.06-1.06L11.06 10l3.72-3.72a.75.75 0 00-1.06-1.06L10 8.94 6.28 5.22z" /> </svg> </button> </div> </div> </div> </div> </li> @ endforeach @ endif </ul> </section> <!-- Order summary --> <section aria-labelledby= "summary-heading" class = "mt-16 rounded-lg bg-gray-50 px-4 py-6 sm:p-6 lg:col-span-5 lg:mt-0 lg:p-8" > <h2 id= "summary-heading" class = "text-lg font-medium text-gray-900" >Order summary</h2> <dl class = "mt-6 space-y-4" > <div class = "flex items-center justify-between" > <dt class = "text-sm text-gray-600" >Subtotal</dt> <dd class = "text-sm font-medium text-gray-900" > ${{ $total }} </dd> </div> <div class = "flex items-center justify-between border-t border-gray-200 pt-4" > <dt class = "flex items-center text-sm text-gray-600" > <span>Shipping estimate</span> <a href= "#" class = "ml-2 flex-shrink-0 text-gray-400 hover:text-gray-500" > <span class = "sr-only" >Learn more about how shipping is calculated</span> <!-- Heroicon name: mini/question-mark-circle --> <svg class = "h-5 w-5" xmlns= "http://www.w3.org/2000/svg" viewBox= "0 0 20 20" fill= "currentColor" aria-hidden= "true" > <path fill-rule= "evenodd" d= "M18 10a8 8 0 11-16 0 8 8 0 0116 0zM8.94 6.94a.75.75 0 11-1.061-1.061 3 3 0 112.871 5.026v.345a.75.75 0 01-1.5 0v-.5c0-.72.57-1.172 1.081-1.287A1.5 1.5 0 108.94 6.94zM10 15a1 1 0 100-2 1 1 0 000 2z" clip-rule= "evenodd" /> </svg> </a> </dt> <dd class = "text-sm font-medium text-gray-900" > $0 .00</dd> </div> <div class = "flex items-center justify-between border-t border-gray-200 pt-4" > <dt class = "text-base font-medium text-gray-900" >Order total</dt> <dd class = "text-base font-medium text-gray-900" >${{ $total }}</dd> </div> </dl> <div class = "mt-6" > <button type= "submit" class = "w-full rounded-md border border-transparent bg-indigo-600 py-3 px-4 text-base font-medium text-white shadow-sm hover:bg-indigo-700 focus:outline-none focus:ring-2 focus:ring-indigo-500 focus:ring-offset-2 focus:ring-offset-gray-50" >Checkout</button> </div> <div class = "mt-6 text-center text-sm" > <p> or <a href= "{{ url('/') }}" class = "font-medium text-indigo-600 hover:text-indigo-500" > Continue Shopping <span aria-hidden= "true" > →</span> </a> </p> </div> </section> </form> </div> </div> @endsection @section( 'scripts' ) <script type= "text/javascript" > $( ".update-cart" ).change( function (e) { e.preventDefault(); var ele = $(this); $.ajax({ url: '{{ route(' update.cart ') }}' , method: "patch" , data: { _token: '{{ csrf_token() }}' , id: ele.parents( "li" ).attr( "data-id" ), quantity: ele.parents( "li" ).find( ".quantity" ).val() }, success: function (response) { window.location.reload(); } }); }); $( ".remove-from-cart" ).click( function (e) { e.preventDefault(); var ele = $(this); if (confirm( "Are you sure want to remove?" )) { $.ajax({ url: "{{ route('remove.from.cart') }}" , method: "DELETE" , data: { _token: '{{ csrf_token() }}' , id: ele.parents( "li" ).attr( "data-id" ) }, success: function (response) { window.location.reload(); } }); } }); </script> @endsection |
এবার আপনার route file web.php তে নিচের মতো করে route গুলো ডিফাইন করে দিন।
1 2 3 4 5 6 7 | use App\Http\Controllers\ProductController; Route::get( '/' , [ProductController:: class , 'index' ]); Route::get( '/cart' , [ProductController:: class , 'cart' ])->name( 'cart' ); Route::get( '/add-to-cart/{id}' , [ProductController:: class , 'addToCart' ])->name( 'add.to.cart' ); Route::patch( '/update-cart' , [ProductController:: class , 'update' ])->name( 'update.cart' ); Route:: delete ( '/remove-from-cart' , [ProductController:: class , 'remove' ])->name( 'remove.from.cart' ); |
তো হয়েগেলো , এখন আপনি ব্রাউজারে http://localhost:8000 হিট করলেই একটি কার্যকর এবং প্রফেশনাল shopping cart দেখতে পাবেন।