PHP Arrays
PHP Arrays পর্ব-৫: PHP Array Difference Functions
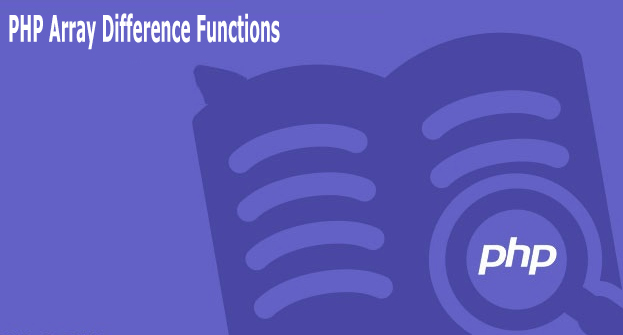
দুই বা ততোধিক array এর মধ্যে বিভিন্ন ধরণের পার্থক্য বের করার জন্য PHP তে ৮ টি function রয়েছে যেগুলোকে বলা হয় PHP Array Difference Functions আজকের পর্বে আমরা PHP array Difference function গুলো নিয়ে আলোচনা করব। চলুন দেখা যাক :
array_diff() Function
PHP তে দুই বা ততোধিক array এর মধ্যে শুধু value এর উপর ভিত্তি করে Difference বের করার জন্য array_diff() function টি ব্যবহৃত হয়। অর্থাৎ, প্রথম array এর যেইসব value গুলো অন্য array গুলোর যেকোনোটির মধ্যে পাওয়া যাবেনা , শুধু সেইসব value গুলো বের করবে। চলুন একটা উদাহরণ দেখা যাক :
<?php $array1 = array("a" => "green", "red", "blue", "red"); $array2 = array("b" => "green", "yellow", "red"); $result = array_diff($array1, $array2); echo "<pre>"; print_r($result); echo "</pre>"; ?>
Output:
Array ( [1] => blue )
Example #2
class Student { private $name; private $courses = []; public function __construct($name) { $this->name = $name; } public function enrollInCourse($course) { $this->courses[] = $course; } public function getCourses() { return $this->courses; } public function getNotEnrolledCourses($allCourses) { return array_diff($allCourses, $this->courses); } public function getName() { return $this->name; } } // List of available courses $allCourses = array("Math", "English", "Science", "History"); // Create a student $student1 = new Student("Alice"); // Enroll the student in some courses $student1->enrollInCourse("Math"); $student1->enrollInCourse("English"); // Get the courses the student is enrolled in $enrolledCourses = $student1->getCourses(); // Use getNotEnrolledCourses to find the courses the student is not enrolled in $notEnrolledCourses = $student1->getNotEnrolledCourses($allCourses); // Display the result echo $student1->getName() . " is enrolled in the following courses:\n"; print_r($enrolledCourses); echo "\n"; echo $student1->getName() . " is not enrolled in the following courses:\n"; print_r($notEnrolledCourses);
Output:
Alice is enrolled in the following courses: Array ( [0] => Math [1] => English ) Alice is not enrolled in the following courses: Array ( [2] => Science [3] => History )
Example #3
class Supplier { private $name; private $products = []; public function __construct($name, array $products) { $this->name = $name; $this->products = $products; } public function getProducts() { return $this->products; } public function getName() { return $this->name; } } class ProductCatalog { public static function findDifference(Supplier $supplierA, Supplier $supplierB) { $productsOnlyInA = array_diff($supplierA->getProducts(), $supplierB->getProducts()); $productsOnlyInB = array_diff($supplierB->getProducts(), $supplierA->getProducts()); return [ 'onlyInA' => $productsOnlyInA, 'onlyInB' => $productsOnlyInB, ]; } } // Create instances of suppliers $supplierA = new Supplier("Supplier A", ["Laptop", "Printer", "Mouse", "Keyboard", "Monitor"]); $supplierB = new Supplier("Supplier B", ["Laptop", "Printer", "Headphones", "Scanner", "Speaker"]); // Use ProductCatalog to find the difference between suppliers $differences = ProductCatalog::findDifference($supplierA, $supplierB); // Display the results echo "Products available in {$supplierA->getName()} but not in {$supplierB->getName()}:\n"; print_r($differences['onlyInA']); echo "\n"; echo "Products available in {$supplierB->getName()} but not in {$supplierA->getName()}:\n"; print_r($differences['onlyInB']);
Output
Products available in Supplier A but not in Supplier B: Array ( [2] => Mouse [3] => Keyboard [4] => Monitor ) Products available in Supplier B but not in Supplier A: Array ( [2] => Headphones [3] => Scanner [4] => Speaker )
array_udiff() Function
PHP তে array_udiff() function টি array_diff() function এর মতোই অর্থাৎ, দুই বা ততোধিক array এর মধ্যে শুধু value এর উপর ভিত্তি করে Difference বের করার জন্য ব্যবহৃত হয় । তবে array_udiff() function কাজটি নিজে করবেনা, বরং user এর supply করা callback function দিয়ে করবে । চলুন একটা উদাহরণ দেখা যাক :
<?php function myfunction($a,$b) { if ($a===$b) { return 0; } return ($a>$b)?1:-1; } $a1=array("a"=>"red","b"=>"green","e"=>"blue","yellow"); $a2=array("A"=>"red","b"=>"GREEN","yellow","black"); $a3=array("a"=>"green","b"=>"red","yellow","black"); $result=array_udiff($a1,$a2,$a3,"myfunction"); echo "<pre>"; print_r($result); echo "</pre>"; ?>
Output:
Array ( [e] => blue )
Example # 2
<?php class Employee { private $id; private $name; private $department; public function __construct($id, $name, $department) { $this->id = $id; $this->name = $name; $this->department = $department; } public function getId() { return $this->id; } public function getName() { return $this->name; } public function getDepartment() { return $this->department; } } class Department { private $employees = []; public function addEmployee(Employee $employee) { $this->employees[] = $employee; } public function getEmployees() { return $this->employees; } public function findEmployeesNotInDepartment(Department $otherDepartment) { $compareEmployeesById = function (Employee $a, Employee $b) { return $a->getId() - $b->getId(); }; return array_udiff($this->employees, $otherDepartment->getEmployees(), $compareEmployeesById); } } // Create employees $employee1 = new Employee(1, 'Alice', 'HR'); $employee2 = new Employee(2, 'Bob', 'IT'); $employee3 = new Employee(3, 'Charlie', 'Marketing'); // Create departments $departmentA = new Department(); $departmentA->addEmployee($employee1); $departmentA->addEmployee($employee2); $departmentB = new Department(); $departmentB->addEmployee($employee2); $departmentB->addEmployee($employee3); // Use the new method to find employees in Department A but not in Department B $employeesOnlyInDepartmentA = $departmentA->findEmployeesNotInDepartment($departmentB); // Use the new method to find employees in Department B but not in Department A $employeesOnlyInDepartmentB = $departmentB->findEmployeesNotInDepartment($departmentA); // Display the results echo "Employees in Department A but not in Department B:\n"; foreach ($employeesOnlyInDepartmentA as $employee) { echo $employee->getName() . " (ID: " . $employee->getId() . ")\n"; } echo "\n"; echo "Employees in Department B but not in Department A:\n"; foreach ($employeesOnlyInDepartmentB as $employee) { echo $employee->getName() . " (ID: " . $employee->getId() . ")\n"; }
Output:
Employees in Department A but not in Department B: Alice (ID: 1) Employees in Department B but not in Department A: Charlie (ID: 3)
Example #3
class Product { private $name; private $price; public function __construct($name, $price) { $this->name = $name; $this->price = $price; } public function getName() { return $this->name; } public function getPrice() { return $this->price; } } class Supplier { private $products = []; public function addProduct(Product $product) { $this->products[] = $product; } public function getProducts() { return $this->products; } public function findProductsWithDifferentPrices(Supplier $otherSupplier) { $compareProductsByName = function (Product $a, Product $b) { return strcmp($a->getName(), $b->getName()); }; return array_udiff($this->products, $otherSupplier->getProducts(), $compareProductsByName); } } // Example usage: // Create products $productA1 = new Product('Laptop', 1200); $productA2 = new Product('Printer', 200); $productA3 = new Product('Mouse', 15); $productB1 = new Product('Laptop', 1300); $productB2 = new Product('Printer', 210); $productB3 = new Product('Keyboard', 30); // Create suppliers $supplierA = new Supplier(); $supplierA->addProduct($productA1); $supplierA->addProduct($productA2); $supplierA->addProduct($productA3); $supplierB = new Supplier(); $supplierB->addProduct($productB1); $supplierB->addProduct($productB2); $supplierB->addProduct($productB3); // Use the new method to find products with price differences between suppliers $differentPricesProducts = $supplierA->findProductsWithDifferentPrices($supplierB); // Display the results echo "Products with different prices between Supplier A and Supplier B:\n"; foreach ($differentPricesProducts as $product) { echo $product->getName() . " - Supplier A: $" . $product->getPrice() . ", Supplier B: $" . $supplierB->getProducts()[array_search($product, $supplierA->getProducts())]->getPrice() . "\n"; }
Output:
Products with different prices between Supplier A and Supplier B: Keyboard - Supplier A: $0, Supplier B: $30 Mouse - Supplier A: $15, Supplier B: $0
array_diff_assoc() Function
PHP তে array_diff_assoc() function এর কাজ হচ্ছে দুই বা ততোধিক array এর মধ্যে শুধু value এর উপর ভিত্তি করে Difference বের করবেনা , একই সাথে key এর পার্থক্য বা difference ও দেখবে। অর্থাৎ, প্রথম array এর যেইসব value গুলো অন্য array গুলোর যেকোনোটির মধ্যে পাওয়া যাবেনা , আর পাওয়া গেলেও যদি key গুলো একই (same) না হয় শুধু সেইসব value গুলো বের করার জন্য PHP তে array_diff_assoc() function টি ব্যবহৃত হয়।। চলুন একটা উদাহরণ দেখা যাক :
<?php $array1 = array("a" => "green", "b" => "brown", "d" => "blue", "red"); $array2 = array("a" => "green", "yellow", "red"); $result = array_diff_assoc($array1, $array2); echo "<pre>"; print_r($result); echo "</pre>"; ?>
Output:
Array ( [b] => brown [d] => blue [0] => red )
Example #2
class Student { private $name; private $grades = []; public function __construct($name, array $grades) { $this->name = $name; $this->grades = $grades; } public function getGrades() { return $this->grades; } public function getName() { return $this->name; } } class GradeAnalyzer { public static function findDifferingSubjects(Student $studentA, Student $studentB) { $gradesA = $studentA->getGrades(); $gradesB = $studentB->getGrades(); return array_diff_assoc($gradesA, $gradesB); } } // Create students $student1 = new Student("Alice", ["Math" => 90, "English" => 85, "Science" => 92]); $student2 = new Student("Bob", ["Math" => 92, "English" => 88, "Science" => 92]); // Use GradeAnalyzer to find differing subjects between students $differingSubjects = GradeAnalyzer::findDifferingSubjects($student1, $student2); // Display the results echo "Differing subjects and grades between {$student1->getName()} and {$student2->getName()}:\n"; foreach ($differingSubjects as $subject => $gradeA) { $gradeB = $student2->getGrades()[$subject]; echo "$subject - {$student1->getName()}: $gradeA, {$student2->getName()}: $gradeB\n"; }
Output
Differing subjects and grades between Alice and Bob: Math - Alice: 90, Bob: 92 English - Alice: 85, Bob: 88
array_diff_uassoc() Function
PHP তে array_diff_uassoc() function টি array_diff_assoc() ফাঙ্কশনের মতোই। অর্থাৎ, key এবং value দুইটারই ভিত্তিতেই Difference বের করা যায়। তবে array এর key গুলোকে চেক করার জন্য array_diff_uassoc() function নিজে করবেনা, বরং user এর supply করা callback function দিয়ে করবে । চলুন একটা উদাহরণ দেখা যাক :
। চলুন একটা উদাহরণ দেখা যাক :
<?php function myfunction($a,$b) { if ($a===$b) { return 0; } return ($a>$b)?1:-1; } $a1=array("a"=>"red","b"=>"green","f"=>"blue"); $a2=array("a"=>"red","b"=>"green","d"=>"blue"); $a3=array("e"=>"yellow","a"=>"red","d"=>"blue"); $result=array_diff_uassoc($a1,$a2,$a3,"myfunction"); echo "<pre>"; print_r($result); echo "</pre>"; ?>
Output:
Array ( [f] => blue )
Example #2
<?php // Initial array of users $oldUsers = array( 'user1' => array('name' => 'John', 'age' => 25, 'city' => 'New York'), 'user2' => array('name' => 'Jane', 'age' => 30, 'city' => 'Los Angeles'), 'user3' => array('name' => 'Bob', 'age' => 22, 'city' => 'Chicago') ); // Updated array of users $newUsers = array( 'user1' => array('name' => 'John', 'age' => 26, 'city' => 'New York'), 'user2' => array('name' => 'Jane', 'age' => 30, 'city' => 'Los Angeles'), 'user3' => array('name' => 'Bob', 'age' => 23, 'city' => 'Chicago'), 'user4' => array('name' => 'Alice', 'age' => 28, 'city' => 'San Francisco') ); function userComparison($a, $b) { return $a<=>$b; } // Find the users with changed information @$changedUsers = array_diff_uassoc($newUsers, $oldUsers, 'userComparison'); // Display the result print_r($changedUsers);
Output
Array ( [user4] => Array ( [name] => Alice [age] => 28 [city] => San Francisco ) )
array_udiff_assoc() Function
PHP তে array_udiff_assoc() function টি array_diff_assoc() ফাঙ্কশনের মতোই। অর্থাৎ, key এবং value দুইটার ভিত্তিতেই Difference বের করা যায়। তবে array এর মধ্যে key গুলোকে array_udiff_assoc() ফাঙ্কশন (Built-in) নিজে compare করবে। আর value গুলোকে user এর supply করা callback function দিয়ে Difference বের করবে। চলুন একটা উদাহরণ দেখা যাক :
<?php function myfunction($a,$b) { if ($a===$b) { return 0; } return ($a>$b)?1:-1; } $a1=array("a"=>"red","b"=>"green","e"=>"blue"); $a2=array("a"=>"red","b"=>"blue","e"=>"green"); $result=array_udiff_assoc($a1,$a2,"myfunction"); print_r($result); ?>
Output:
Array ( [b] => green [e] => blue )
Example #2
<?php // Initial array of products from Supplier A $supplierA = array( 'laptop' => array('name' => 'Laptop', 'price' => 1200, 'brand' => 'HP'), 'smartphone' => array('name' => 'Smartphone', 'price' => 800, 'brand' => 'Samsung'), 'tablet' => array('name' => 'Tablet', 'price' => 400, 'brand' => 'Apple'), ); // Updated array of products from Supplier B $supplierB = array( 'laptop' => array('name' => 'Laptop', 'price' => 1300, 'brand' => 'Dell'), 'smartphone' => array('name' => 'Smartphone', 'price' => 750, 'brand' => 'Samsung'), 'tablet' => array('name' => 'Tablet', 'price' => 420, 'brand' => 'Apple'), ); // Custom comparison function based on product name and price function productPriceComparison($a, $b) { // Compare product names (keys) $nameComparison = strcmp($a['name'], $b['name']); if ($nameComparison !== 0) { return $nameComparison; } // Compare prices return $a['price'] - $b['price']; } // Find the price differences between Supplier A and Supplier B $priceDifferences = array_udiff_assoc($supplierB, $supplierA, 'productPriceComparison'); // Display the result print_r($priceDifferences);
Output
Array ( [laptop] => Array ( [name] => Laptop [price] => 1300 [brand] => Dell ) [smartphone] => Array ( [name] => Smartphone [price] => 750 [brand] => Samsung ) [tablet] => Array ( [name] => Tablet [price] => 420 [brand] => Apple ) )
array_udiff_uassoc() Function
PHP তে array_udiff_uassoc() function টি array_udiff_assoc() ফাঙ্কশনের মতোই। অর্থাৎ, key এবং value দুইটারই ভিত্তিতেই Difference বের করা যায়। তবে array এর মধ্যে key এবং value উভয়কেই আলাদা আলাদা user এর supply করা callback function দিয়ে Difference বের করবে। চলুন একটা উদাহরণ দেখা যাক :
<?php function myfunction_key($a,$b) { if ($a===$b) { return 0; } return ($a>$b)?1:-1; } function myfunction_value($a,$b) { if ($a===$b) { return 0; } return ($a>$b)?1:-1; } $a1=array("a"=>"red","b"=>"green","f"=>"blue"); $a2=array("a"=>"red","b"=>"green","e"=>"blue"); $result=array_udiff_uassoc($a1,$a2,"myfunction_key","myfunction_value"); echo "<pre>"; print_r($result); echo "</pre>"; ?>
Output:
Array ( [f] => blue )
Example # 2
<?php // Student data in the first array $students1 = [ 'john' => ['name' => 'John Doe', 'math' => 85, 'science' => 90, 'english' => 88], 'jane' => ['name' => 'Jane Smith', 'math' => 92, 'science' => 88, 'english' => 95], 'bob' => ['name' => 'Bob Johnson', 'math' => 78, 'science' => 85, 'english' => 80], ]; // Student data in the second array $students2 = [ 'john' => ['name' => 'John Doe', 'math' => 85, 'science' => 90, 'english' => 88], 'jane' => ['name' => 'Jane Smith', 'math' => 92, 'science' => 89, 'english' => 95], // Updated science score ]; // Custom callback function for both value and key comparison function studentCompare($a, $b) { // Check if both parameters are arrays if (is_array($a) && is_array($b)) { // Compare keys $keyComparison = strcmp($a, $b); if ($keyComparison === 0) { // Compare values (subject scores) return array_sum($a) - array_sum($b); } return $keyComparison; } // Return a default value if the parameters are not arrays return 1; } // Find the students present in $students1 but not in $students2 based on both keys and values $missingStudents = array_udiff_uassoc($students1, $students2, 'studentCompare', 'studentCompare'); // Display the result print_r($missingStudents); ?>
Output:
Array ( [product4] => Array ( [name] => Camera [price] => 500 [brand] => Canon ) )
array_diff_key() function
দুই বা ততোধিক array এর মধ্যে শুধু key এর উপর ভিত্তি করে Difference বের করবে অর্থাৎ, প্রথম array এর যেইসব key গুলো অন্য array গুলোর যেকোনোটির মধ্যে পাওয়া যাবেনা শুধু সেইসব key এর value গুলো আলাদা ভাবে বের করার জন্য PHP তে array_diff_key() function টি ব্যবহৃত হয়। চলুন একটা উদাহরণ দেখা যাক :
<?php $array1 = array('blue' => 1, 'red' => 2, 'green' => 3, 'purple' => 4); $array2 = array('green' => 5, 'yellow' => 7, 'cyan' => 8); $array3 = array('blue' => 6, 'yellow' => 7, 'mauve' => 8); echo "<pre>"; print_r(array_diff_key($array1, $array2, $array3)); echo "</pre>"; ?>
Output:
Array ( [red] => 2 [purple] => 4 )
ব্যাখ্যা: লক্ষ্য করুন, প্রথম array এর red এবং purple key দুটি অন্য array গুলোর মধ্যে পাওয়া না যাওয়ায় Output হিসেবে শুধু red এবং purple প্রদর্শন করতেছে।
Example #2
// Initial array of products $oldProducts = array( 'product1' => array('name' => 'Laptop', 'price' => 1200, 'brand' => 'HP', 'stock' => 50), 'product2' => array('name' => 'Smartphone', 'price' => 800, 'brand' => 'Samsung', 'stock' => 100), 'product3' => array('name' => 'Tablet', 'price' => 400, 'brand' => 'Apple', 'stock' => 30) ); // Updated array of products $newProducts = array( 'product1' => array('name' => 'Laptop', 'price' => 1300, 'brand' => 'Dell', 'stock' => 60, 'color' => 'Silver'), 'product2' => array('name' => 'Smartphone', 'price' => 800, 'brand' => 'Samsung', 'stock' => 120), 'product4' => array('name' => 'Camera', 'price' => 500, 'brand' => 'Canon', 'stock' => 25, 'resolution' => 'HD') ); // Find the differences in terms of keys $differences = array_diff_key($newProducts, $oldProducts); // Display the result print_r($differences);
Output:
Array ( [product4] => Array ( [name] => Camera [price] => 500 [brand] => Canon [stock] => 25 [resolution] => HD ) )
array_diff_ukey() function
array_diff_ukey() function টি array_diff_key() ফাঙ্কশনের মতোই দুই বা ততোধিক array এর মধ্যে শুধু key এর উপর ভিত্তি করে Difference বের করবে , তবে সেটা array_diff_ukey() function নিজে করবেনা, বরং user এর supply করা callback function দিয়ে করবে । চলুন একটা উদাহরণ দেখা যাক :
<?php function myfunction($a,$b) { if ($a===$b) { return 0; } return ($a>$b)?1:-1; } $a1=array("a"=>"red","b"=>"green","d"=>"blue","f"=>"Cyan"); $a2=array("a"=>"blue","b"=>"black","e"=>"blue"); $result=array_diff_ukey($a1,$a2,"myfunction"); echo "<pre>"; print_r($result); echo "</pre>"; ?>
Output:
Array ( [d] => blue [f] => Cyan )
Example #2
<?php // Initial array of users $oldUsers = array( 'user123' => array('name' => 'John', 'age' => 25, 'email' => 'john@example.com'), 'user456' => array('name' => 'Jane', 'age' => 30, 'email' => 'jane@example.com'), 'user789' => array('name' => 'Bob', 'age' => 22, 'email' => 'bob@example.com'), ); // Updated array of users $newUsers = array( 'user123' => array('name' => 'John', 'age' => 26, 'email' => 'john@example.com'), 'user456' => array('name' => 'Jane', 'age' => 30, 'email' => 'jane.updated@example.com'), 'user101' => array('name' => 'Alice', 'age' => 28, 'email' => 'alice@example.com'), ); // Comparison function for user IDs function userIdComparison($a, $b) { return strcmp($a, $b); } // Find the users that exist in $newUsers but not in $oldUsers based on user IDs $newUsersOnly = array_diff_ukey($newUsers, $oldUsers, 'userIdComparison'); // Display the result print_r($newUsersOnly);
Output
Array ( [user101] => Array ( [name] => Alice [age] => 28 [email] => alice@example.com ) )