PHP Arrays
PHP Arrays পর্ব-৬: PHP Array Intersection Functions
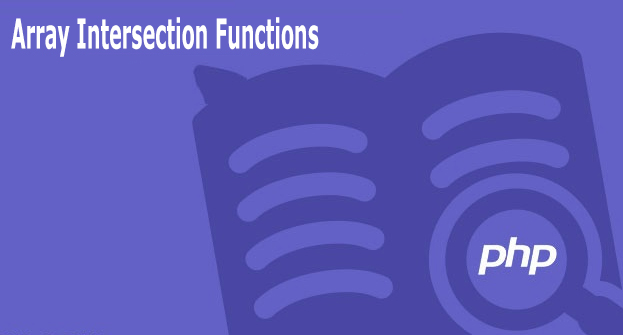
দুই বা ততোধিক array এর মধ্যে common value গুলো বের করার জন্য PHP তে ৮ টি function রয়েছে যেগুলোকে বলা হয় PHP Array Intersection Functions আজকের পর্বে আমরা PHP array Intersection function গুলো নিয়ে আলোচনা করব। চলুন দেখা যাক :
array_intersect() Function
PHP তে দুই বা ততোধিক array এর মধ্যে শুধু common value গুলো বের করার জন্য array_intersect() function টি ব্যবহৃত হয়। অর্থাৎ, প্রথম array এর যেইসব value গুলো অন্য array গুলোর সবগুলোর মধ্যে পাওয়া যাবে , শুধু সেইসব value গুলো বের করবে। চলুন একটা উদাহরণ দেখা যাক :
1 2 3 4 5 6 7 8 | <?php $array1 = array ( "a" => "green" , "red" , "blue" ); $array2 = array ( "b" => "green" , "yellow" , "red" ); $result = array_intersect ( $array1 , $array2 ); echo "<pre>" ; print_r( $result ); echo "</pre>" ; ?> |
Output:
1 2 3 4 5 | Array ( [a] => green [0] => red ) |
Example #2
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 | <?php class User { public $id ; public $name ; public $email ; public $skills ; public function __construct( $id , $name , $email , $skills ) { $this ->id = $id ; $this ->name = $name ; $this ->email = $email ; $this ->skills = $skills ; } public function display() { echo "User " . $this ->id . ": " ; echo "Name: " . $this ->name . ", " ; echo "Email: " . $this ->email . ", " ; echo "Skills: [" . implode( ", " , $this ->skills) . "]\n" ; } public static function findCommonUsers( $users , $criteria ) { $commonUsers = []; foreach ( $users as $user ) { $intersection = array_intersect ( $user ->skills, $criteria [ 'skills' ]); if (! empty ( $intersection )) { $commonUsers [] = $user ; } } return $commonUsers ; } } // Sample user data $user1 = new User(1, "John Doe" , "john@example.com" , [ "PHP" , "JavaScript" , "HTML" ]); $user2 = new User(2, "Jane Smith" , "jane@example.com" , [ "JavaScript" , "CSS" , "Python" ]); $user3 = new User(3, "Alice Johnson" , "alice@example.com" , [ "HTML" , "CSS" , "Java" ]); // Criteria for finding common users $criteria = array ( "skills" => [ "JavaScript" ]); // Finding common users based on criteria $users = [ $user1 , $user2 , $user3 ]; $commonUsers = User::findCommonUsers( $users , $criteria ); // Displaying the results echo "User 1: " ; $user1 ->display(); echo "User 2: " ; $user2 ->display(); echo "User 3: " ; $user3 ->display(); if ( empty ( $commonUsers )) { echo "No common users found.\n" ; } else { echo "Common users:\n" ; foreach ( $commonUsers as $user ) { $user ->display(); } } ?> |
Output:
User 1: User 1: Name: John Doe, Email: john@example.com, Skills: [PHP, JavaScript, HTML] User 2: User 2: Name: Jane Smith, Email: jane@example.com, Skills: [JavaScript, CSS, Python] User 3: User 3: Name: Alice Johnson, Email: alice@example.com, Skills: [HTML, CSS, Java] Common users: User 1: Name: John Doe, Email: john@example.com, Skills: [PHP, JavaScript, HTML] User 2: Name: Jane Smith, Email: jane@example.com, Skills: [JavaScript, CSS, Python]
array_uintersect() Function
PHP তে array_uintersect() function টি array_intersect() function এর মতোই অর্থাৎ, দুই বা ততোধিক array এর মধ্যে শুধু value এর উপর ভিত্তি করে Intersection বা common value গুলো বের করার জন্য ব্যবহৃত হয় । তবে array_uintersect() function কাজটি নিজে করবেনা, বরং user এর supply করা callback function দিয়ে করবে । চলুন একটা উদাহরণ দেখা যাক :
1 2 3 4 5 6 | <?php $array1 = array ( "a" => "green" , "b" => "brown" , "c" => "blue" , "red" ); $array2 = array ( "a" => "GREEN" , "B" => "brown" , "yellow" , "red" ); print_r( array_uintersect ( $array1 , $array2 , "strcasecmp" )); ?> |
Output:
1 2 3 4 5 6 | Array ( [a] => green [b] => brown [0] => red ) |
Example #2
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 | <?php class Product { public $id ; public $name ; public $category ; public $price ; public function __construct( $id , $name , $category , $price ) { $this ->id = $id ; $this ->name = $name ; $this ->category = $category ; $this ->price = $price ; } public function display() { echo "Product " . $this ->id . ": " ; echo "Name: " . $this ->name . ", " ; echo "Category: " . $this ->category . ", " ; echo "Price: $" . $this ->price . "\n" ; } public static function compareProducts( $a , $b ) { // Compare based on category and price $categoryComparison = strcmp ( $a ->category, $b ->category); $priceComparison = $a ->price - $b ->price; return ( $categoryComparison === 0 && $priceComparison === 0) ? 0 : ( $categoryComparison < 0 ? -1 : 1); } } // Sample product data $product1 = new Product(1, "Laptop" , "Electronics" , 1200); $product2 = new Product(2, "T-shirt" , "Apparel" , 25); $product3 = new Product(3, "Headphones" , "Electronics" , 100); // Criteria for finding common products $criteria = array ( "category" , "price" ); // Finding common products based on criteria $commonProducts = array_uintersect ([ $product1 , $product2 , $product3 ], [ $product2 , $product3 ], [ 'Product' , 'compareProducts' ]); // Displaying the results echo "Product 1: " ; $product1 ->display(); echo "Product 2: " ; $product2 ->display(); echo "Product 3: " ; $product3 ->display(); if ( empty ( $commonProducts )) { echo "No common products found.\n" ; } else { echo "Common products:\n" ; foreach ( $commonProducts as $product ) { $product ->display(); } } ?> |
Output:
Product 1: Product 1: Name: Laptop, Category: Electronics, Price: $1200 Product 2: Product 2: Name: T-shirt, Category: Apparel, Price: $25 Product 3: Product 3: Name: Headphones, Category: Electronics, Price: $100 Common products: Product 2: Name: T-shirt, Category: Apparel, Price: $25 Product 3: Name: Headphones, Category: Electronics, Price: $100
Example #3
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 | <?php class User{ public $id ; public $name ; public $email ; public $skills ; public function __construct( $id , $name , $email , $skills ){ $this ->id = $id ; $this ->name = $name ; $this ->email = $email ; $this ->skills = $skills ; } public function display(){ echo "User " . $this ->id. ": " ; echo "Name: " . $this ->name . ", " ; echo "Email: " . $this ->email. ", " ; echo "Skills: [" . implode( ", " , $this ->skills). "]\n" ; } public static function findCommonUsers( $users , $criteria ){ $commonUsers = []; foreach ( $users as $user ) { $intersection = array_uintersect ( $user ->skills, $criteria [ 'skills' ], "strcasecmp" ); if (! empty ( $intersection )){ $commonUsers [] = $user ; } } return $commonUsers ; } } $user1 = new User(1, "Mamnun Bin Masud" , "mamnun@bin.com" , [ "Quran" , "Hadit" , "Arabic" ]); $user2 = new User(2, "Muhibbullah Bin Masud" , "muhibbullah@bin.com" , [ "Arabic" , "English" , "Math" ]); $user3 = new User(3, "Mahmud Bin Masud" , "mahmud@bin.com" , [ "Arabic" , "English" , "hadit" ]); $criteria =[ "skills" => [ 'Hadit' ]]; $users =[ $user1 , $user2 , $user3 ]; $commonUsers =User::findCommonUsers( $users , $criteria ); echo "User 1: " ; $user1 ->display(); echo "User 2: " ; $user2 ->display(); echo "User 3: " ; $user3 ->display(); print_r( $commonUsers ); |
array_intersect_assoc() Function
PHP তে array_intersect_assoc() function এর কাজ হচ্ছে দুই বা ততোধিক array এর মধ্যে শুধু value এর উপর ভিত্তি করে Intersection বা Common value গুলো বের করবেনা , একই সাথে key এর intersection অর্থাৎ common key ও দেখবে। অর্থাৎ, প্রথম array এর যেইসব value গুলো অন্য array গুলোর যেকোনোটির মধ্যে পাওয়া যায় , আর key গুলো একই (same) হয় শুধু সেইসব value গুলো বের করার জন্য PHP তে array_intersect_assoc() function টি ব্যবহৃত হয়।। চলুন একটা উদাহরণ দেখা যাক :
1 2 3 4 5 6 | <?php $array1 = array ( "a" => "green" , "b" => "brown" , "c" => "blue" , "red" ); $array2 = array ( "a" => "green" , "b" => "yellow" , "blue" , "red" ); $result_array = array_intersect_assoc ( $array1 , $array2 ); print_r( $result_array ); ?> |
Output:
1 2 3 4 | Array ( [a] => green ) |
Example #2
001 002 003 004 005 006 007 008 009 010 011 012 013 014 015 016 017 018 019 020 021 022 023 024 025 026 027 028 029 030 031 032 033 034 035 036 037 038 039 040 041 042 043 044 045 046 047 048 049 050 051 052 053 054 055 056 057 058 059 060 061 062 063 064 065 066 067 068 069 070 071 072 073 074 075 076 077 078 079 080 081 082 083 084 085 086 087 088 089 090 091 092 093 094 095 096 097 098 099 100 101 102 103 104 105 106 107 108 109 110 111 | <?php class Book { private $id ; private $title ; private $author ; private $genre ; private $price ; public function __construct( $id , $title , $author , $genre , $price ) { $this ->id = $id ; $this ->title = $title ; $this ->author = $author ; $this ->genre = $genre ; $this ->price = $price ; } public function display() { echo "Book " . $this ->id . ": " ; echo "Title: " . $this ->title . ", " ; echo "Author: " . $this ->author . ", " ; echo "Genre: " . $this ->genre . ", " ; echo "Price: $" . $this ->price . "\n" ; } public function toArray() { return array ( "id" => $this ->id, "title" => $this ->title, "author" => $this ->author, "genre" => $this ->genre, "price" => $this ->price ); } public static function findCommonBooks( array ... $booksArray ) { // Extract array values (Book objects) for each array element $bookArrays = array_map ( function ( $books ) { return array_map ( function ( $book ) { return $book ->toArray(); }, $books ); }, $booksArray ); // Find common books based on at least one common attribute $commonBookData = call_user_func_array( 'array_intersect_assoc' , $bookArrays ); if ( empty ( $commonBookData )) { return null; } else { // Extract the first common book $commonBookData = reset( $commonBookData ); // Create a new Book object with common attributes return new self( $commonBookData [ 'id' ] ?? null, $commonBookData [ 'title' ] ?? null, $commonBookData [ 'author' ] ?? null, $commonBookData [ 'genre' ] ?? null, $commonBookData [ 'price' ] ?? null ); } } public static function calculateAveragePrice( array $books ) { $totalPrice = 0; $bookCount = count ( $books ); foreach ( $books as $book ) { $totalPrice += $book ->price; } return ( $bookCount > 0) ? ( $totalPrice / $bookCount ) : 0; } } // Sample books $book1 = new Book(1, "The Great Gatsby" , "F. Scott Fitzgerald" , "Fiction" , 20); $book2 = new Book(2, "To Kill a Mockingbird" , "Harper Lee" , "Fiction" , 15); $book3 = new Book(3, "1984" , "George Orwell" , "Dystopian" , 25); // Finding common books based on at least one common attribute (using array_intersect_assoc) $commonBook = Book::findCommonBooks([ $book1 , $book2 , $book3 ]); // Displaying the results echo "Book 1: " ; $book1 ->display(); echo "Book 2: " ; $book2 ->display(); echo "Book 3: " ; $book3 ->display(); if ( $commonBook === null) { echo "No common books found.\n" ; } else { echo "Common book:\n" ; $commonBook ->display(); } // Calculate average price of the books $averagePrice = Book::calculateAveragePrice([ $book1 , $book2 , $book3 ]); echo "Average price of the books: $" . $averagePrice . "\n" ; ?> |
Output:
Book 1: Book 1: Title: The Great Gatsby, Author: F. Scott Fitzgerald, Genre: Fiction, Price: $20 Book 2: Book 2: Title: To Kill a Mockingbird, Author: Harper Lee, Genre: Fiction, Price: $15 Book 3: Book 3: Title: 1984, Author: George Orwell, Genre: Dystopian, Price: $25 Common book: Book 1: Title: The Great Gatsby, Author: F. Scott Fitzgerald, Genre: Fiction, Price: $20 Average price of the books: $20
array_intersect_uassoc() Function
PHP তে array_intersect_uassoc() function টি array_intersect_assoc() ফাঙ্কশনের মতোই। অর্থাৎ, key এবং value দুইটাই মিল আছে কিনা তা দেখে। তবে array এর key গুলোকে চেক করার জন্য array_intersect_uassoc() function নিজে করবেনা, বরং user এর supply করা callback function দিয়ে করবে । চলুন একটা উদাহরণ দেখা যাক :
1 2 3 4 5 | <?php $array1 = array ( "a" => "green" , "b" => "brown" , "c" => "blue" , "red" ); $array2 = array ( "a" => "GREEN" , "B" => "brown" , "yellow" , "red" ); print_r( array_intersect_uassoc ( $array1 , $array2 , "strcasecmp" )); ?> |
Output:
1 2 3 4 | Array ( [b] => brown ) |
Example #2
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 | <?php function custom_key_compare( $key1 , $key2 ) { // Case-insensitive comparison of keys return strcasecmp ( $key1 , $key2 ); } // Arrays to intersect $array1 = array ( "a" => 1, "b" => 2, "c" => 3); $array2 = array ( "B" => 2, "D" => 3, "A" => 4); // Using array_intersect_uassoc with the custom key comparison function $result = array_intersect_uassoc ( $array1 , $array2 , 'custom_key_compare' ); // Display the result print_r( $result ); ?> |
Example #3
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 | <?php class Employee { public $id ; public $name ; public $skills ; public function __construct( $id , $name , $skills ) { $this ->id = $id ; $this ->name = $name ; $this ->skills = $skills ; } public function display() { echo "Employee " . $this ->id . ": " ; echo "Name: " . $this ->name . ", " ; echo "Skills: [" . implode( ", " , $this ->skills) . "]\n" ; } } // Available employees $employees = [ 1 => new Employee(1, "Alice" , [ "PHP" , "JavaScript" , "HTML" ]), 2 => new Employee(2, "Bob" , [ "JavaScript" , "Python" , "CSS" ]), 3 => new Employee(3, "Charlie" , [ "PHP" , "Java" , "SQL" ]), ]; // Desired set of skills $desired_skills = [ "PHP" , "JavaScript" ]; // Custom callback function for key comparison function custom_key_compare( $key1 , $key2 ) { return strcasecmp ( $key1 , $key2 ); } // Use array_intersect_uassoc with the custom key comparison function $matching_employees = array_intersect_uassoc ( $desired_skills , $employees [1]->skills, 'custom_key_compare' ); // Filter employees based on the matching skills $filtered_employees = array_filter ( $employees , function ( $employee ) use ( $matching_employees ) { return ! empty ( array_intersect_uassoc ( $matching_employees , $employee ->skills, 'custom_key_compare' )); }); // Display the matching employees echo "Desired Skills: [" . implode( ", " , $desired_skills ) . "]\n" ; echo "Matching Employees:\n" ; foreach ( $filtered_employees as $employee ) { $employee ->display(); } ?> |
Output
Desired Skills: [PHP, JavaScript] Matching Employees: Employee 1: Name: Alice, Skills: [PHP, JavaScript, HTML] Employee 3: Name: Charlie, Skills: [PHP, Java, SQL]
array_uintersect_assoc() Function
PHP তে array_uintersect_assoc() function টি array_intersect_assoc() ফাঙ্কশনের মতোই। অর্থাৎ, key এবং value দুইটাই মিল আছে কিনা তা দেখে। তবে array এর মধ্যে key গুলোকে array_uintersect_assoc() ফাঙ্কশন (Built-in) নিজে check করবে। আর value গুলোকে user এর supply করা callback function দিয়ে Intersection বা সমতা চেক করবে। চলুন একটা উদাহরণ দেখা যাক :
1 2 3 4 5 6 | <?php $array1 = array ( "a" => "green" , "b" => "brown" , "c" => "blue" , "red" ); $array2 = array ( "a" => "GREEN" , "B" => "brown" , "yellow" , "red" ); print_r( array_uintersect_assoc ( $array1 , $array2 , "strcasecmp" )); ?> |
Output:
1 2 3 4 | Array ( [a] => green ) |
Example #2
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 | <?php // Define multiple datasets $dataset1 = [ "id" => 1, "name" => "John" , "age" => 25, "city" => "New York" , ]; $dataset2 = [ "id" => 2, "name" => "Jane" , "age" => 30, "city" => "Los Angeles" , ]; $dataset3 = [ "id" => 3, "name" => "Bob" , "age" => 25, "city" => "Chicago" , ]; // Define user preferences for filtering based on age and city $user_preferences = [ "age" => 25, "city" => "New York" , ]; // Custom comparison function for array_uintersect_assoc function compare_datasets( $a , $b ) { // Compare based on age and city $ageComparison = $a [ 'age' ] - $b [ 'age' ]; $cityComparison = $a [ 'city' ]=== $b [ 'city' ]; // Combine individual comparisons using logical AND return $ageComparison === 0 && $cityComparison === 0; } // Find the intersection of datasets based on user preferences $user_filtered_data = array_uintersect_assoc ([ $dataset1 , $dataset2 , $dataset3 ], [ $user_preferences ], 'compare_datasets' ); // Display the result print_r( $user_filtered_data ); |
Output
Array ( [0] => Array ( [id] => 1 [name] => John [age] => 25 [city] => New York ) )
array_uintersect_uassoc() Function
PHP তে array_uintersect_uassoc() function টি array_uintersect_assoc() ফাঙ্কশনের মতোই। অর্থাৎ, key এবং value দুইটারই ভিত্তিতেই Intersection বের করা যায়। তবে array এর মধ্যে key এবং value উভয়কেই আলাদা আলাদা user এর supply করা callback function দিয়ে Intersection বের করবে। চলুন একটা উদাহরণ দেখা যাক :
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 | <?php function myfunction_key( $a , $b ) { if ( $a === $b ) { return 0; } return ( $a > $b )?1:-1; } function myfunction_value( $a , $b ) { if ( $a === $b ) { return 0; } return ( $a > $b )?1:-1; } $a1 = array ( "a" => "red" , "b" => "green" , "c" => "blue" ); $a2 = array ( "a" => "red" , "b" => "green" , "c" => "green" ); $result = array_uintersect_uassoc ( $a1 , $a2 , "myfunction_key" , "myfunction_value" ); print_r( $result ); ?> |
Output
1 2 3 4 | Array ( [a] => red [b] => green ) |
Example #2
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 | <?php // Simulated employee data $employees = [ 1 => [ "id" => 1, "name" => "Alice" , "age" => 25, "department" => "HR" ], 2 => [ "id" => 2, "name" => "Bob" , "age" => 30, "department" => "IT" ], 3 => [ "id" => 3, "name" => "Charlie" , "age" => 22, "department" => "Marketing" ], // ... additional employee data ]; // Criteria for finding employees $criteria = [ "age" => 25, "department" => "HR" , ]; // Comparison function for array_uintersect_assoc function employee_compare( $employee1 , $employee2 ) { // Custom comparison based on age $ageComparison = $employee1 [ "age" ] - $employee2 [ "age" ]; // Custom comparison based on department $departmentComparison = strcasecmp ( $employee1 [ "department" ], $employee2 [ "department" ]); return $ageComparison === 0 && $departmentComparison === 0 ? 0 : 1; } $matchingEmployees = []; // Loop through each employee to find matches foreach ( $employees as $employee ) { // Use array_uintersect_assoc to find matching employees $result = array_uintersect_assoc ([ $employee ], [ $criteria ], 'employee_compare' ); if (! empty ( $result )){ $matchingEmployees = $result ; } } // Display the matching employees echo "Employees Matching Criteria:\n" ; print_r( $matchingEmployees ); ?> |
Output
Employees Matching Criteria: Array ( [0] => Array ( [id] => 1 [name] => Alice [age] => 25 [department] => HR ) )
array_intersect_key() function
দুই বা ততোধিক array এর মধ্যে শুধু key এর উপর ভিত্তি করে Intersection করবে অর্থাৎ, প্রথম array এর যেইসব key গুলো অন্য array গুলোর যেকোনোটির মধ্যে পাওয়া যাবে শুধু সেইসব key এর value গুলো আলাদা ভাবে বের করার জন্য PHP তে array_intersect_key() function টি ব্যবহৃত হয়। চলুন একটা উদাহরণ দেখা যাক :
1 2 3 4 5 6 7 | <?php $array1 = array ( 'blue' => 1, 'red' => 2, 'green' => 3, 'purple' => 4); $array2 = array ( 'green' => 5, 'blue' => 6, 'yellow' => 7, 'cyan' => 8); echo "<pre>" ; print_r( array_intersect_key ( $array1 , $array2 )); echo "</pre>" ; ?> |
Output:
1 2 3 4 5 | Array ( [blue] => 1 [green] => 3 ) |
array_intersect_ukey() function
array_intersect_ukey() function টি array_intersect_key() ফাঙ্কশনের মতোই দুই বা ততোধিক array এর মধ্যে শুধু key এর উপর ভিত্তি করে Intersection করবে , তবে সেটা array_intersect_ukey() function নিজে করবেনা, বরং user এর supply করা callback function দিয়ে করবে । চলুন একটা উদাহরণ দেখা যাক :
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 | <?php function myfunction( $a , $b ) { if ( $a === $b ) { return 0; } return ( $a > $b )?1:-1; } $a1 = array ( "a" => "red" , "b" => "green" , "c" => "blue" ); $a2 = array ( "a" => "blue" , "b" => "black" , "e" => "blue" ); $result = array_intersect_ukey ( $a1 , $a2 , "myfunction" ); echo "<pre>" ; print_r( $result ); echo "</pre>" ?> |
Output:
1 2 3 4 5 | Array ( [a] => red [b] => green ) |