Laravel Database Query Builder
LARAVEL DATABASE QUERY BUILDER PART-4: Laravel where Clauses Basic to Advance
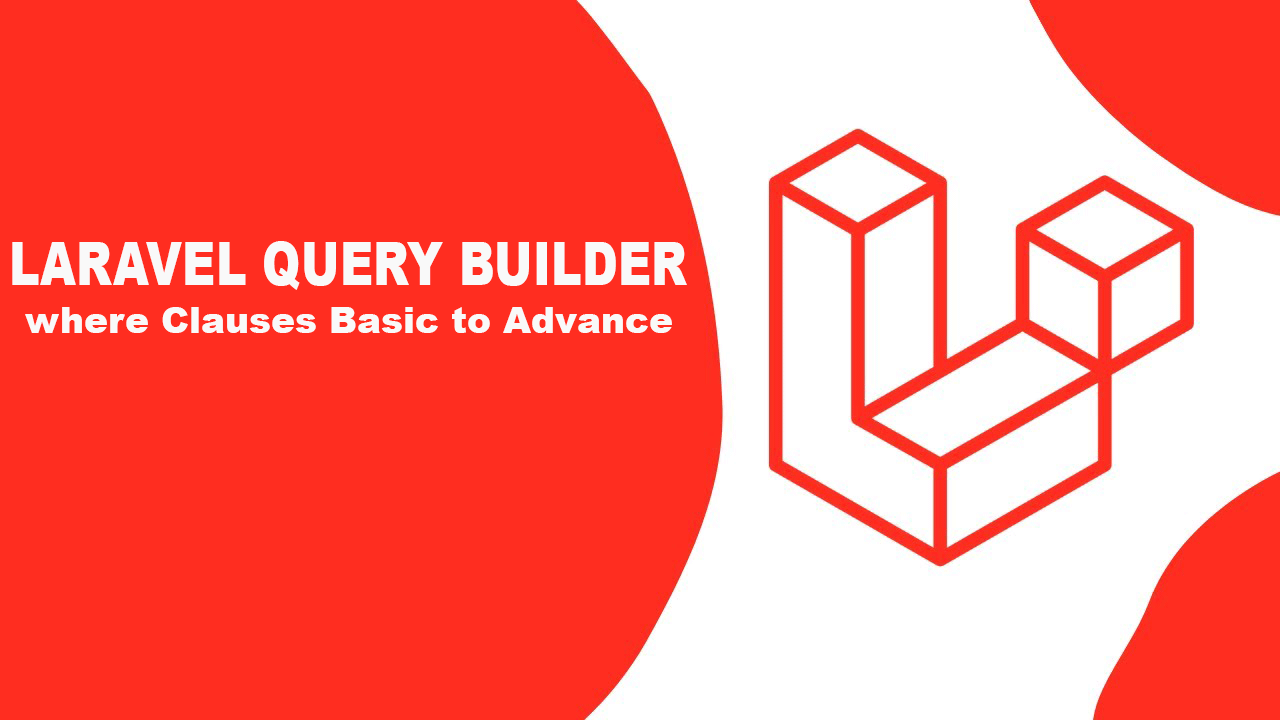
এই পর্বে আমরা Laravel Query Builder দিয়ে Database এর যেকোনো table থেকে Data Select ব্যবহার করার সময় Where এবং Conditional clause এর ব্যবহার শিখবো।
তবে আপনি SELECT স্টেটমেন্ট ছাড়াও, আপনি কোন rows গুলি আপডেট বা মুছতে হবে তা নির্দিষ্ট করতে UPDATE বা DELETE statement এ ও WHERE ক্লজটি ব্যবহার করতে পারেন ।
আমরা নিম্নোক্ত Table Structure এ where Clause এর ব্যবহার দেখব।
-- -- Table structure for table `employees` -- CREATE TABLE `employees` ( `id` bigint(20) UNSIGNED NOT NULL, `name` varchar(255) NOT NULL, `email` varchar(100) NOT NULL, `phone` varchar(20) NOT NULL, `age` float NOT NULL, `status` enum('Pending','Wait','Awarded') NOT NULL DEFAULT 'Pending', `created_at` timestamp NULL DEFAULT NULL, `updated_at` timestamp NULL DEFAULT NULL ) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_unicode_ci; -- -- Dumping data for table `employees` -- INSERT INTO `employees` (`id`, `name`, `email`, `phone`, `age`, `status`, `created_at`, `updated_at`) VALUES (1, 'Incidunt officia.', 'incidunt@mail.com', '01855665544', 24, 'Awarded', '2023-01-11 06:51:10', '2023-01-11 06:51:10'), (2, 'Quidem enim qui.', 'enim@mail.com', '01455887799', 25.5, 'Pending', '2023-01-11 06:51:10', '2023-01-11 06:51:10'), (3, 'Consequatur eaque.', 'eaque@gmail.com', '01855996633', 26, 'Wait', '2023-01-11 06:51:10', '2023-01-11 06:51:10'), (4, 'Qui nihil dolorem.', 'nihil@mail.com', '01877996655', 27.5, 'Awarded', '2023-01-11 06:51:10', '2023-01-11 06:51:10'), (5, 'Odio ea dolorem.', 'odio@mail.com', '01799665544', 22, 'Pending', '2023-01-11 06:51:10', '2023-01-11 06:51:10'), (6, 'Id aut tempore.', 'aiaut@mail.com', '01788996655', 23, 'Wait', '2023-01-11 06:51:10', '2023-01-11 06:51:10'), (7, 'Mollitia saepe ipsa.', 'mollitia@mail.com', '01988665544', 25, 'Awarded', '2023-01-11 06:51:10', '2023-01-11 06:51:10'), (8, 'Tempore rerum eum.', 'eum@mail.com', '01966885566', 22.5, 'Pending', '2023-01-11 06:51:10', '2023-01-11 06:51:10'), (9, 'Illum accusantium.', 'illum@gmail.com', '01655447788', 18, 'Wait', '2023-01-11 06:51:10', '2023-01-11 06:51:10'), (10, 'Dignissimos dolore.', 'dolore@gmail.com', '01855669911', 20, 'Pending', '2023-01-11 06:51:10', '2023-01-11 06:51:10'); -- -- Indexes for dumped tables -- -- -- Indexes for table `employees` -- ALTER TABLE `employees` ADD PRIMARY KEY (`id`); -- -- AUTO_INCREMENT for dumped tables -- -- -- AUTO_INCREMENT for table `employees` -- ALTER TABLE `employees` MODIFY `id` bigint(20) UNSIGNED NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=1;
Where Clause Basics
query তে “where” clauses যোগ করার জন্য আপনি query builder’s where method ব্যবহার করতে পারেন। where method এ তিনটি আর্গুমেন্ট দিতে হয়। প্রথম argument টি কলামের নাম। দ্বিতীয় argument টি হল একটি অপারেটর, যেটি ডাটাবেসের সমর্থিত অপারেটরগুলির যেকোনো একটি হতে পারে। তৃতীয় argument হল কলামের ভ্যালু এর সাথে তুলনা (compare ) করার মান।
where method কে একটার সাথে আরেকটার চেইনিং কল করা হয় , তখন where method, AND অপারেটর ব্যবহার করে।
উদাহরণস্বরূপ, নিম্নলিখিত query দ্বারা সেই সব এমপ্লয়ীদের retrieve করব যাদের status কলামের মান Awarded এবং বয়স কলামের মান 25 অথবা -এর বেশি:
use Illuminate\Support\Facades\DB; Route::get('/query', function () { $employees = DB::table('employees') ->where('status', '=', 'Awarded') ->where('age', '>=', 25) ->get(); echo "<pre>"; print_r($employees); echo "</pre>"; });
উপরের উদাহরণটি নিম্নলিখিত SQL তৈরি করবে:
select * from `employees` where `status` = 'Awarded' and `age` >= 25
সুবিধার জন্য, আপনি যদি যাচাই করতে চান যে একটি column = একটি value এর সাথে, আপনি value টিকে where method এ দ্বিতীয় আর্গুমেন্ট হিসাবে পাস করতে পারেন। লারাভেল ধরে নিবে আপনি = অপারেটর ব্যবহার করতে চান:
use Illuminate\Support\Facades\DB; Route::get('/query', function () { $employees = DB::table('employees') ->where('status','Awarded') ->where('age', '>=', 25) ->get(); echo "<pre>"; print_r($employees); echo "</pre>";
উপরের উদাহরণটি নিম্নলিখিত SQL তৈরি করবে:
select * from `employees` where `status` = 'Awarded' and `age` >= 25
যেটা আমি পূর্বেই বলেছি, আপনি আপনার ডাটাবেস সিস্টেম এ সমর্থিত যে কোনো Operater ই where clause এ ব্যবহার করতে পারেন:
$employees= DB::table('employees') ->where('age', '>=', 20) ->get(); $employees= DB::table('employees') ->where('age', '<>', 23) ->get(); $employees= DB::table('employees') ->where('name', 'like', 'T%') ->get();
আপনি where ফাংশনে conditions গুলির একটি array pass করতে পারেন। অ্যারের প্রতিটি উপাদান একটি অ্যারে হওয়া উচিত যেখানে where method এ তিনটি arguments সাধারণত pass করা হয়:
use Illuminate\Support\Facades\DB; Route::get('/query', function () { $employees = DB::table('employees')->where([ ['status', '=', 'Awarded'], ['age', '<>', '25'], ])->get(); echo "<pre>"; print_r($employees); echo "</pre>"; });
উপরের উদাহরণটি নিম্নলিখিত SQL তৈরি করবে:
select * from `employees` where (`status` = 'Awarded' and `age` <> '25')
Or Where Clauses
Laravel query builder এ যখন where method কে একটার সাথে আরেকটার চেইনিং কল করা হয় , তখন where method AND অপারেটর ব্যবহার করে। তবে, আপনি চাইলে ক্যোয়ারীতে or operator ব্যবহার করার জন্য এ orWhere method ব্যবহার করতে পারেন। orWhere method, where method এর মত একই আর্গুমেন্ট গ্রহণ করে:
use Illuminate\Support\Facades\DB; Route::get('/query', function () { $employees = DB::table('employees') ->where('status', '=', 'Awarded') ->orWhere('age', '>=', 25) ->get(); echo "<pre>"; print_r($employees); echo "</pre>"; });
উপরের উদাহরণটি নিম্নলিখিত SQL তৈরি করবে:
select * from `employees` where `status` = 'Awarded' or `age` >= 25
আপনি যদি parentheses এর মধ্যে একটি “or” condition কে group করতে চান, তাহলে আপনি orWhere method এ first argument হিসাবে একটি closure পাস করতে পারেন:
use Illuminate\Support\Facades\DB; use Illuminate\Database\Query\Builder; Route::get('/query', function () { $employees = DB::table('employees') ->where('age', '>', 25) ->orWhere(function(Builder $query) { $query->where('age', '>',20) ->where('status', 'Awarded'); }) ->get(); echo "<pre>"; print_r($employees); echo "</pre>"; });
উপরের উদাহরণটি নিম্নলিখিত SQL তৈরি করবে:
select * from `employees` where `age` > 25 or (`age` > 20 and `status` = 'Awarded')
Where Not Clauses
whereNot এবং orWhereNot methods গুলি একটি প্রদত্ত query constraints কে negate করতে ব্যবহার করা যেতে পারে। উদাহরণ স্বরূপ, নিম্নলিখিত ক্যোয়ারীতে এমন employee দের বাদ দেওয়া হয়েছে যেগুলি age ২৪ এর উপরে রয়েছে বা যার status এখনো waiting এ আছে :
use Illuminate\Support\Facades\DB; use Illuminate\Database\Query\Builder; Route::get('/query', function () { $employees = DB::table('employees') ->whereNot(function (Builder $query) { $query->where('status', 'Wait') ->orWhere('age', '>', 24); })->get(); echo "<pre>"; print_r($employees); echo "</pre>"; });
উপরের উদাহরণটি নিম্নলিখিত SQL তৈরি করবে:
select * from `employees` where not (`status` = 'Wait' or `age` > 24)
JSON Where Clauses
Laravel Framework ডাটাবেসে JSON column types অনুসন্ধানকে সমর্থন করে যা JSON column types কে সমর্থন করে। বর্তমানে, এর মধ্যে রয়েছে MySQL 5.7+, PostgreSQL, SQL Server 2016, এবং SQLite 3.39.0 (JSON1 এক্সটেনশন সহ)। একটি JSON কলাম query করতে, -> অপারেটর ব্যবহার করতে হয়।
JSON column Select বুঝার জন্য প্রথমে নিম্নোক্ত টেবিল স্ট্রাকচার এবং ডাটা গুলো এক্সেকিউট করে নিন :
-- -- Table structure for table `members` -- CREATE TABLE `members` ( `id` int(11) NOT NULL, `name` varchar(200) NOT NULL, `address` longtext CHARACTER SET utf8mb4 COLLATE utf8mb4_bin NOT NULL CHECK (json_valid(`address`)) ) ENGINE=InnoDB DEFAULT CHARSET=latin1 COLLATE=latin1_swedish_ci; -- -- Dumping data for table `members` -- INSERT INTO `members` (`id`, `name`, `address`) VALUES (1, 'Masud Alam', '{\"city\": \"Mumbai\", \"country\": \"India\"}'), (2, 'Sohel Alam', '{\"city\": \"Khulna\", \"country\": \"Bangladesh\"}'), (3, 'Rashedul Islam', '{\"city\": \"Barishal\", \"country\": \"India\"}'); -- -- Indexes for dumped tables -- -- -- Indexes for table `members` -- ALTER TABLE `members` ADD PRIMARY KEY (`id`); -- -- AUTO_INCREMENT for dumped tables -- -- -- AUTO_INCREMENT for table `members` -- ALTER TABLE `members` MODIFY `id` int(11) NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=1;
এবার নিম্নোক্ত কোড গুলো দিয়ে JSON column Select এর উদাহরণটি দেখে নিন :
use Illuminate\Support\Facades\DB; Route::get('/query', function () { $members = DB::table('members') ->where('address->country', 'India') ->get(); echo "<pre>"; print_r($members); echo "</pre>"; });
উপরের উদাহরণটি নিম্নলিখিত SQL তৈরি করবে:
select * from `members` where json_unquote(json_extract(`address`, '$."country"')) = 'India'
JSON অ্যারেগুলিকে query করতে আপনি whereJsonContains ব্যবহার করতে পারেন৷ তবে এই feature টি 3.38.0 এর নিচের SQLite ডাটাবেস সংস্করণ এ সমর্থন করবেনা
use Illuminate\Support\Facades\DB; Route::get('/query', function () { $members = DB::table('members') ->whereJsonContains('address->country', 'India') ->get(); echo "<pre>"; print_r($members); echo "</pre>"; });
উপরের উদাহরণটি নিম্নলিখিত SQL তৈরি করবে:
select * from `members` where json_contains(`address`, '\"India\"', '$."country"')
এছাড়াও আপনি JSON কলামের জন্য আপনার ক্যোয়ারীতে একাধিক AND conditions যোগ করতে Laravel-এ মাল্টিপল whereJsonContains method ব্যবহার করতে পারেন:
use Illuminate\Support\Facades\DB; Route::get('/query', function () { $members = DB::table('members') ->whereJsonContains('address->country', 'India') ->whereJsonContains('address->city', 'Mumbai') ->get(); echo "<pre>"; print_r($members); echo "</pre>"; });
উপরের উদাহরণটি নিম্নলিখিত SQL তৈরি করবে:
select * from `members` where json_contains(`address`, '\"India\"', '$."country"') and json_contains(`address`, '\"Mumbai\"', '$."city"')
এছাড়াও আপনি JSON কলামের জন্য আপনার ক্যোয়ারীতে একাধিক OR conditions যোগ করতে Laravel-এ মাল্টিপল orwhereJsonContains method ব্যবহার করতে পারেন:
use Illuminate\Support\Facades\DB; Route::get('/query', function () { $members = DB::table('members') ->orwhereJsonContains('address->country', 'India') ->orwhereJsonContains('address->city', 'Mumbai') ->get(); echo "<pre>"; print_r($members); echo "</pre>"; });
উপরের উদাহরণটি নিম্নলিখিত SQL তৈরি করবে:
select * from `members` where json_contains(`address`, '\"India\"', '$."country"') or json_contains(`address`, '\"Mumbai\"', '$."city"')
Additional Where Clauses
Additional Where Clauses সহ পরবর্তী উদাহরণ গুলোতে আমরা নিম্নোক্ত users টেবিল স্ট্রাকচার এবং তার ডেটা গুলো ব্যবহার করব :
-- -- Table structure for table `users` -- CREATE TABLE `users` ( `id` bigint(20) UNSIGNED NOT NULL, `first_name` varchar(32) NOT NULL, `last_name` varchar(32) NOT NULL, `email` varchar(64) NOT NULL, `phone` varchar(15) DEFAULT NULL, `address` varchar(200) DEFAULT NULL, `age` tinyint(4) NOT NULL, `role` enum('subscriber','author','moderator','admin') NOT NULL, `email_verified_at` varchar(255) DEFAULT NULL, `created_at` timestamp NULL DEFAULT NULL, `updated_at` timestamp NULL DEFAULT NULL, `active` enum('false','true') NOT NULL ) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_unicode_ci; -- -- Dumping data for table `users` -- INSERT INTO `users` (`id`, `first_name`, `last_name`, `email`, `phone`, `address`, `age`, `role`, `email_verified_at`, `created_at`, `updated_at`, `active`) VALUES (1, 'Faridur', 'Faridur', 'udare@example.org', '01946609792', '47980 Albin Circle Apt. 255\r\nNorth Theoberg, NE 73650', 23, 'moderator', '2023-02-13 13:01:39', '2023-02-06 01:38:05', '2023-02-08 07:44:28', 'true'), (2, 'Devin', ' Huel', 'morris.funk@example.org', '01946609792', 'Khulna,Bangladesh', 18, 'admin', '2023-02-13 13:01:39', '2023-02-06 01:38:05', '2023-02-06 01:38:05', 'true'), (3, 'Antonia', ' Rolfson', 'lowell.marquardt@example.com', '01955810564', '2004 Kelsi Parkways Suite 072\nPort Estevanborough, OR 42939', 20, 'author', '2023-02-13 13:01:39', '2023-02-06 01:38:05', '2023-02-06 01:38:05', 'false'), (4, 'Miss Eve ', 'Ullrich', 'bschaden@example.org', '01955810564', '5416 Hassan Parks\nNew Mohammadbury, OR 73376', 30, 'subscriber', '2023-02-13 13:01:39', '2023-02-06 01:38:05', '2023-02-06 01:38:05', 'true'), (5, 'Prof. Chance ', 'Swift', 'dennis06@example.com', '01955810564', '63655 Bashirian Groves\nEast Alisaburgh, IN 06538', 35, 'moderator', '2023-02-13 13:01:39', '2023-02-06 01:38:05', '2023-02-06 01:38:05', 'false'), (6, 'Prof. Roselyn ', 'Champlin MD', 'qbreitenberg@example.net', '01911896252', '33346 Kaylin Oval\nCeasarmouth, AK 12740', 28, 'subscriber', '2023-02-13 13:01:39', '2023-02-06 01:38:05', '2023-02-06 01:38:05', 'true'), (7, 'Felipa ', 'Rau', 'kayden61@example.org', '01911896252', '1271 Rosalinda Forge Apt. 845\nJosephburgh, TX 44744-0104', 45, 'subscriber', '2023-02-13 13:01:39', '2023-02-06 01:38:05', '2023-02-06 01:38:05', 'true'), (8, 'Soriful Islam', 'Soriful Islam', 'soriful.islam@example.org', NULL, 'Rajshahi,Bangladesh', 25, 'author', '2023-02-13 13:01:39', NULL, NULL, 'false'), (9, 'Masud ', 'Alam', 'masud.eden@gmail.com', '01722817591', 'House#812, Road#4, Baitul Aman Housing,Adabor', 28, 'admin', '2023-03-01 06:38:31', NULL, NULL, 'false'), (10, 'Shourav', ' Akter', 'shourav5@gmail.com', '01788229988', 'Pabna,Bangladesh', 18, 'subscriber', '2023-03-01 06:38:31', NULL, NULL, 'false'), (11, 'Rakibul Haque', 'Rakibul Haque', 'rakibul@gmail.com', '01899227788', 'Khulna,Bangladesh', 22, 'subscriber', '2023-03-01 06:38:31', NULL, NULL, 'false'), (12, 'Abidur Rahman', 'Abidur Rahman', 'abidur@mail.com', '01922676556', 'Dhaka,Bangladesh', 23, 'moderator', '2023-03-01 06:38:31', NULL, NULL, 'true'); -- -- Indexes for dumped tables -- -- -- Indexes for table `users` -- ALTER TABLE `users` ADD PRIMARY KEY (`id`), ADD UNIQUE KEY `users_email_unique` (`email`); -- -- AUTO_INCREMENT for dumped tables -- -- -- AUTO_INCREMENT for table `users` -- ALTER TABLE `users` MODIFY `id` bigint(20) UNSIGNED NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=1;
whereBetween
একটি কলামের value দুটি value এর মধ্যে রয়েছে এমন row গুলোকে বের করার জন্য আমরা whereBetween method টি ব্যবহার করতে পারি।
use Illuminate\Support\Facades\DB; Route::get('/query', function () { $users = DB::table('users') ->whereBetween('age', [18, 25]) ->get(); echo "<pre>"; print_r($users); echo "</pre>"; });
উপরের উদাহরণটি নিম্নলিখিত SQL তৈরি করবে:
select * from `users` where `age` between 18 and 25
orwhereBetween
একটি কলামের value দুটি value এর মধ্যে রয়েছে এমন row গুলোকে বের করার জন্য AND Condition এর পরিবর্তে যদি আপনি OR Condition যুক্ত করতে চান , সেক্ষেত্রে আপনাকে orwhereBetween method টি ব্যবহার করতে হবে।
use Illuminate\Support\Facades\DB; Route::get('/query', function () { $users = DB::table('users') ->orwhereBetween('age', [18, 20]) ->orwhereBetween('age', [20, 25]) ->get(); echo "<pre>"; print_r($users); echo "</pre>"; });
উপরের উদাহরণটি নিম্নলিখিত SQL তৈরি করবে:
select * from `users` where `age` between 18 and 20 or `age` between 20 and 25
whereNotBetween
একটি কলামের value দুটি value এর মধ্যে বিদ্যমান নয় এমন row গুলোকে বের করার জন্য আমরা whereNotBetween method টি ব্যবহার করতে পারি।
use Illuminate\Support\Facades\DB; Route::get('/query', function () { $users = DB::table('users') ->whereNotBetween('age', [20, 25]) ->get(); echo "<pre>"; print_r($users); echo "</pre>"; });
উপরের উদাহরণটি নিম্নলিখিত SQL তৈরি করবে:
select * from `users` where `age` not between 20 and 25
orwhereNotBetween
whereNotBetween method টি ব্যবহার একাধিক ব্যবহার করলে এটি সাধারণত AND Condition যুক্ত করে। এক্ষেত্রে আপনি যদি OR Condition যুক্ত করতে চান , তাহলে আপনাকে orwhereNotBetween method টি ব্যবহার করতে হবে।
use Illuminate\Support\Facades\DB; Route::get('/query', function () { $users = DB::table('users') ->whereNotBetween('age', [20, 25]) ->orwhereNotBetween('id', [3, 6]) ->get(); echo "<pre>"; print_r($users); echo "</pre>"; });
উপরের উদাহরণটি নিম্নলিখিত SQL তৈরি করবে:
select * from `users` where `age` not between 20 and 25 or `id` not between 3 and 6
whereBetweenColumns / whereNotBetweenColumns / orWhereBetweenColumns / orWhereNotBetweenColumns
Laravel এর একটি নির্দিষ্ট কলাম এর value কে অপর দুটি কলামের value এর উপর ভিত্তি করে retrive করা বা না করার জন্য whereBetweenColumns / whereNotBetweenColumns / orWhereBetweenColumns / orWhereNotBetweenColumns Method গুলো ব্যবহৃত হয়।
আর এই ব্যাপারটি বুঝার জন্য প্রথমে নিম্নোক্ত টেবিল স্ট্রাকচার এবং ডাটা গুলো এক্সেকিউট করে নিন:
-- -- Table structure for table `patients` -- CREATE TABLE `patients` ( `patient_id` int(11) NOT NULL, `name` varchar(40) NOT NULL, `weight` float NOT NULL, `minimum_allowed_weight` float NOT NULL, `maximum_allowed_weight` float NOT NULL ) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_general_ci; -- -- Dumping data for table `patients` -- INSERT INTO `patients` (`patient_id`, `name`, `weight`, `minimum_allowed_weight`, `maximum_allowed_weight`) VALUES (1, 'Imran Khan', 65.5, 50, 75), (2, 'Mostafizur Rahman', 68, 50, 65), (3, 'Toriqul Islam', 55, 58, 75), (4, 'Sofiqul Islam', 55, 50, 66); -- -- Indexes for dumped tables -- -- -- Indexes for table `patients` -- ALTER TABLE `patients` ADD PRIMARY KEY (`patient_id`);
Laravel এর একটি নির্দিষ্ট কলাম এর value কে অপর দুটি কলামের value এর Range এর মধ্যের row গুলো নিয়ে আসার জন্য আমরা whereBetweenColumns Method টি ব্যবহার করব:
use Illuminate\Support\Facades\DB; Route::get('/query', function () { $patients = DB::table('patients') ->whereBetweenColumns('weight', ['minimum_allowed_weight', 'maximum_allowed_weight']) ->get(); echo "<pre>"; print_r($patients); echo "</pre>"; });
উপরের উদাহরণটি নিম্নলিখিত SQL তৈরি করবে:
select * from `patients` where `weight` between `minimum_allowed_weight` and `maximum_allowed_weight`
উপরের উদাহরণের ঠিক বিপরীত কাজটি করার জন্য whereNotBetweenColumns মেথড টি ব্যবহার করব:
use Illuminate\Support\Facades\DB; Route::get('/query', function () { $patients = DB::table('patients') ->whereNotBetweenColumns('weight', ['minimum_allowed_weight', 'maximum_allowed_weight']) ->get(); echo "<pre>"; print_r($patients); echo "</pre>"; });
উপরের উদাহরণটি নিম্নলিখিত SQL তৈরি করবে:
select * from `patients` where `weight` not between `minimum_allowed_weight` and `maximum_allowed_weight`
whereBetweenColumns এবং whereNotBetweenColumns দুটি আপনি চাইলে একাধিক বার চেইনিং এর মাধ্যমে ব্যবহার করতে পারেন এক্ষেত্রে এই দুটি মেথড AND Logic ব্যবহার করবে। এক্ষেত্রে আপনি যদি OR Logic ব্যবহার করতে চান , তাহলে আপনাকে orWhereBetweenColumns / orWhereNotBetweenColumns Method ব্যবহার করতে হবে।
whereIn / whereNotIn / orWhereIn / orWhereNotIn / whereIntegerInRaw / whereIntegerNotInRaw
whereIn method এর মাধ্যমে একটি নির্দিষ্ট কলামের value একটি প্রদত্ত array মধ্যে রয়েছে কিনা সেটি চেক করে এবং যেসব value পাওয়া যায় সে গুলো return করে:
use Illuminate\Support\Facades\DB; Route::get('/query', function () { $employees = DB::table('employees') ->whereIn('id', [1, 5, 8]) ->get(); echo "<pre>"; print_r($employees); echo "</pre>"; });
উপরের উদাহরণটি নিম্নলিখিত SQL তৈরি করবে:
select * from `employees` where `id` in (1, 5, 8)
আবার এর ঠিক উল্টোটা , অর্থাৎ একটি নির্দিষ্ট কলামের value একটি প্রদত্ত array মধ্যে অবস্থিত value গুলো ছাড়া বাকি সব return করার জন্য আপনি whereNotIn Method টি ব্যবহার করতে পারেন :
use Illuminate\Support\Facades\DB; Route::get('/query', function () { $employees = DB::table('employees') ->whereNotIn('id', [1, 5, 8]) ->get(); echo "<pre>"; print_r($employees); echo "</pre>"; });
উপরের উদাহরণটি নিম্নলিখিত SQL তৈরি করবে:
select * from `employees` where `id` not in (1, 5, 8)
whereIn / whereNotIn Method দুটি আপনি চাইলে একাধিক বার চেইনিং এর মাধ্যমে ব্যবহার করতে পারেন এক্ষেত্রে এই দুটি মেথড AND Logic ব্যবহার করবে। এক্ষেত্রে আপনি যদি OR Logic ব্যবহার করতে চান , তাহলে আপনাকে orWhereIn / orWhereNotIn Method দুটি ব্যবহার করতে হবে।
এছাড়াও আপনি চাইলে whereIn Method এর Second Argument হিসেবে একটি query object ব্যবহার করতে পারেন :
$activeUsers = DB::table('users')->select('id')->where('is_active', 1); $users = DB::table('comments') ->whereIn('user_id', $activeUsers) ->get();
উপরের উদাহরণটি নিম্নলিখিত SQL তৈরি করবে:
select * from comments where user_id in ( select id from users where is_active = 1 )
whereIntegerInRaw / whereIntegerNotInRaw
আপনি যদি আপনার query তে integer bindings এ একটি বড় অ্যারে যোগ করেন, যেখানে আপনার মেমরির ব্যবহার ব্যাপকভাবে reduce করতে whereIntegerInRaw বা whereIntegerNotInRaw methods ব্যবহার করা যেতে পারে।
Laravel-এ whereIntegerInRaw method টি query তে একটি raw where clause যোগ করতে ব্যবহৃত হয় যা integer values গুলোর array তে একটি column value উপস্থিত আছে কি অথবা নাই তা পরীক্ষা করে।
ধরুন আমাদের কাছে age column সহ একটি users table আছে, এবং আমরা সেই সমস্ত users দের retrieve করতে চাই যাদের বয়স হয় 18, 21 বা 25, আমরা এই value গুলো পাওয়ার জন্য whereIntegerInRaw Method টি ব্যবহার করতে পারি:
use Illuminate\Support\Facades\DB; Route::get('/query', function () { $ages = [18, 21, 25]; $users = DB::table('users') ->whereIntegerInRaw('age', $ages) ->get(); echo "<pre>"; print_r($users); echo "</pre>"; });
উপরের উদাহরণটি নিম্নলিখিত SQL তৈরি করবে:
select * from `users` where `age` in (18, 21, 25)
আবার এর ঠিক উল্টোটা , অর্থাৎ একটি নির্দিষ্ট কলামের value একটি প্রদত্ত array মধ্যে অবস্থিত value গুলো ছাড়া বাকি সব return করার জন্য আপনি whereIntegerNotInRaw Method টি ব্যবহার করতে পারেন :
use Illuminate\Support\Facades\DB; Route::get('/query', function () { $ages = [18, 21, 25]; $users = DB::table('users') ->whereIntegerNotInRaw ('age', $ages) ->get(); echo "<pre>"; print_r($users); echo "</pre>"; });
উপরের উদাহরণটি নিম্নলিখিত SQL তৈরি করবে:
select * from `users` where `age` not in (18, 21, 25)
whereNull / whereNotNull / orWhereNull / orWhereNotNull
whereNull Method এর মাধ্যমে একটি কলাম Null কি না সেটা যাচাই করতে পারবেন:
use Illuminate\Support\Facades\DB; Route::get('/query', function () { $users = DB::table('users') ->whereNull('updated_at') ->get(); echo "<pre>"; print_r($users); echo "</pre>"; });
উপরের উদাহরণটি নিম্নলিখিত SQL তৈরি করবে:
select * from `users` where `updated_at` is null
একইভাবে whereNotNull Method এর মাধ্যমে একটি কলাম Null নয় সেটা যাচাই করতে পারবেন:
use Illuminate\Support\Facades\DB; Route::get('/query', function () { $users = DB::table('users') ->whereNotNull('updated_at') ->get(); echo "<pre>"; print_r($users); echo "</pre>"; });
উপরের উদাহরণটি নিম্নলিখিত SQL তৈরি করবে:
select * from `users` where `updated_at` is not null
whereNull / whereNotNull Method দুটি আপনি চাইলে একাধিক বার চেইনিং এর মাধ্যমে ব্যবহার করতে পারেন এক্ষেত্রে এই দুটি মেথড AND Logic ব্যবহার করবে। এক্ষেত্রে আপনি যদি OR Logic ব্যবহার করতে চান , তাহলে আপনাকে orWhereNull / orWhereNotNull Method দুটি ব্যবহার করতে হবে:
whereNull / whereNotNull Method Chaining Example
use Illuminate\Support\Facades\DB; Route::get('/query', function () { $users = DB::table('users') ->whereNull('updated_at') ->whereNotNull('created_at') ->get(); echo "<pre>"; print_r($users); echo "</pre>"; });
উপরের উদাহরণটি নিম্নলিখিত SQL তৈরি করবে:
select * from `users` where `updated_at` is null and `created_at` is not null
এবার চলুন orWhereNull Method এর উদাহরণ দেখা যাক:
use Illuminate\Support\Facades\DB; Route::get('/query', function () { $users = DB::table('users') ->where('name', 'John') ->orWhereNull('email') ->get(); echo "<pre>"; print_r($users); echo "</pre>"; });
উপরের উদাহরণটি নিম্নলিখিত SQL তৈরি করবে:
elect * from `users` where `name` = 'John' or `email` is null
whereDate / whereMonth / whereDay / whereYear / whereTime
whereDate Method
একটি নির্দিষ্ট তারিখের উপর ভিত্তি করে একটি ডাটাবেস টেবিল থেকে রেকর্ড ফিল্টার করতে whereDate method টি ব্যবহার করা হয়। এটির দুটি arguments লাগে: ফিল্টার করার জন্য কলামের নাম এবং ফিল্টার করার তারিখ।
ধরুন আপনার কাছে columns id, name এবং create_at সহ একটি users টেবিল রয়েছে। আপনি একটি নির্দিষ্ট তারিখে তৈরি করা সমস্ত ইউজারদেরকে retrieve করতে চান, ধরুন 27 শে ফেব্রুয়ারি, 2023। আপনি লারাভেলের whereDate method টি নিম্নরূপ ব্যবহার করতে পারেন:
use Illuminate\Support\Facades\DB; Route::get('/query', function () { $users = DB::table('users') ->whereDate('created_at', '2023-02-27') ->get(); echo "<pre>"; print_r($users); echo "</pre>"; });
উপরের উদাহরণটি নিম্নলিখিত SQL তৈরি করবে:
select * from `users` where date(`created_at`) = '2023-02-27'
এটি সেই সমস্ত ইউজারদের retrieve করবে যাদের created_at date নির্দিষ্ট date এর সাথে মেলে। উল্লেখ্য যে whereDate method টি শুধুমাত্র created_at কলামের date এর অংশের তুলনা করে এবং time অংশটিকে ইগনোর করে।
আপনি whereDate method টির সাথে অন্যান্য date তুলনা করতে বিভিন্ন comparison operator গুলো ব্যবহার করতে পারেন। উদাহরণস্বরূপ, 27 ফেব্রুয়ারী, 2023 এর আগে তৈরি করা সমস্ত ইউজারদের retrieve করতে, আপনি < অপারেটরের সাথে whereDate method টি নিম্নরূপ ব্যবহার করতে পারেন:
use Illuminate\Support\Facades\DB; Route::get('/query', function () { $users = DB::table('users') ->whereDate('created_at', '<', '2023-02-27') ->get(); echo "<pre>"; print_r($users); echo "</pre>"; });
উপরের উদাহরণটি নিম্নলিখিত SQL তৈরি করবে:
select * from `users` where date(`created_at`) < '2023-02-27'
এটি সেই সমস্ত ইউজারদের retrieve করবে যাদের created_at তারিখ ফেব্রুয়ারী 27, 2023 এর আগের।
whereMonth Method
একটি নির্দিষ্ট মাসের উপর ভিত্তি করে একটি ডাটাবেস টেবিল থেকে রেকর্ড ফিল্টার করতে whereMonth method টি ব্যবহার করা হয়। এটির দুটি arguments লাগে: ফিল্টার করার জন্য কলামের নাম এবং ফিল্টার করার মাসের ।
ধরুন আপনার কাছে columns id, name এবং created_at সহ একটি users টেবিল রয়েছে। আপনি একটি নির্দিষ্ট মাসের তৈরি করা সমস্ত ইউজারদেরকে retrieve করতে চান, ধরুন ফেব্রুয়ারি মাসের । আপনি লারাভেলের whereMonth method টি নিম্নরূপ ব্যবহার করতে পারেন:
use Illuminate\Support\Facades\DB; Route::get('/query', function () { $users = DB::table('users') ->whereMonth('created_at', '02') ->get(); echo "<pre>"; print_r($users); echo "</pre>"; });
উপরের উদাহরণটি নিম্নলিখিত SQL তৈরি করবে:
select * from `users` where month(`created_at`) = '02'
এটি সেই সমস্ত ইউজারদের retrieve করবে যাদের created_at মাস নির্দিষ্ট মাসের এর সাথে মেলে। উল্লেখ্য যে whereMonth method টি শুধুমাত্র created_at কলামের Month এর অংশের তুলনা করে এবং দিন , বছর এবং সময়ের অংশটিকে ইগনোর করে।
আপনি whereMonth method টির সাথে অন্যান্য month তুলনা করতে বিভিন্ন comparison operator গুলো ব্যবহার করতে পারেন। উদাহরণস্বরূপ, ফেব্রুয়ারী এর আগে তৈরি করা সমস্ত ইউজারদের retrieve করতে, আপনি < অপারেটরের সাথে whereMonth method টি নিম্নরূপ ব্যবহার করতে পারেন:
use Illuminate\Support\Facades\DB; Route::get('/query', function () { $users = DB::table('users') ->whereMonth('created_at', '<', '02') ->get(); echo "<pre>"; print_r($users); echo "</pre>"; });
উপরের উদাহরণটি নিম্নলিখিত SQL তৈরি করবে:
select * from `users` where month(`created_at`) < '02'
এটি সেই সমস্ত ইউজারদের retrieve করবে যাদের created_at মাস ফেব্রুয়ারী এর আগের।
whereYear Method
একটি নির্দিষ্ট বছরের উপর ভিত্তি করে একটি ডাটাবেস টেবিল থেকে রেকর্ড ফিল্টার করতে whereYear method টি ব্যবহার করা হয়। এটির দুটি arguments লাগে: ফিল্টার করার জন্য কলামের নাম এবং ফিল্টার করার বছরের ।
ধরুন আপনার কাছে columns id, name এবং created_at সহ একটি users টেবিল রয়েছে। আপনি একটি নির্দিষ্ট বছরের তৈরি করা সমস্ত ইউজারদেরকে retrieve করতে চান, ধরুন ২০২৩ সালের । আপনি লারাভেলের whereYear method টি নিম্নরূপ ব্যবহার করতে পারেন:
use Illuminate\Support\Facades\DB; Route::get('/query', function () { $users = DB::table('users') ->whereYear ('created_at', '2023') ->get(); echo "<pre>"; print_r($users); echo "</pre>"; });
উপরের উদাহরণটি নিম্নলিখিত SQL তৈরি করবে:
select * from `users` where year(`created_at`) = '2023'
এটি সেই সমস্ত ইউজারদের retrieve করবে যাদের created_at বছর নির্দিষ্ট বছরের এর সাথে মেলে। উল্লেখ্য যে whereYear method টি শুধুমাত্র created_at কলামের Year এর অংশের তুলনা করে এবং দিন , মাস এবং সময়ের অংশটিকে ইগনোর করে।
আপনি whereYear method টির সাথে অন্যান্য Year তুলনা করতে বিভিন্ন comparison operator গুলো ব্যবহার করতে পারেন। উদাহরণস্বরূপ, ২০২৩ সালের আগে তৈরি করা সমস্ত ইউজারদের retrieve করতে, আপনি < অপারেটরের সাথে whereYear method টি নিম্নরূপ ব্যবহার করতে পারেন:
use Illuminate\Support\Facades\DB; Route::get('/query', function () { $users = DB::table('users') ->whereYear('created_at', '<', '2023') ->get(); echo "<pre>"; print_r($users); echo "</pre>"; });
উপরের উদাহরণটি নিম্নলিখিত SQL তৈরি করবে:
select * from `users` where year(`created_at`) < '2023'
এটি সেই সমস্ত ইউজারদের retrieve করবে যাদের created_at বছর ২০২৩ সালের আগের।
whereDay Method
একটি মাসের একটি নির্দিষ্ট দিনের উপর ভিত্তি করে একটি ডাটাবেস টেবিল থেকে রেকর্ড ফিল্টার করতে whereDay method টি ব্যবহার করা হয়। এটির দুটি arguments লাগে: ফিল্টার করার জন্য কলামের নাম এবং ফিল্টার করার মাসের একটি নির্দিষ্ট দিনের ।
ধরুন আপনার কাছে columns id, name এবং created_at সহ একটি users টেবিল রয়েছে। আপনি একটি মাসের একটি নির্দিষ্ট দিনের তৈরি করা সমস্ত ইউজারদেরকে retrieve করতে চান, ধরুন ডিসেম্বর মাসের ৩১ তারিখের। আপনি লারাভেলের whereDay method টি নিম্নরূপ ব্যবহার করতে পারেন:
use Illuminate\Support\Facades\DB; Route::get('/query', function () { $users = DB::table('users') ->whereDay ('created_at', '31') ->get(); echo "<pre>"; print_r($users); echo "</pre>"; });
উপরের উদাহরণটি নিম্নলিখিত SQL তৈরি করবে:
select * from `users` where day(`created_at`) = '31'
এটি সেই সমস্ত ইউজারদের retrieve করবে যাদের created_at বছর নির্দিষ্ট দিনের সাথে মেলে। উল্লেখ্য যে whereDay method টি শুধুমাত্র created_at কলামের Day এর অংশের তুলনা করে এবং বছর, মাস এবং সময়ের অংশটিকে ইগনোর করে।
আপনি whereDay method টির সাথে অন্যান্য Day তুলনা করতে বিভিন্ন comparison operator গুলো ব্যবহার করতে পারেন। উদাহরণস্বরূপ, ৩১ তারিখের আগে তৈরি করা সমস্ত ইউজারদের retrieve করতে, আপনি < অপারেটরের সাথে whereDay method টি নিম্নরূপ ব্যবহার করতে পারেন:
use Illuminate\Support\Facades\DB; Route::get('/query', function () { $users = DB::table('users') ->whereDay('created_at', '<', '31') ->get(); echo "<pre>"; print_r($users); echo "</pre>"; });
উপরের উদাহরণটি নিম্নলিখিত SQL তৈরি করবে:
select * from `users` where day(`created_at`) < '31'
এটি সেই সমস্ত ইউজারদের retrieve করবে যাদের created_at দিন ৩১ তারিখের আগের।
whereTime Method
একটি নির্দিষ্ট সময়ের উপর ভিত্তি করে একটি ডাটাবেস টেবিল থেকে রেকর্ড ফিল্টার করতে whereTime method টি ব্যবহার করা হয়। এটির দুটি arguments লাগে: ফিল্টার করার জন্য কলামের নাম এবং ফিল্টার করার একটি নির্দিষ্ট সময়ের ।
ধরুন আপনার কাছে columns id, name এবং created_at সহ একটি users টেবিল রয়েছে। আপনি একটি সময়ে তৈরি করা সমস্ত ইউজারদেরকে retrieve করতে চান, ধরুন 07:38:05
সময়ের । আপনি লারাভেলের whereTime method টি নিম্নরূপ ব্যবহার করতে পারেন:
use Illuminate\Support\Facades\DB; Route::get('/query', function () { $users = DB::table('users') ->whereTime('created_at', '07:38:05') ->get(); echo "<pre>"; print_r($users); echo "</pre>"; });
উপরের উদাহরণটি নিম্নলিখিত SQL তৈরি করবে:
select * from `users` where time(`created_at`) = '07:38:05'
এটি সেই সমস্ত ইউজারদের retrieve করবে যাদের created_at একটি নির্দিষ্ট সময়ের সাথে মেলে। উল্লেখ্য যে whereTime method টি শুধুমাত্র created_at কলামের Time এর অংশের তুলনা করে এবং বছর, মাস এবং দিন অংশটিকে ইগনোর করে।
আপনি whereTime method টির সাথে অন্যান্য Day তুলনা করতে বিভিন্ন comparison operator গুলো ব্যবহার করতে পারেন। উদাহরণস্বরূপ, 07:38:05 সময়ের আগে তৈরি করা সমস্ত ইউজারদের retrieve করতে, আপনি < অপারেটরের সাথে whereDay method টি নিম্নরূপ ব্যবহার করতে পারেন:
use Illuminate\Support\Facades\DB; Route::get('/query', function () { $users = DB::table('users') ->whereTime('created_at', '<', '07:38:05') ->get(); echo "<pre>"; print_r($users); echo "</pre>"; });
উপরের উদাহরণটি নিম্নলিখিত SQL তৈরি করবে:
select * from `users` where time(`created_at`) < '07:38:05'
এটি সেই সমস্ত ইউজারদের retrieve করবে যাদের created_at 07:38:05 আগের।
whereColumn / orWhereColumn
Laravel-এ whereColumn method টি দুটি কলামের মানের মধ্যে তুলনা করতে ব্যবহৃত হয়।
whereColumn method এ দুটি আর্গুমেন্ট লাগে: প্রথম আর্গুমেন্ট হল সেই কলাম যা আপনি তুলনা করতে চান এবং দ্বিতীয় আর্গুমেন্ট হল সেই কলাম যার সাথে আপনি এটি তুলনা করতে চান৷ উদাহরণস্বরূপ, কলাম created_at এবং updated_at সহ একটি users table কল্পনা করুন। created_at কলামটি updated_at কলামের সমান এমন সমস্ত users কে খুঁজে পেতে, আপনি নিম্নলিখিত কোডটি ব্যবহার করতে পারেন:
use Illuminate\Support\Facades\DB; Route::get('/query', function () { $users = DB::table('users') ->whereColumn('created_at', 'updated_at') ->get(); echo "<pre>"; print_r($users); echo "</pre>"; });
উপরের উদাহরণটি নিম্নলিখিত SQL তৈরি করবে:
select * from `users` where `created_at` = `updated_at`
whereColumn method টি অন্যান্য comparison অপারেটরগুলির সাথেও ব্যবহার করা যেতে পারে, যেমন <, >, <=, >=, এবং <>। উদাহরণ স্বরূপ:
use Illuminate\Support\Facades\DB; Route::get('/query', function () { $users = DB::table('users') ->whereColumn('created_at','<','updated_at') ->get(); echo "<pre>"; print_r($users); echo "</pre>"; });
উপরের উদাহরণটি নিম্নলিখিত SQL তৈরি করবে:
select * from `users` where `created_at` < `updated_at`
এছাড়াও আপনি whereColumn method ব্যবহার করে comparisons করার জন্য একটি array পাস করতে পারেন। তখন conditions গুলি and Operator ব্যবহার করবে:
use Illuminate\Support\Facades\DB; Route::get('/query', function () { $users = DB::table('users') ->whereColumn([ ['first_name', '=', 'last_name'], ['updated_at', '>', 'created_at'], ])->get(); echo "<pre>"; print_r($users); echo "</pre>"; });
উপরের উদাহরণটি নিম্নলিখিত SQL তৈরি করবে:
select * from `users` where (`first_name` = `last_name` and `updated_at` > `created_at`)
Logical Grouping
Laravel Query Builder-এ, logical grouping বলতে একাধিক গ্রুপ করার ক্ষমতা বোঝায় যেখানে logical operator গুলো যেমন and এবং or ব্যবহার করে multiple where clauses একসাথে করা হয়। এটি আপনাকে আপনার query গুলোর জন্য আরও complex conditions তৈরি করতে দেয়।
উদাহরণ স্বরূপ, ধরা যাক আপনি সমস্ত ইউজারদের retrieve করতে চান যারা হয় admins বা moderators এবং যারা active। আপনি Laravel Query Builder এ logical grouping ব্যবহার করে এটি পেতে পারেন:
use Illuminate\Support\Facades\DB; Route::get('/query', function () { $users = DB::table('users') ->where(function ($query) { $query->where('role', '=', 'admin') ->orWhere('role', '=', 'moderator'); }) ->where('active', '=', 'true') ->get(); echo "<pre>"; print_r($users); echo "</pre>"; });
Advanced Where Clauses
Where Exists Clauses
Laravel Query Builder-এ, “whereExists” method টি একটি query তে একটি subquery constraint যোগ করতে ব্যবহৃত হয়। এই method টি আপনাকে একটি subquery specify করতে দেয় যা অন্য টেবিলে একটি রেকর্ড বিদ্যমান কিনা তা পরীক্ষা করতে ব্যবহার করা হয় এবং তারপর সেই চেকের ফলাফলটি ব্যবহার করে মূল query এর ফলাফলগুলি ফিল্টার করতে দেয় ।
Advance Where Clauses এর উদাহরণ গুলোতে আমরা আমাদের পূর্বের তৈরি করা users টেবিল এর পাশাপাশি নিম্নোক্ত posts টেবিল স্ট্রাকচার এবং তার ডেটা গুলো ব্যবহার করব :
-- -- Table structure for table `posts` -- CREATE TABLE `posts` ( `id` int(11) NOT NULL, `user_id` int(11) NOT NULL, `title` varchar(255) NOT NULL, `content` text NOT NULL ) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_general_ci; -- -- Dumping data for table `posts` -- INSERT INTO `posts` (`id`, `user_id`, `title`, `content`) VALUES (1, 2, 'What is HTML?', 'HTML (HyperText Markup Language) is a language used for creating webpages which is the fundamental building block of the Web.\r\n\r\n'), (2, 1, 'HTML Basics', 'In this tutorial, you will learn about HTML Basics and their implementation with the help of examples.'), (3, 3, 'HTML Hierarchy', 'HTML elements are hierarchical, which means that they can be nested inside each other to create a tree-like structure of the content on the web page.'), (4, 2, 'What are HTML elements?', 'HTML elements consist of several parts, including the opening and closing tags, the content, and the attributes. Here is an explanation of each of these parts:'), (5, 4, 'What are HTML Attributes?', 'HTML elements can have attributes, which provide additional information about the element.'); -- -- Indexes for dumped tables -- -- -- Indexes for table `posts` -- ALTER TABLE `posts` ADD PRIMARY KEY (`id`), ADD KEY `user_id` (`user_id`); ALTER TABLE `posts` ADD FULLTEXT KEY `title` (`title`); -- -- AUTO_INCREMENT for dumped tables -- -- -- AUTO_INCREMENT for table `posts` -- ALTER TABLE `posts` MODIFY `id` int(11) NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=1;
ধরা যাক আপনার ডাটাবেসে দুটি টেবিল আছে: users এবং posts। প্রতিটি ইউজারের একাধিক পোস্ট থাকতে পারে। আপনি এমন সমস্ত ইউজারদের retrieve করতে চান যাদের অন্তত একটি পোস্ট আছে।
আপনি এই কাজটি করার জন্য whereExists Method ব্যবহার করতে পারেন:
use Illuminate\Support\Facades\DB; Route::get('/query', function () { $users = DB::table('users') ->whereExists(function ($query) { $query->select(DB::raw(1)) ->from('posts') ->whereRaw('posts.user_id = users.id'); }) ->get(); echo "<pre>"; print_r($users); echo "</pre>"; });
আবার, আপনি closure ব্যবহার করার পরিবর্তে whereExists Method এ একটি query object দিতে পারেন:
use Illuminate\Support\Facades\DB; Route::get('/query', function () { $posts = DB::table('posts') ->select(DB::raw(1)) ->whereColumn('posts.user_id', 'users.id'); $users = DB::table('users') ->whereExists($posts) ->get(); echo "<pre>"; print_r($users); echo "</pre>"; });
উপরের উদাহরণ দুটি নিম্নলিখিত SQL তৈরি করবে:
select * from `users` where exists (select 1 from `posts` where posts.user_id = users.id)
Full Text Where Clauses
Laravel query builder এ, আপনি whereFullText method টি ব্যবহার করে একটি ডাটাবেস টেবিলের একটি নির্দিষ্ট কলামে একটি full-text search করতে পারেন। এখানে একটি query builder এ, whereFullText ব্যবহার করার একটি উদাহরণ দেওয়া হলো:
use Illuminate\Support\Facades\DB; Route::get('/query', function () { $searchQuery = "HTML"; $results = DB::table('posts') ->whereFullText('title', $searchQuery) ->get(); echo "<pre>"; print_r($results); echo "</pre>"; });
উপরের উদাহরণে, আমরা এমন সমস্ত পোস্টের জন্য সার্চ করছি যেগুলির title column এ “HTML ” শব্দটি রয়েছে৷ whereFullText method এ দুটি আর্গুমেন্ট লাগে – সার্চ এর জন্য কলামের নাম এবং search query string।
উপরের উদাহরণটি নিম্নলিখিত SQL তৈরি করবে:
select * from `posts` where match (`title`) against ('HTML' in natural language mode)