PHP Arrays
PHP Arrays পর্ব-৪: PHP Array Data Manipulation Functions
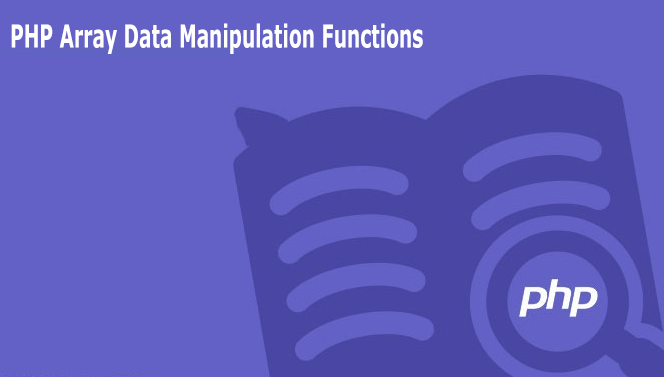
PHP array তে Data কে বিভিন্ন ভাবে manipulation করতে হয়, আর এই Data Manipulation এর মধ্যে আমাদেরকে array তে নতুন Data ঢুকানো, পুরাতন Data আপডেট অথবা রিমুভ ও করতে হয় , একইভাবে আমাদের কে একটি array এর সাথে আরেকটি array কে Merge এবং Combine ও করতে হয়। আরো করতে হয় বিভিন্ন রকমের filtering। আজকের পর্বে আমরা PHP array এর Data এর বিভিন্ন Manipulation গুলো দেখবো। চলুন এক এক করে সবগুলো দেখা যাক :
PHP array_filter() Function
PHP তে array এর মধ্যের Data গুলোকে প্রোগ্রামার এর দেওয়া callback function এর উপর ভিত্তি করে ফিল্টারিং করার জন্য array_filter function টি ব্যবহৃত হয়। চলুন আমরা একটা নির্দিষ্ট array এর value গুলো থেকে Odd এবং even নম্বর গুলোকে array_filter function দিয়ে filtering করে নেই।
<?php function odd($var) { // returns whether the input integer is odd return $var % 2==1; } function even($var) { // returns whether the input integer is even return $var % 2==0; } $array = range(1,20); echo "<pre>"; echo "Odd :"; print_r(array_filter($array, "odd")); echo "Even:"; print_r(array_filter($array, "even")); echo "</pre>"; ?>
Output:
Odd :Array ( [0] => 1 [2] => 3 [4] => 5 [6] => 7 [8] => 9 [10] => 11 [12] => 13 [14] => 15 [16] => 17 [18] => 19 ) Even:Array ( [1] => 2 [3] => 4 [5] => 6 [7] => 8 [9] => 10 [11] => 12 [13] => 14 [15] => 16 [17] => 18 [19] => 20 )
লক্ষ্য করুন , এখানে আমরা 1-20 দিয়ে তৈরী array টি কে দুটি callback function odd এবং even ফাঙ্কশন দিয়ে যথাক্রমে Odd Number গুলো এবং Even Number গুলো filtering করি।
সাধারণত, বাই ডিফল্ট array_filter function টি শুধু array এর value কে নিয়ে কাজ করে তবে আপনি চাইলে শুধু key অথবা key এবং value দুটিকে নিয়েই কাজ করতে পারেন। আর এর জন্য আপনাকে ARRAY_FILTER_USE_KEY এবং ARRAY_FILTER_USE_BOTH দুটি built-in constant ব্যবহার করতে হবে। চলুন একটি উদাহরণ দিয়ে বুঝে নেয়া যাক :
<?php function filterByKey($k){ return ($k=="b" || $k=="e"); } function filterByBoth($v,$k){ return ($k=="b" || $v=="Canada"); } $array=["a"=>"America","b"=>"Bangladesh","c"=>"Canada","d"=>"Denmark","e"=>"England"]; echo "<pre>"; echo "Filtering By Key :"; print_r(array_filter($array, "filterByKey",ARRAY_FILTER_USE_KEY)); echo "Filtering By Both :"; print_r(array_filter($array, "filterByBoth",ARRAY_FILTER_USE_BOTH)); ?>
Output
Filtering By Key :Array ( [b] => Bangladesh [e] => England ) Filtering By Both :Array ( [b] => Bangladesh [c][/c]
এছাড়া আপনি array_filter() ফাঙ্কশন এর মধ্যে regular expression ব্যবহার করেও সব ধরণের ডেটা ফিল্টারিং করতে পারেন। নিচের উদাহরণে আপন দেখতে পাবেন কিভাবে আমরা সেইসব Country List পাবো , যাদের শুরুতে “a” দিয়ে শুরু:
<?php $countries=["a"=>"America","b"=>"Bangladesh","c"=>"Canada","d"=>"Denmark","e"=>"England","australia"]; $filtered_country=array_filter($countries, function($name){ return preg_match('/^a/i',$name); }); print_r($filtered_country); ?>
Example#3
<?php // Sample array of users $users = [ ['id' => 1, 'name' => 'John', 'status' => 'active'], ['id' => 2, 'name' => 'Jane', 'status' => 'inactive'], ['id' => 3, 'name' => 'Doe', 'status' => 'active'], ['id' => 4, 'name' => 'Alice', 'status' => 'inactive'], ]; // Callback function to filter inactive users function filterInactive($user) { return $user['status'] === 'active'; } // Use array_filter to filter the users $activeUsers = array_filter($users, 'filterInactive'); // Display the result print_r($activeUsers); ?>
Example#4
<?php // Sample array of products $products = [ ['id' => 1, 'name' => 'Laptop', 'price' => 1200], ['id' => 2, 'name' => 'Smartphone', 'price' => 800], ['id' => 3, 'name' => 'Headphones', 'price' => 150], ['id' => 4, 'name' => 'Tablet', 'price' => 500], ]; // Price threshold for filtering $priceThreshold = 1000; // Callback function to filter products above the price threshold function filterExpensiveProducts($product) { return $product['price'] <= 1000; } // Use array_filter to filter the products $affordableProducts = array_filter($products, 'filterExpensiveProducts'); // Display the result print_r($affordableProducts); ?>
Example#5
<?php // Sample array of file names $fileNames = [ 'document.txt', 'image.jpg', 'data.csv', 'presentation.pptx', 'script.js', ]; // File extension to filter $targetExtension = 'txt'; // Callback function to filter files with a specific extension function filterFilesByExtension($fileName) { $fileParts = pathinfo($fileName); return isset($fileParts['extension']) && $fileParts['extension'] === 'txt'; } // Use array_filter to filter the files $textFiles = array_filter($fileNames, 'filterFilesByExtension'); // Display the result print_r($textFiles); ?>
PHP array_map() Function
PHP তে এক বা একাধিক array এর মধ্যের প্রত্যেকটি Data বা element কে প্রোগ্রামার এর দেওয়া callback function এর উপর ভিত্তি করে যেকোনো operation করার জন্য array_map function টি ব্যবহৃত হয়। চলুন একটা উদাহরণ দিয়ে বুঝে নেয়া যাক ।
<?php function cube($n) { return($n * $n * $n); } $a = array(1, 2, 3, 4, 5); $b = array_map("cube", $a); print_r($b); ?>
Result:
Array ( [0] => 1 [1] => 8 [2] => 27 [3] => 64 [4] => 125 )
একই ভাবে array_map function এ একাধিক array নিয়েও কাজ করতে পারি। নিচের উদাহরণ দেখুন
<?php function show_Spanish($n, $m) { return("The number $n is called $m in Spanish"); } $a = array(1, 2, 3, 4, 5); $b = array("uno", "dos", "tres", "cuatro", "cinco"); echo "<pre>"; echo "Array Mapping :"; $c = array_map("show_Spanish", $a, $b); print_r($c); ?>
Output
Array Mapping :Array ( [0] => The number 1 is called uno in Spanish [1] => The number 2 is called dos in Spanish [2] => The number 3 is called tres in Spanish [3] => The number 4 is called cuatro in Spanish [4] => The number 5 is called cinco in Spanish )
Example#3
<?php // Sample array of product prices $productPrices = [50.99, 29.95, 99.99, 149.99, 79.99]; // Discount percentage to apply $discountPercentage = 10; // Callback function to apply a discount to each price function applyDiscount($price) { // Calculate the discounted price $discountedPrice = $price - ($price * ($GLOBALS['discountPercentage'] / 100)); // Round the result to 2 decimal places to represent currency return round($discountedPrice, 2); } // Use array_map to apply the discount to each product price $discountedPrices = array_map('applyDiscount', $productPrices); // Display the result print_r($discountedPrices); ?>
Example#4
<?php // Sample array of names $names = ['John', 'Jane', 'Alice', 'Bob']; // Callback function to transform each name to uppercase function convertToUppercase($name) { return strtoupper($name); } // Use array_map to convert each name to uppercase $uppercaseNames = array_map('convertToUppercase', $names); // Display the result print_r($uppercaseNames); ?>
PHP array_walk() Function
Array এর প্রত্যেকটি Member এর উপর user এর বা প্রোগ্রামারের দেওয়া callback function ব্যবহার করার জন্য PHP তে array_walk() ফাঙ্কশনটি ব্যবহৃত হয়। array_walk() ফাঙ্কশন array এর মধ্যের value গুলোকে user define callback function এর প্রথম Parameter হিসেবে পাস করে , আর key গুলোকে দ্বিতীয় Parameter হিসেবে পাস করে। চলুন একটা উদাহরণ দিয়ে ব্যাপারটা আরো ভালোভাবে বুঝে নেয়া যাক :
<?php function arrayValues($value,$key) { echo $value,"<br>"; } function arrayKeys($value,$key) { echo $key,"<br>"; } function arrayKeyVal($value,$key) { echo "$key=$value","<br>"; } $array=["a"=>"America","b"=>"Bangladesh","c"=>"Canada","d"=>"Denmark","e"=>"England"]; echo "<strong>Get Array Values with array_walk Function :</strong><br>"; array_walk($array, "arrayValues"); echo "<br><br>"; echo "<strong>Get Array Keys with array_walk Function :</strong><br>"; array_walk($array, "arrayKeys"); echo "<br><br>"; echo "<strong>Get Array Key and Values with array_walk Function :</strong><br>"; array_walk($array, "arrayKeyVal"); ?>
Output
Get Array Values with array_walk Function : America Bangladesh Canada Denmark England Get Array Keys with array_walk Function : a b c d e Get Array Key and Values with array_walk Function : a=America b=Bangladesh c=Canada d=Denmark e=England
লক্ষ্য করুন, উপরে আমরা array_walk function টির জন্য তিনটা ফাঙ্কশন তৈরী করি , তারমধ্যে arrayValues ফাঙ্কশনের কাজ হচ্ছে array এর value গুলোকে string হিসেবে print করবে এবং arrayKeys ফাঙ্কশনের কাজ হচ্ছে array থেকে শুধু key গুলোকে print করবে, সর্বেশেষ arrayKeyVal ফাঙ্কশনের কাজ হচ্ছে array key এবং value উভয়ই print করবে।
কিছু array_walk ফাঙ্কশন দিয়ে উদাহরণ:
Example-1 Nested Array to HTML Table Generation
<?php function createTableHeader($value,$key){ echo "<th>".ucfirst($key)."</th>"; } function print_row($item) { echo('<tr>'); array_walk($item, 'print_cell'); echo('</tr>'); } function print_cell($item) { echo('<td>'); echo($item); echo('</td>'); } $students=[ ["id"=>1,"name"=>"Masud Alam", "age"=>36], ["id"=>2,"name"=>"Sohel Alam", "age"=>32], ["id"=>3,"name"=>"sahed Alam", "age"=>39] ]; ?> <table border="1"> <?php array_walk($students[0], 'createTableHeader'); ?> <?php array_walk($students, 'print_row');?> </table>
Example-2 Nested Array to HTML Table Generation
<?php function createTableHeader($value,$key){ echo "<th>".ucfirst($key)."</th>"; } function print_row($item) { echo('<tr>'); echo('<td>'); echo(implode('</td><td>', $item)); echo('</td>'); echo('</tr>'); } function print_cell($item) { echo('<td>'); echo($item); echo('</td>'); } $students=[ ["id"=>1,"name"=>"Masud Alam", "age"=>36], ["id"=>2,"name"=>"Sohel Alam", "age"=>32], ["id"=>3,"name"=>"sahed Alam", "age"=>39] ]; echo "<table border=\"1\">"; array_walk($students[0], 'createTableHeader'); array_walk($students, 'print_row'); echo "</table>"; ?>
Example-3 Single Array to HTML Table Generation
<?php function getTable($value,$key){ echo "<tr>"; echo "<th>$key</th><td>$value</td>"; echo "</tr>"; } $student=["id"=>1,"name"=>"Masud Alam", "age"=>36]; echo "<table border=\"1\">"; echo array_walk($student, "getTable"); echo "</table>";
Another example
<?php // Sample array of user data $users = [ ['id' => 1, 'name' => 'John', 'age' => 25], ['id' => 2, 'name' => 'Jane', 'age' => 30], ['id' => 3, 'name' => 'Alice', 'age' => 22], ['id' => 4, 'name' => 'Bob', 'age' => 28], ]; // Bonus to add to each user's age $bonusAge = 2; // Callback function to add a bonus to the user's age function addAgeBonus(&$user, $key, $bonusAge) { $user['age'] += $bonusAge; } // Use array_walk to add the bonus to each user's age array_walk($users, 'addAgeBonus', $bonusAge); // Display the modified array print_r($users); ?>
Another Example
<?php // Sample array of product prices $productPrices = [50.99, 29.95, 99.99, 149.99, 79.99]; // Tax rate to apply to each product price $taxRate = 0.08; // Callback function to calculate the total price after applying tax function calculateTotalPrice(&$price, $key, $taxRate) { $price += $price * $taxRate; } // Use array_walk to apply the tax to each product price array_walk($productPrices, 'calculateTotalPrice', $taxRate); // Display the modified array print_r($productPrices); ?>
Another Example
<?php // Sample array of file names $fileNames = [ 'Document File.txt', 'Image File.jpg', 'Data File.csv', 'Presentation File.pptx', 'Script File.js', ]; // Callback function to normalize file names function normalizeFileName(&$fileName, $key) { // Convert to lowercase and replace spaces with underscores $fileName = strtolower(str_replace(' ', '_', $fileName)); } // Use array_walk to normalize each file name array_walk($fileNames, 'normalizeFileName'); // Display the modified array print_r($fileNames); ?>
array_walk_recursive Function
Nested array_walk_recursive ফাঙ্কশন টি array_walk ফাঙ্কশনের মতোই Array এর প্রত্যেকটি Member এর উপর user এর বা প্রোগ্রামারের দেওয়া callback function ব্যবহার করার জন্য ব্যবহৃত হয়, তবে সেটি single array এর পরিবর্তে Nested array এর উপর কাজ করে। এটিও Nested array এর মধ্যের value গুলোকে user define callback function এর প্রথম Parameter হিসেবে পাস করে , আর key গুলোকে দ্বিতীয় Parameter হিসেবে পাস করে। চলুন একটা উদাহরণ দিয়ে ব্যাপারটা আরো ভালোভাবে বুঝে নেয়া যাক :
<?php $fruits = ['sweet' => ['a' => 'apple', 'b' => 'banana'], 'sour' => 'lemon']; function test_print($item, $key) { echo "$key holds $item \n"; } echo "<pre>"; array_walk_recursive($fruits, 'test_print'); echo "</pre>"; ?>
Output
a holds apple b holds banana sour holds lemon
Example#2
<?php // Sample multidimensional array representing user preferences $userPreferences = [ 'username' => 'john_doe', 'location' => [ 'latitude' => 37.7749, 'longitude' => -122.4194, ], 'distance_units' => 'miles', 'favorites' => [ 'movies' => [ 'Inception' => 3, 'The Matrix' => 5, 'Interstellar' => 8, ], 'books' => [ '1984' => 10, 'To Kill a Mockingbird' => 15, ], ], ]; // Conversion factor from miles to kilometers $milesToKilometers = 1.60934; // Callback function to convert numerical values from miles to kilometers function convertMilesToKilometers(&$value, $key) { if (is_numeric($value)) { $value *= $GLOBALS['milesToKilometers']; } } // Use array_walk_recursive to apply the conversion array_walk_recursive($userPreferences, 'convertMilesToKilometers'); // Display the modified user preferences array print_r($userPreferences); ?>
Example#3
<?php // Sample nested array representing a directory structure with file sizes in bytes $directoryStructure = [ 'folder1' => [ 'file1.txt' => 1024, 'file2.jpg' => 204800, ], 'folder2' => [ 'file3.pdf' => 512000, 'file4.png' => 3072, ], ]; // Callback function to convert file sizes to a human-readable format function convertFileSize(&$value, $key) { if (is_numeric($value)) { $value = formatFileSize($value); } } // Function to format file size in a human-readable format function formatFileSize($sizeInBytes) { $units = ['B', 'KB', 'MB', 'GB', 'TB']; $index = 0; while ($sizeInBytes >= 1024 && $index < count($units) - 1) { $sizeInBytes /= 1024; $index++; } return round($sizeInBytes, 2) . ' ' . $units[$index]; } // Use array_walk_recursive to apply the conversion array_walk_recursive($directoryStructure, 'convertFileSize'); // Display the modified directory structure array print_r($directoryStructure); ?>
array_reduce() Function
PHP তে array এর সব গুলো element কে callback ফাঙ্কশনের মাধ্যমে একটা single Value তে রূপান্তর করার জন্য PHP তে array_reduce function টি ব্যবহৃত হয়। এক্ষেত্রে callback function টি তে দুটি Parameter দিতে হয়, তারমধ্যে প্রথমটি carry হিসেবে ব্যবহৃত হয় এবং দ্বিতীয়টি array এর প্রত্যেকটি item গ্রহণ করার জন্য ব্যবহৃত হয়। চলুন একটা উদাহরণ দেখা যাক :
<?php function sum($carry, $item) { $carry += $item; return $carry; } function product($carry, $item) { $carry *= $item; return $carry; } $a = array(1, 2, 3, 4, 5); $x = array(); echo "<pre>"; var_dump(array_reduce($a, "sum")); // int(15) var_dump(array_reduce($a, "product", 10)); // int(1200), because: 10*1*2*3*4*5 var_dump(array_reduce($x, "sum", "No data to reduce")); // string(17) "No data to reduce" echo "</pre>"; ?>
Output
int(15) int(1200) string(17) "No data to reduce"
Example #2
<?php
// Sample array representing orders
$orders = [
['product' => 'Laptop', 'price' => 1200],
['product' => 'Phone', 'price' => 800],
['product' => 'Headphones', 'price' => 100],
['product' => 'Mouse', 'price' => 25],
];
// Callback function to calculate the total order amount
function calculateTotalAmount($carry, $order) {
return $carry + $order['price'];
}
// Use array_reduce to calculate the total order amount
$totalAmount = array_reduce($orders, 'calculateTotalAmount', 0);
// Display the total order amount
echo "Total Order Amount: $totalAmount";
?>
Example #3
<?php
// Sample array representing students and their grades
$students = [
['name' => 'Alice', 'grades' => [90, 85, 92, 88]],
['name' => 'Bob', 'grades' => [78, 95, 88, 92]],
['name' => 'Charlie', 'grades' => [85, 90, 87, 94]],
// ... more students
];
// Callback function to calculate the average grade for a student
function calculateStudentAverage($carry, $student) {
$average = array_sum($student['grades']) / count($student['grades']);
$carry[] = ['name' => $student['name'], 'average' => $average];
return $carry;
}
// Use array_reduce to calculate the average grade for each student
$studentAverages = array_reduce($students, 'calculateStudentAverage', []);
// Display the average grade for each student
echo "Student Averages:\n";
foreach ($studentAverages as $studentAverage) {
echo "{$studentAverage['name']}: {$studentAverage['average']}\n";
}
// Callback function to calculate the overall class average
function calculateClassAverage($carry, $student) {
return $carry + $student['average'];
}
// Use array_reduce to calculate the overall class average
$classAverage = array_reduce($studentAverages, 'calculateClassAverage', 0) / count($studentAverages);
// Display the overall class average
echo "\nClass Average: $classAverage\n";
?>
Example #4
<?php
// Sample array representing employees and their compensation
$employees = [
['name' => 'Alice', 'salary' => 60000, 'bonus' => 5000],
['name' => 'Bob', 'salary' => 75000, 'bonus' => 8000],
['name' => 'Charlie', 'salary' => 90000, 'bonus' => 6000],
// ... more employees
];
// Callback function to calculate the total compensation for an employee
function calculateEmployeeCompensation($carry, $employee) {
$totalCompensation = $employee['salary'] + $employee['bonus'];
$carry[] = ['name' => $employee['name'], 'total_compensation' => $totalCompensation];
return $carry;
}
// Use array_reduce to calculate the total compensation for each employee
$employeeCompensations = array_reduce($employees, 'calculateEmployeeCompensation', []);
// Display the total compensation for each employee
echo "Employee Compensations:\n";
foreach ($employeeCompensations as $employeeCompensation) {
echo "{$employeeCompensation['name']}: {$employeeCompensation['total_compensation']}\n";
}
// Callback function to calculate the overall company expenditure on compensation
function calculateCompanyExpenditure($carry, $employee) {
return $carry + $employee['total_compensation'];
}
// Use array_reduce to calculate the overall company expenditure on compensation
$companyExpenditure = array_reduce($employeeCompensations, 'calculateCompanyExpenditure', 0);
// Display the overall company expenditure on compensation
echo "\nCompany Expenditure on Compensation: $companyExpenditure\n";
?>
array_chunk() Function
একটি নির্দিষ্ট array কে একাধিক array তে বিভক্ত করার জন্য PHP তে array_chunk() function টি ব্যবহৃত হয়। array_chunk() ফাঙ্কশনে প্রথম Parameter টি থাকে যেই array টি একাধিক array বিভক্ত হবে সেটি receive করবে , দ্বিতীয় array টি ব্যবহৃত হবে array টি কতটি খন্ডে বিভক্ত হবে সেটির জন্য , আর তৃতীয় parameter টি ব্যবহৃত হয় খন্ডে খন্ডে বিভক্ত array গুলোতে আগের key থাকবে কিনা তা নির্ধারণের জন্য , আগের index রাখার জন্য আপনাকে তৃতীয় Parameter টি true করে দিতে হবে। বাই ডিফল্ট তৃতীয় Parameter টি false থাকে। চলুন একটা উদাহরণ দিয়ে বিষয়টা বুঝে নেয়া যাক :
<?php $input_array = array('a', 'b', 'c', 'd', 'e'); print_r(array_chunk($input_array, 2)); print_r(array_chunk($input_array, 2, true)); ?>
Output
Array ( [0] => Array ( [0] => a [1] => b ) [1] => Array ( [0] => c [1] => d ) [2] => Array ( [0] => e ) ) Array ( [0] => Array ( [0] => a [1] => b ) [1] => Array ( [2] => c [3] => d ) [2] => Array ( [4] => e ) )
লক্ষ্য করুন, প্রথম array_chunk টিতে তৃতীয় parameter টি true না দেওয়ায় নতুন array গুলোর প্রত্যেকটির পূর্বের key এর পরিবর্তে নতুন key assign হয়েছে। আর দ্বিতীয় array_chunk() ফাঙ্কশনে তৃতীয় Parameter true দেওয়ায়, প্রতিটি নতুন array এর key তে তাদের আগের key গুলোই রয়েছে।
Example # 2
<?php // Sample array representing user data $userData = [ ['id' => 1, 'name' => 'Alice', 'email' => 'alice@example.com'], ['id' => 2, 'name' => 'Bob', 'email' => 'bob@example.com'], ['id' => 3, 'name' => 'Charlie', 'email' => 'charlie@example.com'], ['id' => 4, 'name' => 'David', 'email' => 'david@example.com'], ['id' => 5, 'name' => 'Eva', 'email' => 'eva@example.com'], ['id' => 6, 'name' => 'Frank', 'email' => 'frank@example.com'], // ... more user data ]; // Number of users to display per page $usersPerPage = 3; // Use array_chunk to split the user data into paginated chunks $paginatedUserData = array_chunk($userData, $usersPerPage); // Simulate displaying each page foreach ($paginatedUserData as $pageIndex => $pageData) { echo "Page $pageIndex:\n"; foreach ($pageData as $user) { echo "{$user['id']}: {$user['name']} ({$user['email']})\n"; } echo "\n"; } ?>
array_slice() Function
একটি নির্দিষ্ট array এর একটা নির্দিষ্ট অংশ কেটে নিয়ে নতুন array তৈরী করার জন্য PHP তে array_slice() function টি ব্যবহৃত হয়। array_slice() ফাঙ্কশনে চারটি Parameter ব্যবহৃত হয়, প্রথমটি ব্যবহৃত হয় যেই array কে slice করবেন সেটার জন্য , দ্বিতীয় Parameter টি Offset অর্থাৎ কোথায় থেকে slice শুরু হবে তার জন্য , তৃতীয় Parameter slicing করা array এর length এর জন্য, অর্থাৎ পূর্বের array এর কত গুলো value নিয়ে নতুন array তৈরী হবে তার জন্য । আর সর্বশেষ Parameter টি ব্যবহৃত হয় আগের key থাকবে কিনা তার জন্য, বাই ডিফল্ট false থাকে। চলুন একটা উদাহরণ দিয়ে আরো ভালো ভাবে বুঝে নেয়া যাক :
<?php $input = array("a", "b", "c", "d", "e"); $output = array_slice($input, 2); // returns "c", "d", and "e" $output = array_slice($input, -2, 1); // returns "d" $output = array_slice($input, 0, 3); // returns "a", "b", and "c" // note the differences in the array keys print_r(array_slice($input, 2, -1)); print_r(array_slice($input, 2, -1, true)); ?>
Output
Array ( [0] => c [1] => d ) Array ( [2] => c [3] => d )
Example #2
<?php // Sample array representing articles $articles = [ ['id' => 101, 'title' => 'Introduction to PHP', 'content' => '...'], ['id' => 102, 'title' => 'Web Development Basics', 'content' => '...'], ['id' => 103, 'title' => 'Database Design Principles', 'content' => '...'], ['id' => 104, 'title' => 'Responsive Web Design Techniques', 'content' => '...'], ['id' => 105, 'title' => 'PHP Error Handling', 'content' => '...'], ['id' => 106, 'title' => 'Building RESTful APIs', 'content' => '...'], // ... more articles ]; // Number of articles to display per page $articlesPerPage = 3; // Current page number (considering a user navigating pages) $currentPage = 2; // Use array_slice to get the articles for the current page $startIndex = ($currentPage - 1) * $articlesPerPage; $currentPageArticles = array_slice($articles, $startIndex, $articlesPerPage); // Simulate displaying the articles on the current page echo "Articles on Page $currentPage:\n"; foreach ($currentPageArticles as $article) { echo "{$article['id']}: {$article['title']}\n"; // Simulate displaying article content } ?>
array_column() Functions
Nested Array এর মধ্যের array গুলোর একটি নির্দিষ্ট column এর বিপরীতে সব গুলো value কে print করার জন্য PHP তে array_column() ফাঙ্কশন টি ব্যবহৃত হয়। চলুন একটি উদাহরণ দিয়ে বুঝে নেয়া যাক :
<?php // Array representing a possible record set returned from a database $records = array( array( 'id' => 2135, 'first_name' => 'John', 'last_name' => 'Doe', ), array( 'id' => 3245, 'first_name' => 'Sally', 'last_name' => 'Smith', ), array( 'id' => 5342, 'first_name' => 'Jane', 'last_name' => 'Jones', ), array( 'id' => 5623, 'first_name' => 'Peter', 'last_name' => 'Doe', ) ); $first_names = array_column($records, 'first_name'); print_r($first_names); ?>
Output
Array ( [0] => John [1] => Sally [2] => Jane [3] => Peter )
আপনি চাইলে তৃতীয় আরেকটি Parameter ব্যবহার করতে পারেন। সে ক্ষেত্রে দ্বিতীয় প্যারামিটার টি তে যেই column name দিবেন সেটির value গুলো আপনার নতুন তৈরী array এর value হিসেবে print হবে। আর তৃতীয় Parameter টি তে যেই column name দিবেন সেটির value গুলো আপনার নতুন তৈরী array এর key হিসেবে print হবে। চলুন একটি উদাহরণ দিয়ে বুঝে নেয়া যাক :
<?php // Array representing a possible record set returned from a database $records = array( array( 'id' => 2135, 'first_name' => 'John', 'last_name' => 'Doe', ), array( 'id' => 3245, 'first_name' => 'Sally', 'last_name' => 'Smith', ), array( 'id' => 5342, 'first_name' => 'Jane', 'last_name' => 'Jones', ), array( 'id' => 5623, 'first_name' => 'Peter', 'last_name' => 'Doe', ) ); $last_names = array_column($records, 'last_name', 'id'); echo "<pre>"; print_r($last_names); echo "</pre>"; ?>
Output
Array ( [2135] => Doe [3245] => Smith [5342] => Jones [5623] => Doe )
লক্ষ্য করুন, এখানে দ্বিতীয় Parameter টি নতুন তৈরী array এর value হিসেবে print হয়েছে , এবং তৃতীয় Parameter টি নতুন তৈরী array এর key হিসেবে print হয়েছে।
Another Example
<?php // Sample array representing order data $orderData = [ ['order_id' => 101, 'items' => [['product' => 'Laptop', 'quantity' => 2, 'price' => 1200], ['product' => 'Mouse', 'quantity' => 1, 'price' => 25]]], ['order_id' => 102, 'items' => [['product' => 'Smartphone', 'quantity' => 3, 'price' => 800], ['product' => 'Tablet', 'quantity' => 1, 'price' => 500]]], ['order_id' => 103, 'items' => [['product' => 'Headphones', 'quantity' => 2, 'price' => 100], ['product' => 'Laptop', 'quantity' => 1, 'price' => 1200]]], // ... more order data ]; // Use array_column to flatten the 'items' array and extract product names and total prices $flattenedItems = array_merge(...array_column($orderData, 'items')); // Use array_column to group items by product name and calculate total sales $productSales = []; foreach ($flattenedItems as $item) { $productName = $item['product']; $quantity = $item['quantity']; $price = $item['price']; if (!isset($productSales[$productName])) { $productSales[$productName] = ['total_quantity' => 0, 'total_sales' => 0]; } $productSales[$productName]['total_quantity'] += $quantity; $productSales[$productName]['total_sales'] += ($quantity * $price); } // Simulate displaying the total sales for each product echo "Product Sales:\n"; foreach ($productSales as $productName => $salesData) { $totalQuantity = $salesData['total_quantity']; $totalSales = $salesData['total_sales']; echo "$productName - Total Quantity: $totalQuantity, Total Sales: $totalSales\n"; } ?>
array_combine() Functions
দুটি array এর মধ্যে একটিকে key হিসেবে এবং অন্যটিকে value হিসেবে combine করে নতুন array তৈরী করার জন্য PHP তে array_combine() function টি ব্যবহৃত হয়। চলুন একটা উদাহরণ দেখা যাক :
<?php $a = array('green', 'red', 'yellow'); $b = array('avocado', 'apple', 'banana'); $c = array_combine($a, $b); echo "<pre>"; print_r($c); echo "</pre>"; ?>
Output
Array ( [green] => avocado [red] => apple [yellow] => banana )
Another Example
<?php // Sample arrays representing product IDs and names $productIds = [101, 102, 103, 104, 105]; $productNames = ['Laptop', 'Smartphone', 'Headphones', 'Mouse', 'Tablet']; // Use array_combine to create an associative array with product IDs as keys and product names as values $productAssociativeArray = array_combine($productIds, $productNames); // Simulate displaying the resulting associative array echo "Product ID to Name Mapping:\n"; foreach ($productAssociativeArray as $productId => $productName) { echo "Product ID: $productId, Product Name: $productName\n"; } ?>
array_merge() Function
দুই বা ততোধিক array কে একটি array তে Merge করার জন্য PHP তে array_merge() function টি ব্যবহৃত হয়। চলুন একটা উদাহরণ দেখা যাক :
<?php $array1 = array("a","b","c","d"); $array2 = array("e","f","g","h"); $result = array_merge($array1, $array2); echo "<pre>"; print_r($result); echo "</pre>"; ?>
Output
Array ( [0] => a [1] => b [2] => c [3] => d [4] => e [5] => f [6] => g [7] => h )
উল্লেখ্য , যদি Merge করা দুটি array তেই একই string index থাকে, সেক্ষেত্রে দুটি array এর মধ্যে প্রথম array এর একই string index এর value টি overwrite হয়ে হয়ে যাবে। নিচের উদাহরণ লক্ষ্য করুন :
<?php $array1 = array("a"=>"America","b","c","d"); $array2 = array("a"=>"England","f","g","h"); $result = array_merge($array1, $array2); echo "<pre>"; print_r($result); echo "</pre>"; ?>
Output
Array ( [a] => England [0] => b [1] => c [2] => d [3] => f [4] => g [5] => h )
লক্ষ্য করুন , এখানে প্রথম array এর ভ্যালু ‘America’ Overwrite হয়ে গেছে।
Another Example
// Sample arrays $array1 = array('name' => 'John', 'age' => 25, 'city' => 'New York'); $array2 = array('occupation' => 'Engineer', 'city' => 'San Francisco', 'country' => 'USA'); // Merge arrays using array_merge $mergedArray = array_merge($array1, $array2); // Display the merged array print_r($mergedArray);
Output
Array ( [name] => John [age] => 25 [city] => San Francisco // Note: Value from $array2 overwrites value from $array1 for the key 'city' [occupation] => Engineer [country] => USA )
array_merge_recursive() Function
দুটি Nested Array কে Merge করে নতুন একটি array তৈরী করার জন্য PHP তে array_merge_recursive() function টি ব্যবহৃত হয়। নিচের উদাহরণটি দেখুন :
<?php $ar1 = array("color" => array("favorite" => "red"), 5); $ar2 = array(10, "color" => array("favorite" => "green", "blue")); $result = array_merge_recursive($ar1, $ar2); print_r($result); ?>
Output:
Array ( [color] => Array ( [favorite] => Array ( [0] => red [1] => green ) [0] => blue ) [0] => 5 [1] => 10 )
Another Example
// Sample arrays $array1 = array('name' => 'John', 'contacts' => array('email' => 'john@example.com', 'phone' => '123456789')); $array2 = array('age' => 25, 'contacts' => array('phone' => '987654321', 'address' => '123 Main St')); // Merge arrays using array_merge_recursive $mergedArray = array_merge_recursive($array1, $array2); // Display the merged array print_r($mergedArray);
Output
Array ( [name] => John [contacts] => Array ( [email] => john@example.com [phone] => Array ( [0] => 123456789 [1] => 987654321 ) [address] => 123 Main St ) [age] => 25 )
Another Example
// Sample arrays representing menu categories $menu1 = array( 'appetizers' => array('spring rolls', 'bruschetta'), 'main_courses' => array('grilled chicken', 'pasta carbonara'), 'desserts' => 'chocolate cake' ); $menu2 = array( 'appetizers' => array('shrimp cocktail', 'stuffed mushrooms'), 'main_courses' => array('steak', 'vegetarian lasagna'), 'drinks' => 'red wine' ); // Merge arrays using array_merge_recursive $mergedMenu = array_merge_recursive($menu1, $menu2); // Display the merged menu print_r($mergedMenu);
Output
Array ( [appetizers] => Array ( [0] => spring rolls [1] => bruschetta [2] => shrimp cocktail [3] => stuffed mushrooms ) [main_courses] => Array ( [0] => grilled chicken [1] => pasta carbonara [2] => steak [3] => vegetarian lasagna ) [desserts] => chocolate cake [drinks] => red wine )
array_push() function
PHP তে কোনো একটা array এর শেষে এক বা একাধিক value (ঢুকানোর) পুশ করার জন্য array_push() function টি ব্যবহৃত হয়। চলুন উদাহরণ দেখা যাক :
<?php $stack = array("orange", "banana"); array_push($stack, "apple", "raspberry"); print_r($stack); ?>
Output
Array ( [0] => orange [1] => banana [2] => apple [3] => raspberry )
Another Example
// Sample shopping cart array $shoppingCart = array(); // Function to add items to the cart function addToCart($product, $price, $quantity) { global $shoppingCart; // Create an associative array representing the item $item = array( 'product' => $product, 'price' => $price, 'quantity' => $quantity ); // Use array_push to add the item to the shopping cart array_push($shoppingCart, $item); echo "$quantity $product(s) added to the cart.\n"; } // Add items to the cart using the addToCart function addToCart('T-shirt', 19.99, 2); addToCart('Jeans', 39.99, 1); addToCart('Shoes', 49.99, 1); // Display the contents of the shopping cart print_r($shoppingCart);
Output:
2 T-shirt(s) added to the cart. 1 Jeans(s) added to the cart. 1 Shoes(s) added to the cart. Array ( [0] => Array ( [product] => T-shirt [price] => 19.99 [quantity] => 2 ) [1] => Array ( [product] => Jeans [price] => 39.99 [quantity] => 1 ) [2] => Array ( [product] => Shoes [price] => 49.99 [quantity] => 1 ) )
Another Example
// Sample conversation array $conversation = array(); // Function to add messages to the conversation function addMessage($sender, $content) { global $conversation; // Create an associative array representing the message $message = array( 'sender' => $sender, 'timestamp' => date('Y-m-d H:i:s'), // Current timestamp 'content' => $content ); // Use array_push to add the message to the conversation array_push($conversation, $message); echo "New message from $sender added to the conversation.\n"; } // Add messages to the conversation using the addMessage function addMessage('Alice', 'Hi, how are you?'); addMessage('Bob', 'I\'m good, thanks! How about you?'); addMessage('Alice', 'Doing well, thanks for asking.'); // Display the contents of the conversation print_r($conversation);
Output:
New message from Alice added to the conversation. New message from Bob added to the conversation. New message from Alice added to the conversation. Array ( [0] => Array ( [sender] => Alice [timestamp] => 2023-12-15 12:30:00 [content] => Hi, how are you? ) [1] => Array ( [sender] => Bob [timestamp] => 2023-12-15 12:31:00 [content] => I'm good, thanks! How about you? ) [2] => Array ( [sender] => Alice [timestamp] => 2023-12-15 12:32:00 [content] => Doing well, thanks for asking. ) )
array_pop() function
PHP তে কোনো একটা array এর শেষ থেকে একটি value বের করে দেওয়ার array_pop() function টি ব্যবহৃত হয়। চলুন উদাহরণ দেখা যাক :
<?php $stack = array("orange", "banana", "apple", "raspberry"); $fruit = array_pop($stack); print_r($stack); ?>
Output
Array ( [0] => orange [1] => banana [2] => apple )
Example #2
// Sample shopping cart array $shoppingCart = array(); // Function to add items to the cart function addToCart($product, $price, $quantity) { global $shoppingCart; // Create an associative array representing the item $item = array( 'product' => $product, 'price' => $price, 'quantity' => $quantity ); // Use array_push to add the item to the shopping cart array_push($shoppingCart, $item); echo "$quantity $product(s) added to the cart.\n"; } // Function to undo the last item added to the cart function undoLastItem() { global $shoppingCart; // Use array_pop to remove the last item from the shopping cart $lastItem = array_pop($shoppingCart); if ($lastItem) { echo "$lastItem[quantity] $lastItem[product](s) removed from the cart.\n"; } else { echo "No items to undo.\n"; } } // Add items to the cart using the addToCart function addToCart('T-shirt', 19.99, 2); addToCart('Jeans', 39.99, 1); addToCart('Shoes', 49.99, 1); // Display the contents of the shopping cart print_r($shoppingCart); // Undo the last item undoLastItem(); // Display the contents of the shopping cart after undo print_r($shoppingCart);
Output
2 T-shirt(s) added to the cart. 1 Jeans(s) added to the cart. 1 Shoes(s) added to the cart. Array ( [0] => Array ( [product] => T-shirt [price] => 19.99 [quantity] => 2 ) [1] => Array ( [product] => Jeans [price] => 39.99 [quantity] => 1 ) [2] => Array ( [product] => Shoes [price] => 49.99 [quantity] => 1 ) ) 1 Shoes(s) removed from the cart. Array ( [0] => Array ( [product] => T-shirt [price] => 19.99 [quantity] => 2 ) [1] => Array ( [product] => Jeans [price] => 39.99 [quantity] => 1 ) )
Example #3
// Sample conversation array $conversation = array(); // Function to add messages to the conversation function addMessage($sender, $content) { global $conversation; // Create an associative array representing the message $message = array( 'sender' => $sender, 'timestamp' => date('Y-m-d H:i:s'), // Current timestamp 'content' => $content ); // Use array_push to add the message to the conversation array_push($conversation, $message); echo "New message from $sender added to the conversation.\n"; } // Function to remove the last message from the conversation function undoLastMessage() { global $conversation; // Use array_pop to remove the last message from the conversation $lastMessage = array_pop($conversation); if ($lastMessage) { echo "Message from {$lastMessage['sender']} removed from the conversation.\n"; } else { echo "No messages to undo.\n"; } } // Add messages to the conversation using the addMessage function addMessage('Alice', 'Hi, how are you?'); addMessage('Bob', 'I\'m good, thanks! How about you?'); addMessage('Alice', 'Doing well, thanks for asking.'); // Display the contents of the conversation print_r($conversation); // Undo the last message undoLastMessage(); // Display the contents of the conversation after undo print_r($conversation);
Output:
New message from Alice added to the conversation. New message from Bob added to the conversation. New message from Alice added to the conversation. Array ( [0] => Array ( [sender] => Alice [timestamp] => 2023-12-15 12:30:00 [content] => Hi, how are you? ) [1] => Array ( [sender] => Bob [timestamp] => 2023-12-15 12:31:00 [content] => I'm good, thanks! How about you? ) [2] => Array ( [sender] => Alice [timestamp] => 2023-12-15 12:32:00 [content] => Doing well, thanks for asking. ) ) Message from Alice removed from the conversation. Array ( [0] => Array ( [sender] => Alice [timestamp] => 2023-12-15 12:30:00 [content] => Hi, how are you? ) [1] => Array ( [sender] => Bob [timestamp] => 2023-12-15 12:31:00 [content] => I'm good, thanks! How about you? ) )
array_unshift() function
PHP তে কোনো একটা array এর শুরুতে এক বা একাধিক value ঢুকানোর জন্য array_unshift() function টি ব্যবহৃত হয়। চলুন উদাহরণ দেখা যাক :
<?php $queue = array("orange", "banana"); array_unshift($queue, "apple", "raspberry"); print_r($queue); ?>
Output
Array ( [0] => apple [1] => raspberry [2] => orange [3] => banana )
Example #2: Latest Task
// Sample task list array $taskList = array(); // Function to add tasks to the beginning of the list function addTask($name, $description) { global $taskList; // Create an associative array representing the task $task = array( 'name' => $name, 'description' => $description, 'status' => 'pending' ); // Use array_unshift to add the task to the beginning of the list array_unshift($taskList, $task); echo "Task \"$name\" added to the top of the list.\n"; } // Add tasks to the list using the addTask function addTask('Write report', 'Draft a report on the quarterly performance.'); addTask('Review code', 'Review the code for the upcoming release.'); // Display the contents of the task list print_r($taskList); // Add a new task to the top of the list addTask('Plan meeting', 'Schedule a team meeting for next week.'); // Display the contents of the task list after the new addition print_r($taskList);
Output
Task "Write report" added to the top of the list. Task "Review code" added to the top of the list. Array ( [0] => Array ( [name] => Review code [description] => Review the code for the upcoming release. [status] => pending ) [1] => Array ( [name] => Write report [description] => Draft a report on the quarterly performance. [status] => pending ) ) Task "Plan meeting" added to the top of the list. Array ( [0] => Array ( [name] => Plan meeting [description] => Schedule a team meeting for next week. [status] => pending ) [1] => Array ( [name] => Review code [description] => Review the code for the upcoming release. [status] => pending ) [2] => Array ( [name] => Write report [description] => Draft a report on the quarterly performance. [status] => pending ) )
Example #3: latest news articles
// Sample array representing a list of news articles $newsArticles = array(); // Function to add news articles to the beginning of the list function addNewsArticle($title, $content) { global $newsArticles; // Create an associative array representing the news article $article = array( 'title' => $title, 'content' => $content, 'timestamp' => date('Y-m-d H:i:s') ); // Use array_unshift to add the news article to the beginning of the list array_unshift($newsArticles, $article); echo "News article \"$title\" added to the top of the list.\n"; } // Add news articles to the list using the addNewsArticle function addNewsArticle('New Discovery in Science', 'Scientists make groundbreaking discovery in the field of astrophysics.'); addNewsArticle('Tech Innovation Expo', 'Cutting-edge technologies showcased at the annual Tech Innovation Expo.'); // Display the contents of the news article list print_r($newsArticles); // Add a new news article to the top of the list addNewsArticle('Environmental Sustainability Initiative', 'Initiative launched to promote environmental sustainability.'); // Display the contents of the news article list after the new addition print_r($newsArticles);
Output:
News article "New Discovery in Science" added to the top of the list. News article "Tech Innovation Expo" added to the top of the list. Array ( [0] => Array ( [title] => Tech Innovation Expo [content] => Cutting-edge technologies showcased at the annual Tech Innovation Expo. [timestamp] => 2023-12-15 14:00:00 ) [1] => Array ( [title] => New Discovery in Science [content] => Scientists make groundbreaking discovery in the field of astrophysics. [timestamp] => 2023-12-15 13:45:00 ) ) News article "Environmental Sustainability Initiative" added to the top of the list. Array ( [0] => Array ( [title] => Environmental Sustainability Initiative [content] => Initiative launched to promote environmental sustainability. [timestamp] => 2023-12-15 14:15:00 ) [1] => Array ( [title] => Tech Innovation Expo [content] => Cutting-edge technologies showcased at the annual Tech Innovation Expo. [timestamp] => 2023-12-15 14:00:00 ) [2] => Array ( [title] => New Discovery in Science [content] => Scientists make groundbreaking discovery in the field of astrophysics. [timestamp] => 2023-12-15 13:45:00 ) )
array_shift() function
PHP তে কোনো একটা array এর শুরুতে একটি value বের করে দেওয়ার array_shift() function টি ব্যবহৃত হয়। চলুন উদাহরণ দেখা যাক :
<?php $stack = array("orange", "banana", "apple", "raspberry"); $fruit = array_shift($stack); print_r($stack); ?>
Output
Array ( [0] => banana [1] => apple [2] => raspberry )
Example #2: Remove Oldest Task
// Sample task list array $taskList = array(); // Function to add tasks to the list function addTask($name, $description) { global $taskList; // Create an associative array representing the task $task = array( 'name' => $name, 'description' => $description, 'status' => 'pending' ); // Use array_push to add the task to the end of the list array_push($taskList, $task); echo "Task \"$name\" added to the list.\n"; } // Function to process the oldest task in the list function processOldestTask() { global $taskList; // Check if there are tasks in the list if (empty($taskList)) { echo "No tasks to process.\n"; return; } // Use array_shift to retrieve and remove the oldest task from the list $oldestTask = array_shift($taskList); echo "Processing task \"$oldestTask[name]\"...\n"; } // Add tasks to the list using the addTask function addTask('Write report', 'Draft a report on the quarterly performance.'); addTask('Review code', 'Review the code for the upcoming release.'); // Display the contents of the task list print_r($taskList); // Process the oldest task processOldestTask(); // Display the contents of the task list after processing print_r($taskList);
Output:
Task "Write report" added to the list. Task "Review code" added to the list. Array ( [0] => Array ( [name] => Write report [description] => Draft a report on the quarterly performance. [status] => pending ) [1] => Array ( [name] => Review code [description] => Review the code for the upcoming release. [status] => pending ) ) Processing task "Write report"... Array ( [0] => Array ( [name] => Review code [description] => Review the code for the upcoming release. [status] => pending ) )
Example #3: display the oldest unread message
// Sample inbox array for a user $userInbox = array(); // Function to add messages to the inbox function addMessageToInbox($sender, $content) { global $userInbox; // Create an associative array representing the message $message = array( 'sender' => $sender, 'content' => $content, 'timestamp' => date('Y-m-d H:i:s'), 'read' => false ); // Use array_push to add the message to the end of the inbox array_push($userInbox, $message); echo "New message from $sender added to the inbox.\n"; } // Function to retrieve and display the oldest unread message function displayOldestUnreadMessage() { global $userInbox; // Check if there are unread messages in the inbox $unreadMessages = array_filter($userInbox, function ($message) { return !$message['read']; }); if (empty($unreadMessages)) { echo "No unread messages in the inbox.\n"; return; } // Use array_shift to retrieve and remove the oldest unread message from the inbox $oldestUnreadMessage = array_shift($unreadMessages); echo "Displaying oldest unread message from {$oldestUnreadMessage['sender']}:\n"; echo "{$oldestUnreadMessage['content']}\n"; // Mark the message as read $oldestUnreadMessage['read'] = true; } // Add messages to the inbox using the addMessageToInbox function addMessageToInbox('Alice', 'Hi, how are you?'); addMessageToInbox('Bob', 'I\'m good, thanks! How about you?'); addMessageToInbox('Alice', 'Doing well, thanks for asking.'); // Display the contents of the inbox print_r($userInbox); // Display the oldest unread message displayOldestUnreadMessage(); // Display the contents of the inbox after displaying the message print_r($userInbox);
Output
New message from Alice added to the inbox. New message from Bob added to the inbox. New message from Alice added to the inbox. Array ( [0] => Array ( [sender] => Alice [content] => Hi, how are you? [timestamp] => 2023-12-15 15:00:00 [read] => false ) [1] => Array ( [sender] => Bob [content] => I'm good, thanks! How about you? [timestamp] => 2023-12-15 15:01:00 [read] => false ) [2] => Array ( [sender] => Alice [content] => Doing well, thanks for asking. [timestamp] => 2023-12-15 15:02:00 [read] => false ) ) Displaying oldest unread message from Alice: Hi, how are you? Array ( [0] => Array ( [sender] => Bob [content] => I'm good, thanks! How about you? [timestamp] => 2023-12-15 15:01:00 [read] => false ) [1] => Array ( [sender] => Alice [content] => Doing well, thanks for asking. [timestamp] => 2023-12-15 15:02:00 [read] => false ) )
array_splice() function
Array এর মধ্যে এক বা একাধিক element add, replace এবং remove এই ধরণের কাজগুলো করার জন্য PHP তে array_splice() ফাঙ্কশনটি ব্যবহৃত হয়। চলুন কয়েকটি উদাহরণ দিয়ে বুজে নেয়া যাক :
<?php $input = array("red", "green", "blue", "yellow"); array_splice($input, 2); // $input is now array("red", "green") $input = array("red", "green", "blue", "yellow"); array_splice($input, 1, -1); // $input is now array("red", "yellow") $input = array("red", "green", "blue", "yellow"); array_splice($input, 1, count($input), "orange"); // $input is now array("red", "orange") $input = array("red", "green", "blue", "yellow"); array_splice($input, -1, 1, array("black", "maroon")); // $input is now array("red", "green", // "blue", "black", "maroon") $input = array("red", "green", "blue", "yellow"); array_splice($input, 3, 0, "purple"); // $input is now array("red", "green", // "blue", "purple", "yellow"); ?>
Example # 2: add, replace, and delete articles
<?php class Library { private $bookCollection = array(); public function addBook($title, $author) { $book = array('title' => $title, 'author' => $author); array_push($this->bookCollection, $book); echo "Added book: $title by $author\n"; } public function displayBooks() { echo "Current Book Collection:\n"; print_r($this->bookCollection); echo "\n"; } public function addBookAtIndex($index, $title, $author) { $book = array('title' => $title, 'author' => $author); array_splice($this->bookCollection, $index, 0, array($book)); echo "Added book at index $index: $title by $author\n"; } public function replaceBookAtIndex($index, $newTitle, $newAuthor) { if (isset($this->bookCollection[$index])) { $book = array('title' => $newTitle, 'author' => $newAuthor); array_splice($this->bookCollection, $index, 1, array($book)); echo "Replaced book at index $index with: $newTitle by $newAuthor\n"; } else { echo "Index $index is out of bounds.\n"; } } public function removeBookAtIndex($index) { if (isset($this->bookCollection[$index])) { array_splice($this->bookCollection, $index, 1); echo "Removed book at index $index\n"; } else { echo "Index $index is out of bounds.\n"; } } } // Instantiate the Library class $library = new Library(); // Add books to the library $library->addBook('The Catcher in the Rye', 'J.D. Salinger'); $library->addBook('To Kill a Mockingbird', 'Harper Lee'); $library->addBook('1984', 'George Orwell'); // Display the initial book collection $library->displayBooks(); // Add a new book at a specific index $library->addBookAtIndex(1, 'The Great Gatsby', 'F. Scott Fitzgerald'); $library->displayBooks(); // Replace a book at a specific index $library->replaceBookAtIndex(2, 'Brave New World', 'Aldous Huxley'); $library->displayBooks(); // Remove a book at a specific index $library->removeBookAtIndex(0); $library->displayBooks();
Example #3 add, remove, and rearrange songs
class Playlist { private $songs = []; public function __construct(array $initialSongs = []) { $this->songs = $initialSongs; } public function displayPlaylist() { echo "Current Playlist:\n"; print_r($this->songs); echo "\n"; } public function addSong($title, $artist) { $song = ['title' => $title, 'artist' => $artist]; array_push($this->songs, $song); echo "Song \"$title\" by $artist added to the playlist.\n"; } public function removeSong($index) { $removedSongs = array_splice($this->songs, $index, 1); if (!empty($removedSongs)) { echo "Removed song at index $index from the playlist:\n"; print_r($removedSongs); } else { echo "No song found at index $index.\n"; } } public function moveSong($fromIndex, $toIndex) { $songToMove = array_splice($this->songs, $fromIndex, 1); array_splice($this->songs, $toIndex, 0, $songToMove); echo "Moved song from index $fromIndex to index $toIndex in the playlist.\n"; } } // Create a playlist with initial songs $initialSongs = [ ['title' => 'Song A', 'artist' => 'Artist A'], ['title' => 'Song B', 'artist' => 'Artist B'], ['title' => 'Song C', 'artist' => 'Artist C'], ]; $playlist = new Playlist($initialSongs); // Display the initial playlist $playlist->displayPlaylist(); // Add a new song to the playlist $playlist->addSong('Song D', 'Artist D'); $playlist->displayPlaylist(); // Remove a song from the playlist $playlist->removeSong(1); $playlist->displayPlaylist(); // Move a song within the playlist $playlist->moveSong(0, 2); $playlist->displayPlaylist();